Table of Contents
Introduction
Welcome to Create Simple GUI Applications the practical guide to building professional desktop applications with Python & Qt.
If you want to learn how to write GUI applications it can be pretty tricky to get started. There are a lot of new concepts you need to understand to get anything to work. A lot of tutorials offer nothing but short code snippets without any explanation of the underlying systems and how they work together. But, like any code, writing GUI applications requires you to learn to think about the problem in the right way.
In this book I will give you the real useful basics that you need to get building functional applications with the PyQt framework. I’ll include explanations, diagrams, walkthroughs and code to make sure you know what you’re doing every step of the way. In no time at all you will have a fully functional Qt application - ready to customise as you like.
The source code for each step is included, but don’t just copy and paste and move on. You will learn much more if you experiment along the way!
So, let’s get started!
Book format
This book is formatted as a series of coding exercises and snippets to allow you to gradually explore and learn the details of PyQt5. However, it is not possible to give you a complete overview of the Qt system in a book of this size (it’s huge, this isn’t), so you are encouraged to experiment and explore along the way.
If you find yourself thinking “I wonder if I can do that” the best thing you can do is put this book down, then go and find out! Just keep regular backups of your code along the way so you always have something to come back to if you royally mess it up.
Qt and PyQt
When you write applications using PyQt what you area really doing is writing applications in Qt. The PyQt library is simply1 a wrapper around the C++ Qt library, to allow it to be used in Python.
Because this is a Python interface to a C++ library the naming conventions used within PyQt do not adhere to PEP8 standards. Most notably functions and variables are named using mixedCase
rather than snake_case
. Whether you adhere to this standard in your own applications based on PyQt is entirely up to you, however you may find it useful to help clarify where the PyQt code ends and your own begins.
Further, while there is PyQt specific documentation available, you will often find yourself reading the Qt documentation itself as it is more complete. If you do you will need to translate object syntax and some methods containing Python-reserved function names as follows:
Qt | PyQt |
---|---|
Qt::SomeValue |
Qt.SomeValue |
object.exec() |
object.exec_() |
object.print() |
object.print_() |
Python 3
This book is written to be compatible with Python 3.4+. Python 3 is the future of the language, and if you’re starting out now is where you should be focusing your efforts. However, in recognition of the fact that many people are stuck supporting or developing on legacy systems, the examples and code used in this book are also tested and confirmed to work on Python 2.7. Any notable incompatibility or gotchas will be flagged with a meh-face to accurately portray the sentiment e.g.
If you are using Python 3 you can safely ignore their indifferent gaze.
Getting Started
Before you start coding you will first need to have a working installation of PyQt and Qt on your system. The following sections will guide you through this process for the main available platforms. If you already have a working installation of PyQt on your Python system you can safely skip this part and get straight onto the fun.
The complete source code all examples in this book is available to download from here.
Installation Windows
PyQt5 for Windows can be installed as for any other application or library. The only slight complication is that you must first determine whether your system supports 32bit or 64bit software. You can determine whether your system supports 32bit or 64bit by looking at the System panel accessible from the control panel.
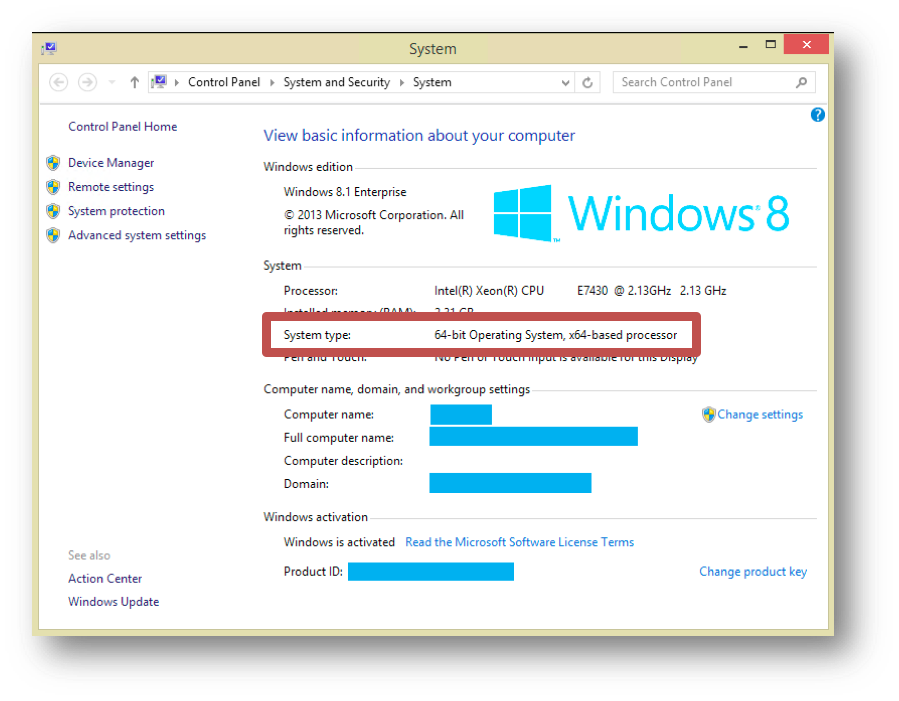
If your system does support 64bit (and most modern systems do) then you
should also check whether your current Python install is 32 or 64 bit. Open a command prompt (Start > cmd
):
1
C
:
\>
python3
Look at the top line of the Python output, where you should be able to see whether you have 32bit or 64bit Python installed. If you want to switch to 32bit or 64bit Python you should do so at this point.
PyQt5 for Python 3
A PyQt5 installer for Windows is available direct from the developer Riverbank Computing. Download the .exe
files from the linked page, making sure you download the currently 64bit or 32bit version for your system. You can install this file as for any other Windows application/library.
After install is finished, you should be able to run python
and import PyQt5
.
PyQt5 for Python 2.7
Unfortunately, if you want to use PyQt5 on Python 2.7 there are no official installers available to do this. This part of a policy by Riverbank Computing to encourage transition to Python 3 and reduce their support burden.
However, there is nothing technically stopping PyQt5 being compiled for Python 2.7 and the helpful people at Abstract Factory have done exactly that.
Simply download the above .rar
file and unpack it with 7zip (or other unzip application). You can then copy the resulting folder to your Python site-packages folder — usually in C:\Python27\lib\site-packages\
Once that is done, you should be able to run python
and import PyQt5
.
Installation Mac
OS X comes with a pre-installed version of Python (2.7), however attempting to install PyQt5 into this is more trouble than it is worth. If you are planning to do a lot of Python development, and you should, it will be easier in the long run to create a distinct installation of Python separate from the system.
By far the simplest way to do this is to use Homebrew. Homebrew is a package manager for command-line software on MacOS X. Helpfully Homebrew also has a pre-built version of PyQt5 in their repositories.
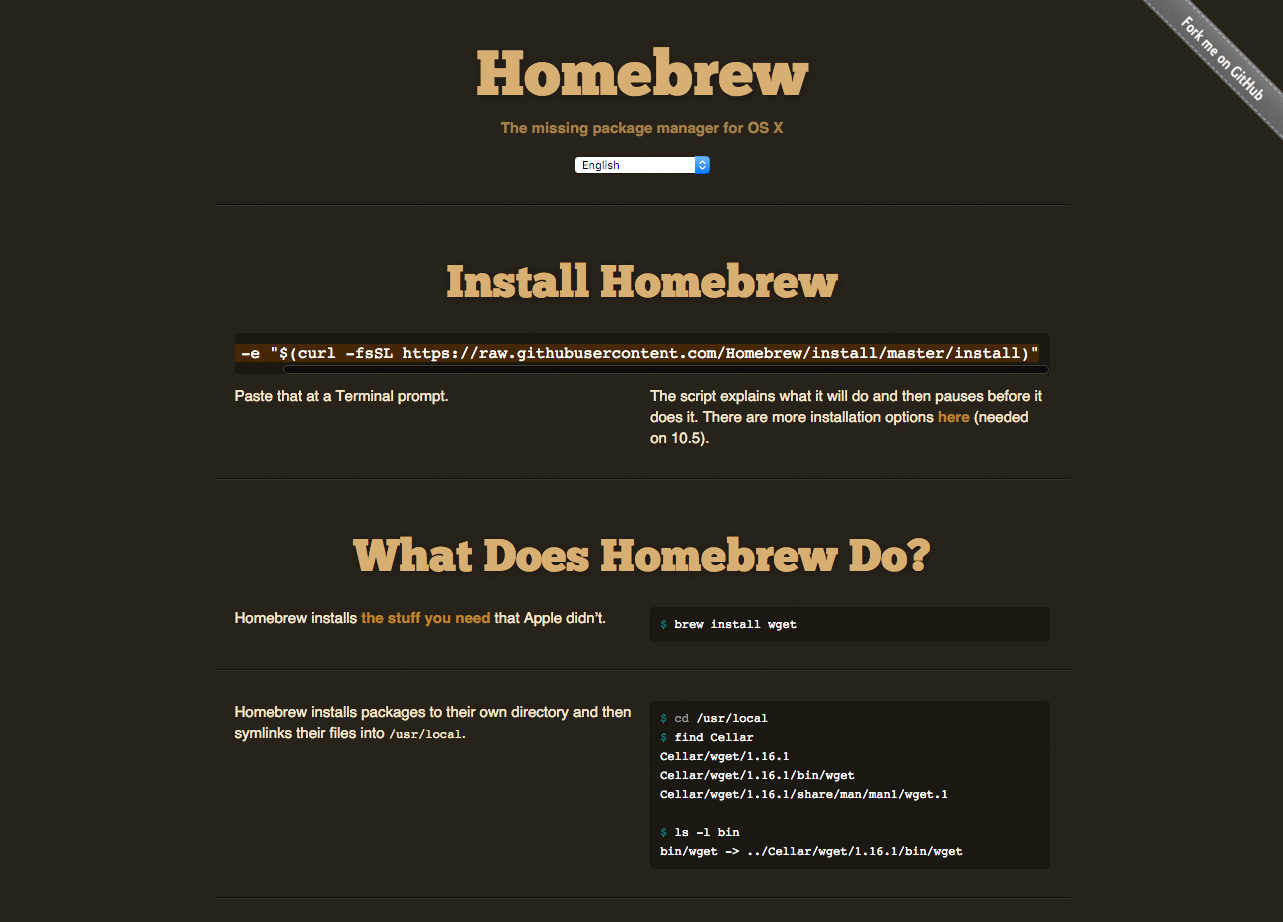
To install Homebrew run the following from the command line:
1
ruby -e "
$(
curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master\
2
/install)
"
Once the Homebrew installation has completed, you can then install Python 3 and PyQt5 as follows:
1
brew install python3
2
brew install pyqt5 --with-python-3
After that has completed, you should be able to run python3
and import PyQt5
.
Installation Linux (Ubuntu)
Installation on Linux is very straightforward as packages for PyQt5 are available in the repositories of most distributions. In Ubuntu you can install either from the command line or via “Software Center”. The packages you are looking for are named python3-pyqt5
or python-pyqt5
depending on which version you are installing for.
You can also install these from the command line as follows:
1
apt-get install python3-pyqt5
Or for Python 2.7:
1
apt-get install python-pyqt5
Once the installation is finished, you should be able to run python3
or python
and import PyQt5
.
Creating Custom Widgets
In the previous chapter we introduced QPainter
and looked at some basic bitmap drawing operations which you can used to draw dots, lines, rectangles and circles on a QPainter
surface such as a QPixmap
.
This process of drawing on a surface with QPainter
is in fact the basis by which all widgets in Qt are drawn.Now you know how to use QPainter
you know how to draw your own custom widgets!
In this chapter we’ll take what we’ve learnt so far and use it to construct a completely new custom widget. For a working example we’ll be building the following widget — a customisable PowerBar meter with a dial control.
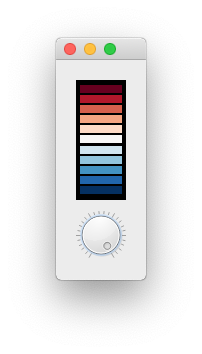
This widget is actually a mix of a compound widget and custom widget in that we are using the built-in Qt QDial
component for the dial, while drawing the power bar ourselves. We then assemble these two parts together into a parent widget which can be dropped into place seamlessly in any application, without needing to know how it’s put together. The resulting widget provides the common QAbstractSlider
interface with some additions for configuring the bar display.
After following this example you will be able to build your very own custom widgets — whether they are compounds of built-ins or completely novel self-drawn wonders.
Getting started
As we’ve previously seen compound widgets are simply widgets with a layout applied, which itself contains >1 other widget. The resulting “widget” can then be used as any other, with the internals hidden/exposed as you like.
The outline for our PowerBar widget is given below — we’ll build our custom widget up gradually from this outline stub.
1
from
PyQt5
import
QtCore
,
QtGui
,
QtWidgets
2
from
PyQt5.QtCore
import
Qt
3
4
5
class
_Bar
(
QtWidgets
.
QWidget
):
6
pass
7
8
class
PowerBar
(
QtWidgets
.
QWidget
):
9
"""
10
Custom Qt Widget to show a power bar and dial.
11
Demonstrating compound and custom-drawn widget.
12
"""
13
14
def
__init__
(
self
,
steps
=
5
,
*
args
,
**
kwargs
):
15
super
(
PowerBar
,
self
)
.
__init__
(
*
args
,
**
kwargs
)
16
17
layout
=
QtWidgets
.
QVBoxLayout
()
18
self
.
_bar
=
_Bar
()
19
layout
.
addWidget
(
self
.
_bar
)
20
21
self
.
_dial
=
QtWidgets
.
QDial
()
22
layout
.
addWidget
(
self
.
_dial
)
23
24
self
.
setLayout
(
layout
)
This simply defines our custom power bar is defined in the _Bar
object — here just unaltered subclass of QWidget
. The PowerBar
widget (which is the complete widget) combines this, using a QVBoxLayout
with the built in QDial
to display them together.
Save this to a file named power_bar.py
We also need a little demo application to display the widget.
1
from
PyQt5
import
QtCore
,
QtGui
,
QtWidgets
2
from
power_bar
import
PowerBar
3
4
5
app
=
QtWidgets
.
QApplication
([])
6
volume
=
PowerBar
()
7
volume
.
show
()
8
app
.
exec_
()
N> We don’t need to create a QMainWindow
since any widget without a parent is a window in it’s own right. Our custom PowerBar
widget will appear as any normal window.
This is all you need, just save it in the same folder as the previous file, under something like demo.py
. You can run this file at any time to see your widget in action. Run it now and you should see something like this:
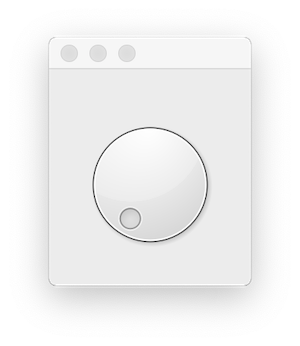
If you stretch the window down you’ll see the dial has more space above it than below — this is being taken up by our (currently invisible) _Bar
widget.
paintEvent
The paintEvent
handler is the core of all widget drawing in PyQt.
Every complete and partial re-draw of a widget is triggered through a paintEvent
which the widget handles to draw itself. A paintEvent
can be triggered by —
- repaint() or update() was called
- the widget was obscured and has now been uncovered
- the widget has been resized
— but it can also occur for many other reasons. What is important is that when a paintEvent
is triggered your widget is able to redraw it.
If a widget is simple enough (like ours is) you can often get away with simply redrawing the entire thing any time anything happens. But for more complicated widgets this can get very inefficient. For these cases the paintEvent
includes the specific region that needs to be updated. We’ll make use of this in later, more complicated examples.
For now we’ll do something very simple, and just fill the entire widget with a single colour. This will allow us to see the area we’re working with to start drawing the bar.
1
def
paintEvent
(
self
,
e
):
2
painter
=
QtGui
.
QPainter
(
self
)
3
brush
=
QtGui
.
QBrush
()
4
brush
.
setColor
(
QtGui
.
QColor
(
'black'
))
5
brush
.
setStyle
(
Qt
.
SolidPattern
)
6
rect
=
QtCore
.
QRect
(
0
,
0
,
painter
.
device
()
.
width
(),
painter
.
device
()
.
he
\
7
ight
())
8
painter
.
fillRect
(
rect
,
brush
)
Positioning
Now we can see the _Bar
widget we can tweak its positioning and size. If you drag around the shape of the window you’ll see the two widgets changing shape to fit the space available. This is what we want, but the QDial
is also expanding vertically more than it should, and leaving empty space we could use for the bar.
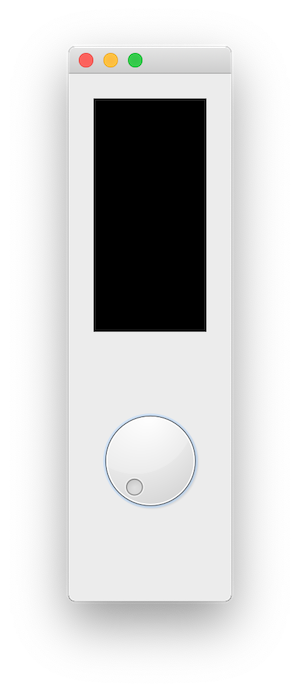
We can use setSizePolicy
on our _Bar
widget to make sure it expands as far as possible. By using the QSizePolicy.MinimumExpanding
the provided sizeHint
will be used as a minimum, and the widget will expand as much as possible.
1
class
_Bar
(
QtWidgets
.
QWidget
):
2
3
def
__init__
(
self
,
*
args
,
**
kwargs
):
4
super
()
.
__init__
(
*
args
,
**
kwargs
)
5
6
self
.
setSizePolicy
(
7
QtWidgets
.
QSizePolicy
.
MinimumExpanding
,
8
QtWidgets
.
QSizePolicy
.
MinimumExpanding
9
)
10
11
def
sizeHint
(
self
):
12
return
QtCore
.
QSize
(
40
,
120
)
It’s still not perfect as the QDial
widget resizes itself a bit awkwardly, but our bar is now expanding to fill all the available space.
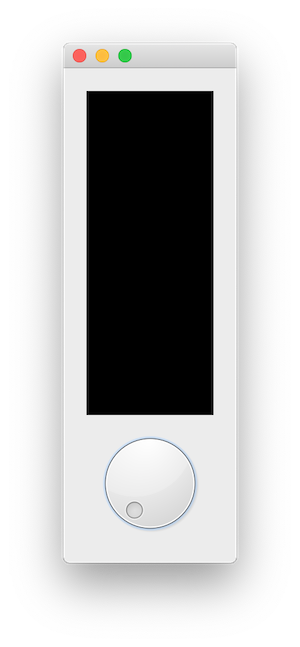
With the positioning sorted we can now move on to define our paint methods to draw our PowerBar meter in the top part (currently black) of the widget.
Updating the display
We now have our canvas completely filled in black, next we’ll use QPainter
draw commands to actually draw something on the widget.
Before we start on the bar, we’ve got a bit of testing to do to make sure we can update the display with the values of our dial. Update the paintEvent
with the following code.
1
def
paintEvent
(
self
,
e
):
2
painter
=
QtGui
.
QPainter
(
self
)
3
4
brush
=
QtGui
.
QBrush
()
5
brush
.
setColor
(
QtGui
.
QColor
(
'black'
))
6
brush
.
setStyle
(
Qt
.
SolidPattern
)
7
rect
=
QtCore
.
QRect
(
0
,
0
,
painter
.
device
()
.
width
(),
painter
.
device
()
.
he
\
8
ight
())
9
painter
.
fillRect
(
rect
,
brush
)
10
11
# Get current state.
12
dial
=
self
.
parent
()
.
_dial
13
vmin
,
vmax
=
dial
.
minimum
(),
dial
.
maximum
()
14
value
=
dial
.
value
()
15
16
pen
=
painter
.
pen
()
17
pen
.
setColor
(
QtGui
.
QColor
(
'red'
))
18
painter
.
setPen
(
pen
)
19
20
font
=
painter
.
font
()
21
font
.
setFamily
(
'Times'
)
22
font
.
setPointSize
(
18
)
23
painter
.
setFont
(
font
)
24
25
painter
.
drawText
(
25
,
25
,
"{}-->{}<--{}"
.
format
(
vmin
,
value
,
vmax
))
26
painter
.
end
()
This draws the black background as before, then uses .parent()
to access our parent PowerBar
widget and through that the QDial
via _dial
. From there we get the current value, as well as the allowed range minimum and maximum values. Finally we draw those using the painter, just like we did in the previous part.
Run this, wiggle the dial around and …..nothing happens. Although we’ve defined the paintEvent
handler we’re not triggering a repaint when the dial changes.
To fix this we need to hook up our _Bar
widget to repaint itself in response to changing values on the dial. We can do this using the QDial.valueChanged
signal, hooking it up to a custom slot method which calls .refresh()
— triggering a full-repaint.
Add the following method to the _Bar
widget.
1
def
_trigger_refresh
(
self
):
2
self
.
update
()
…and add the following to the __init__
block for the parent PowerBar
widget.
1
self
.
_dial
.
valueChanged
.
connect
(
self
.
_bar
.
_trigger_refresh
)
If you re-run the code now, you will see the display updating automatically as you turn the dial (click and drag with your mouse). The current value is displayed as text.
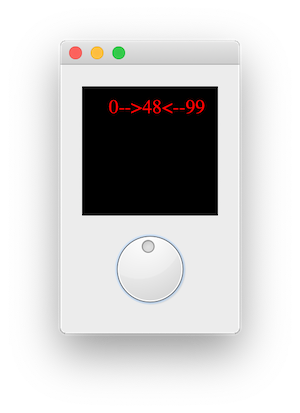
Drawing the bar
Now we have the display updating and displaying the current value of the dial, we can move onto drawing the actual bar display. This is a little complicated, with a bit of maths to calculate bar positions, but we’ll step through it to make it clear what’s going on.
The sketch below shows what we are aiming for — a series of N boxes, inset from the edges of the widget, with spaces between them.
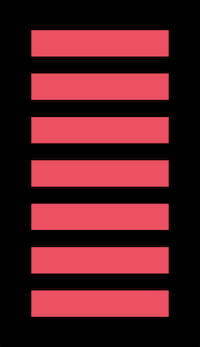
Calculating what to draw
The number of boxes to draw is determined by the current value — and how far along it is between the minimum and maximum value configured for the QDial
. We already have that information in the example above.
1
dial
=
self
.
parent
()
.
_dial
2
vmin
,
vmax
=
dial
.
minimum
(),
dial
.
maximum
()
3
value
=
dial
.
value
()
If value
is half way between vmin
and vmax
then we want to draw half of the boxes (if we have 4 boxes total, draw 2). If value
is at vmax
we want to draw them all.
To do this we first convert our value
into a number between 0 and 1, where 0 = vmin
and 1 = vmax
. We first subtract vmin
from value
to adjust the range of possible values to start from zero — i.e. from vmin...vmax
to 0…(vmax-vmin)
. Dividing this value by vmax-vmin
(the new maximum) then gives us a number between 0 and 1.
The trick then is to multiply this value (called pc
below) by the number of steps and that gives us a number between 0 and 5 — the number of boxes to draw.
1
pc
=
(
value
-
vmin
)
/
(
vmax
-
vmin
)
2
n_steps_to_draw
=
int
(
pc
*
5
)
We’re wrapping the result in int
to convert it to a whole number (rounding down) to remove any partial boxes.
Update the drawText
method in your paint event to write out this number instead.
1
pc
=
(
value
-
vmin
)
/
(
vmax
-
vmin
)
2
n_steps_to_draw
=
int
(
pc
*
5
)
3
painter
.
drawText
(
25
,
25
,
"{}"
.
format
(
n_steps_to_draw
))
As you turn the dial you will now see a number between 0 and 5.
Drawing boxes
Next we want to convert this number 0…5 to a number of bars drawn on the canvas. Start by removing the drawText
and font and pen settings, as we no longer need those.
To draw accurately we need to know the size of our canvas — i.e the size of the widget. We will also add a bit of padding around the edges to give space around the edges of the blocks against the black background.
1
padding
=
5
2
3
# Define our canvas.
4
d_height
=
painter
.
device
()
.
height
()
-
(
padding
*
2
)
5
d_width
=
painter
.
device
()
.
width
()
-
(
padding
*
2
)
We take the height and width and subtract 2 * padding
from each — it’s 2x because we’re padding both the left and right (and top and bottom) edges. This gives us our resulting active canvas area in d_height
and d_width
.
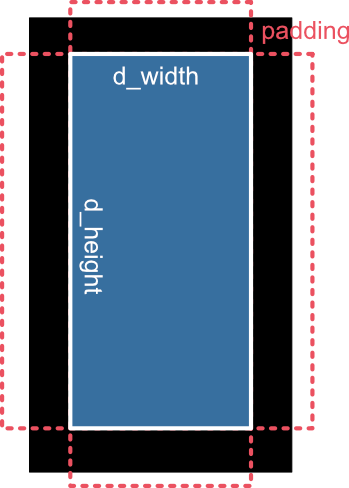
We need to break up our d_height
into 5 equal parts, one for each block — we can calculate that height simply by d_height / 5
. Additionally, since we want spaces between the blocks we need to calculate how much of this step size is taken up by space (top and bottom, so halved) and how much is actual block.
1
step_size
=
d_height
/
5
2
bar_height
=
step_size
*
0.6
3
bar_spacer
=
step_size
*
0.4
/
2
These values are all we need to draw our blocks on our canvas. To do this we count up to the number of steps-1 starting from 0 using range
and then draw a fillRect
over a region for each block.
1
brush
.
setColor
(
QtGui
.
QColor
(
'red'
))
2
3
for
n
in
range
(
5
):
4
rect
=
QtCore
.
QRect
(
5
padding
,
6
padding
+
d_height
-
((
n
+
1
)
*
step_size
)
+
bar_spacer
,
7
d_width
,
8
bar_height
9
)
10
painter
.
fillRect
(
rect
,
brush
)
N> The fill is set to a red brush to begin with but we will customise this later.
The box to draw with fillRect
is defined as a QRect
object to which we pass, in turn, the left x, top y, width and height.
The width is the full canvas width minus the padding, which we previously calculated and stored in d_width
. The left x is similarly just the padding
value (5px) from the left hand side of the widget.
The height of the bar bar_height
we calculated as 0.6 times the step_size
.
This leaves parameter 2 d_height - ((1 + n) * step_size) + bar_spacer
which gives the top y position of the rectangle to draw. This is the only calculation that changes as we draw the blocks.
A key fact to remember here is that y coordinates in QPainter
start at the top and increase down the canvas. This means that plotting at d_height
will be plotting at the very bottom of the canvas. When we draw a rectangle from a point it is drawn to the right and down from the starting position.
In our bar meter we’re drawing blocks, in turn, starting at the bottom and working upwards. So our very first block must be placed at d_height-step_size
and the second at d_height-(step_size*2)
. Our loop iterates from 0 upwards, so we can achieve this with the following formula —
1
d_height
-
((
1
+
n
)
*
step_size
The final adjustment is to account for our blocks only taking up part of each step_size
(currently 0.6). We add a little padding to move the block away from the edge of the box and into the middle, and finally add the padding for the bottom edge. That gives us the final formula —
1
padding
+
d_height
-
((
n
+
1
)
*
step_size
)
+
bar_spacer
,
This produces the following layout.
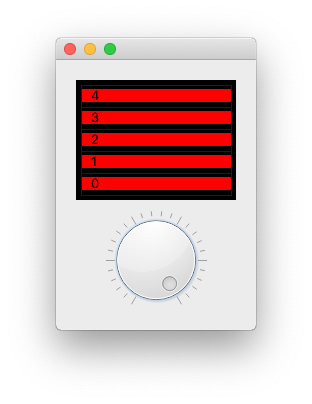
Putting this all together gives the following code, which when run will produce a working power-bar widget with blocks in red. You can drag the wheel back and forth and the bars will move up and down in response.
1
from
PyQt5
import
QtCore
,
QtGui
,
QtWidgets
2
from
PyQt5.QtCore
import
Qt
3
4
class
_Bar
(
QtWidgets
.
QWidget
):
5
6
def
__init__
(
self
,
*
args
,
**
kwargs
):
7
super
()
.
__init__
(
*
args
,
**
kwargs
)
8
9
self
.
setSizePolicy
(
10
QtWidgets
.
QSizePolicy
.
MinimumExpanding
,
11
QtWidgets
.
QSizePolicy
.
MinimumExpanding
12
)
13
14
def
sizeHint
(
self
):
15
return
QtCore
.
QSize
(
40
,
120
)
16
17
def
paintEvent
(
self
,
e
):
18
painter
=
QtGui
.
QPainter
(
self
)
19
20
brush
=
QtGui
.
QBrush
()
21
brush
.
setColor
(
QtGui
.
QColor
(
'black'
))
22
brush
.
setStyle
(
Qt
.
SolidPattern
)
23
rect
=
QtCore
.
QRect
(
0
,
0
,
painter
.
device
()
.
width
(),
painter
.
device
()
.
he
\
24
ight
())
25
painter
.
fillRect
(
rect
,
brush
)
26
27
# Get current state.
28
dial
=
self
.
parent
()
.
_dial
29
vmin
,
vmax
=
dial
.
minimum
(),
dial
.
maximum
()
30
value
=
dial
.
value
()
31
32
padding
=
5
33
34
# Define our canvas.
35
d_height
=
painter
.
device
()
.
height
()
-
(
padding
*
2
)
36
d_width
=
painter
.
device
()
.
width
()
-
(
padding
*
2
)
37
38
# Draw the bars.
39
step_size
=
d_height
/
5
40
bar_height
=
step_size
*
0.6
41
bar_spacer
=
step_size
*
0.4
/
2
42
43
pc
=
(
value
-
vmin
)
/
(
vmax
-
vmin
)
44
n_steps_to_draw
=
int
(
pc
*
5
)
45
brush
.
setColor
(
QtGui
.
QColor
(
'red'
))
46
for
n
in
range
(
n_steps_to_draw
):
47
rect
=
QtCore
.
QRect
(
48
padding
,
49
padding
+
d_height
-
((
n
+
1
)
*
step_size
)
+
bar_spacer
,
50
d_width
,
51
bar_height
52
)
53
painter
.
fillRect
(
rect
,
brush
)
54
55
painter
.
end
()
56
57
def
_trigger_refresh
(
self
):
58
self
.
update
()
59
60
61
class
PowerBar
(
QtWidgets
.
QWidget
):
62
"""
63
Custom Qt Widget to show a power bar and dial.
64
Demonstrating compound and custom-drawn widget.
65
"""
66
67
def
__init__
(
self
,
steps
=
5
,
*
args
,
**
kwargs
):
68
super
(
PowerBar
,
self
)
.
__init__
(
*
args
,
**
kwargs
)
69
70
layout
=
QtWidgets
.
QVBoxLayout
()
71
self
.
_bar
=
_Bar
()
72
layout
.
addWidget
(
self
.
_bar
)
73
74
self
.
_dial
=
QtWidgets
.
QDial
()
75
self
.
_dial
.
valueChanged
.
connect
(
76
self
.
_bar
.
_trigger_refresh
77
)
78
79
layout
.
addWidget
(
self
.
_dial
)
80
self
.
setLayout
(
layout
)
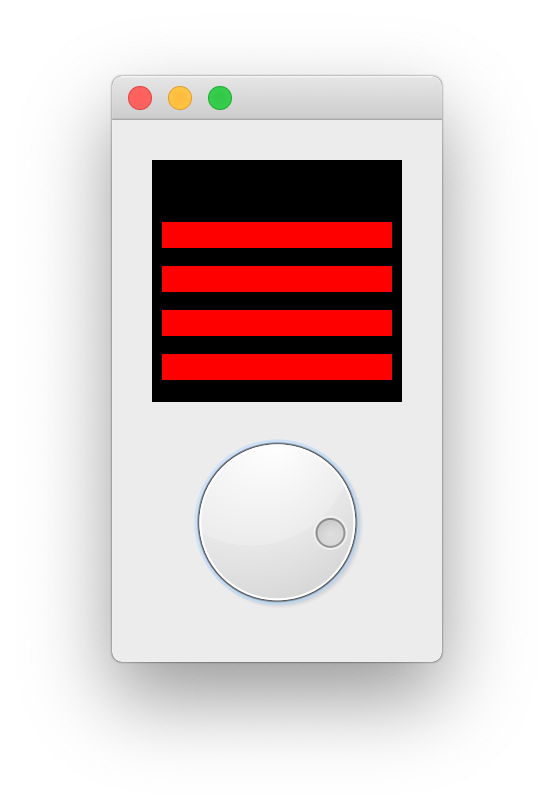
That already does the job, but we can go further to provide more customisation, add some UX improvements and improve the API for working with our widget.
Customising the Bar
We now have a working power bar, controllable with a dial. But it’s nice when creating widgets to provide options to configure the behaviour of your widget to make it more flexible. In this part we’ll add methods to set customisable numbers of segments, colours, padding and spacing.
The elements we’re going to provide customisation of are as follows —
Option | Description |
---|---|
number of bars | How many bars are displayed on the widget |
colours | Individual colours for each of the bars |
background colour | The colour of the draw canvas (default black) |
padding | Space around the widget edge, between bars and edge of canvas. |
bar height / bar percent | Proportion (0…1) of the bar which is solid (the rest will be spacing between adjacent bars) |
We can store each of these as attributes on the _bar
object, and use them from the paintEvent
method to change its behaviour.
The _Bar.__init__
is updated to accept an initial argument for either the number of bars (as an integer) or the colours of the bars (as a list of QColor
, hex values or names). If a number is provided, all bars will be coloured red. If the a list of colours is provided the number of bars will be determined from the length of the colour list. Default values forself._bar_solid_percent
, self._background_color
, self._padding
are also set.
1
class
_Bar
(
QtWidgets
.
QWidget
):
2
clickedValue
=
QtCore
.
pyqtSignal
(
int
)
3
4
def
__init__
(
self
,
steps
,
*
args
,
**
kwargs
):
5
super
()
.
__init__
(
*
args
,
**
kwargs
)
6
7
self
.
setSizePolicy
(
8
QtWidgets
.
QSizePolicy
.
MinimumExpanding
,
9
QtWidgets
.
QSizePolicy
.
MinimumExpanding
10
)
11
12
if
isinstance
(
steps
,
list
):
13
# list of colours.
14
self
.
n_steps
=
len
(
steps
)
15
self
.
steps
=
steps
16
17
elif
isinstance
(
steps
,
int
):
18
# int number of bars, defaults to red.
19
self
.
n_steps
=
steps
20
self
.
steps
=
[
'red'
]
*
steps
21
22
else
:
23
raise
TypeError
(
'steps must be a list or int'
)
24
25
self
.
_bar_solid_percent
=
0.8
26
self
.
_background_color
=
QtGui
.
QColor
(
'black'
)
27
self
.
_padding
=
4.0
# n-pixel gap around edge.
Likewise we update the PowerBar.__init__
to accept the steps parameter, and pass it through.
1
class
PowerBar
(
QtWidgets
.
QWidget
):
2
def
__init__
(
self
,
steps
=
5
,
*
args
,
**
kwargs
):
3
super
()
.
__init__
(
*
args
,
**
kwargs
)
4
5
layout
=
QtWidgets
.
QVBoxLayout
()
6
self
.
_bar
=
_Bar
(
steps
)
7
8
#...continued as before.
We now have the parameters in place to update the paintEvent
method. The modified code is shown below.
1
def
paintEvent
(
self
,
e
):
2
painter
=
QtGui
.
QPainter
(
self
)
3
4
brush
=
QtGui
.
QBrush
()
5
brush
.
setColor
(
self
.
_background_color
)
6
brush
.
setStyle
(
Qt
.
SolidPattern
)
7
rect
=
QtCore
.
QRect
(
0
,
0
,
painter
.
device
()
.
width
(),
painter
.
device
()
.
he
\
8
ight
())
9
painter
.
fillRect
(
rect
,
brush
)
10
11
# Get current state.
12
parent
=
self
.
parent
()
13
vmin
,
vmax
=
parent
.
minimum
(),
parent
.
maximum
()
14
value
=
parent
.
value
()
15
16
# Define our canvas.
17
d_height
=
painter
.
device
()
.
height
()
-
(
self
.
_padding
*
2
)
18
d_width
=
painter
.
device
()
.
width
()
-
(
self
.
_padding
*
2
)
19
20
# Draw the bars.
21
step_size
=
d_height
/
self
.
n_steps
22
bar_height
=
step_size
*
self
.
_bar_solid_percent
23
bar_spacer
=
step_size
*
(
1
-
self
.
_bar_solid_percent
)
/
2
24
25
# Calculate the y-stop position, from the value in range.
26
pc
=
(
value
-
vmin
)
/
(
vmax
-
vmin
)
27
n_steps_to_draw
=
int
(
pc
*
self
.
n_steps
)
28
29
for
n
in
range
(
n_steps_to_draw
):
30
brush
.
setColor
(
QtGui
.
QColor
(
self
.
steps
[
n
]))
31
rect
=
QtCore
.
QRect
(
32
self
.
_padding
,
33
self
.
_padding
+
d_height
-
((
1
+
n
)
*
step_size
)
+
bar_spacer
,
34
d_width
,
35
bar_height
36
)
37
painter
.
fillRect
(
rect
,
brush
)
38
39
painter
.
end
()
You can now experiment with passing in different values for the init to PowerBar
, e.g. increasing the number of bars, or providing a colour list. Some examples are shown below — a good source of hex palettes is the Bokeh source.
1
PowerBar
(
10
)
2
PowerBar
(
3
)
3
PowerBar
([
"#5e4fa2"
,
"#3288bd"
,
"#66c2a5"
,
"#abdda4"
,
"#e6f598"
,
"#ffffbf"
,
"#f
\
4
ee08b"
,
"#fdae61"
,
"#f46d43"
,
"#d53e4f"
,
"#9e0142"
])
5
PowerBar
([
"#a63603"
,
"#e6550d"
,
"#fd8d3c"
,
"#fdae6b"
,
"#fdd0a2"
,
"#feedde"
])
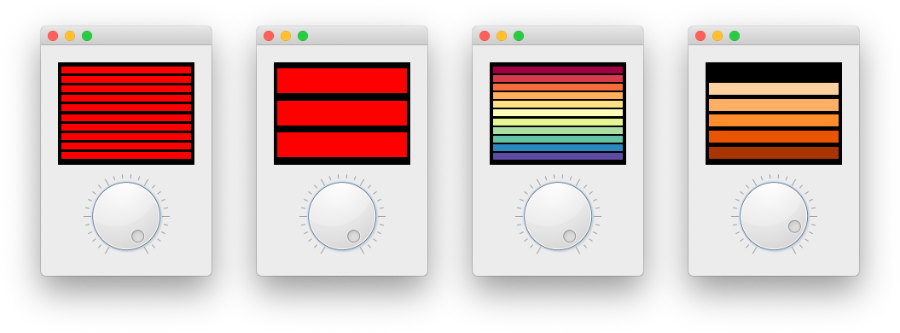
You could fiddle with the padding settings through the variables e.g. self._bar_solid_percent
but it’d be nicer to provide proper methods to set these.
N> We’re following the Qt standard of camelCase method names for these external methods for consistency with the others inherited from QDial
.
1
def
setColor
(
self
,
color
):
2
self
.
_bar
.
steps
=
[
color
]
*
self
.
_bar
.
n_steps
3
self
.
_bar
.
update
()
4
5
def
setColors
(
self
,
colors
):
6
self
.
_bar
.
n_steps
=
len
(
colors
)
7
self
.
_bar
.
steps
=
colors
8
self
.
_bar
.
update
()
9
10
def
setBarPadding
(
self
,
i
):
11
self
.
_bar
.
_padding
=
int
(
i
)
12
self
.
_bar
.
update
()
13
14
def
setBarSolidPercent
(
self
,
f
):
15
self
.
_bar
.
_bar_solid_percent
=
float
(
f
)
16
self
.
_bar
.
update
()
17
18
def
setBackgroundColor
(
self
,
color
):
19
self
.
_bar
.
_background_color
=
QtGui
.
QColor
(
color
)
20
self
.
_bar
.
update
()
In each case we set the private variable on the _bar
object and then call _bar.update()
to trigger a redraw of the widget. The method support changing the colour to a single colour, or updating a list of them — setting a list of colours can also be used to change the number of bars.
N> There is no method to set the bar count, since expanding a list of colours would be faffy. But feel free to try adding this yourself!
Here’s an example using 25px padding, a fully solid bar and a grey background.
1
bar
=
PowerBar
([
"#49006a"
,
"#7a0177"
,
"#ae017e"
,
"#dd3497"
,
"#f768a1"
,
"#fa9fb5
\
2
"
,
"#fcc5c0"
,
"#fde0dd"
,
"#fff7f3"
])
3
bar
.
setBarPadding
(
2
)
4
bar
.
setBarSolidPercent
(
0.9
)
5
bar
.
setBackgroundColor
(
'gray'
)
With these settings you get the following result.
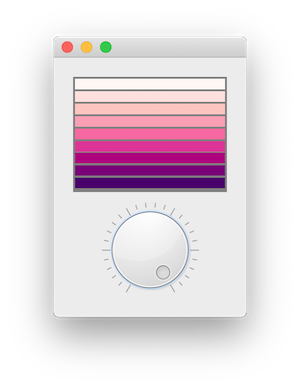
Adding the QAbstractSlider Interface
We’ve added methods to configure the behaviour of the power bar. But we currently provide no way to configure the standard QDial
methods — for example, setting the min, max or step size — from our widget. We could work through and add wrapper methods for all of these, but it would get very tedious very quickly.
1
# Example of a single wrapper, we'd need 30+ of these.
2
def
setNotchesVisible
(
self
,
b
):
3
return
self
.
_dial
.
setNotchesVisible
(
b
)
Instead we can add a little handler onto our outer widget to automatically look for methods (or attributes) on the QDial
instance, if they don’t exist on our class directly. This way we can implement our own methods, yet still get all the QAbstractSlider
goodness for free.
The wrapper is shown below, implemented as a custom __getattr__
method.
1
def
__getattr__
(
self
,
name
):
2
if
name
in
self
.
__dict__
:
3
return
self
[
name
]
4
5
try
:
6
return
getattr
(
self
.
_dial
,
name
)
7
except
AttributeError
:
8
raise
AttributeError
(
9
"'{}' object has no attribute '{}'"
.
format
(
self
.
__class__
.
__name__
,
n
\
10
ame
)
11
)
When accessing a property (or method) — e.g. when when call PowerBar.setNotchesVisible(true)
Python internally uses __getattr__
to get the property from the current object. This handler does this through the object dictionary self.__dict__
. We’ve overridden this method to provide our custom handling logic.
Now, when we call PowerBar.setNotchesVisible(true)
, this handler first looks on our current object (a PowerBar
instance) to see if .setNotchesVisible
exists and if it does uses it. If not it then calls getattr()
on self._dial
instead returning what it finds there. This gives us access to all the methods of QDial
from our custom PowerBar
widget.
If QDial
doesn’t have the attribute either, and raises an AttributeError
we catch it and raise it again from our custom widget, where it belongs.
Updating from the Meter display
Currently you can update the current value of the PowerBar meter by twiddling with the dial. But it would be nice if you could also update the value by clicking a position on the power bar, or by dragging you mouse up and down. To do this we can update our _Bar
widget to handle mouse events.
1
class
_Bar
(
QtWidgets
.
QWidget
):
2
3
clickedValue
=
QtCore
.
pyqtSignal
(
int
)
4
5
# ... existing code ...
6
7
def
_calculate_clicked_value
(
self
,
e
):
8
parent
=
self
.
parent
()
9
vmin
,
vmax
=
parent
.
minimum
(),
parent
.
maximum
()
10
d_height
=
self
.
size
()
.
height
()
+
(
self
.
_padding
*
2
)
11
step_size
=
d_height
/
self
.
n_steps
12
click_y
=
e
.
y
()
-
self
.
_padding
-
step_size
/
2
13
14
pc
=
(
d_height
-
click_y
)
/
d_height
15
value
=
vmin
+
pc
*
(
vmax
-
vmin
)
16
self
.
clickedValue
.
emit
(
value
)
17
18
def
mouseMoveEvent
(
self
,
e
):
19
self
.
_calculate_clicked_value
(
e
)
20
21
def
mousePressEvent
(
self
,
e
):
22
self
.
_calculate_clicked_value
(
e
)
In the __init__
block for the PowerBar
widget we can connect to the _Bar.clickedValue
signal and send the values to self._dial.setValue
to set the current value on the dial.
1
# Take feedback from click events on the meter.
2
self
.
_bar
.
clickedValue
.
connect
(
self
.
_dial
.
setValue
)
If you run the widget now, you’ll be able to click around in the bar area and the value will update, and the dial rotate in sync.
The final code
Below is the complete final code for our PowerBar meter widget, called PowerBar
. You can save this over the previous file (e.g. named power_bar.py
) and then use it in any of your own projects, or customise it further to your own requirements.
1
from
PyQt5
import
QtCore
,
QtGui
,
QtWidgets
2
from
PyQt5.QtCore
import
Qt
3
4
5
class
_Bar
(
QtWidgets
.
QWidget
):
6
7
clickedValue
=
QtCore
.
pyqtSignal
(
int
)
8
9
def
__init__
(
self
,
steps
,
*
args
,
**
kwargs
):
10
super
()
.
__init__
(
*
args
,
**
kwargs
)
11
12
self
.
setSizePolicy
(
13
QtWidgets
.
QSizePolicy
.
MinimumExpanding
,
14
QtWidgets
.
QSizePolicy
.
MinimumExpanding
15
)
16
17
if
isinstance
(
steps
,
list
):
18
# list of colours.
19
self
.
n_steps
=
len
(
steps
)
20
self
.
steps
=
steps
21
22
elif
isinstance
(
steps
,
int
):
23
# int number of bars, defaults to red.
24
self
.
n_steps
=
steps
25
self
.
steps
=
[
'red'
]
*
steps
26
27
else
:
28
raise
TypeError
(
'steps must be a list or int'
)
29
30
self
.
_bar_solid_percent
=
0.8
31
self
.
_background_color
=
QtGui
.
QColor
(
'black'
)
32
self
.
_padding
=
4.0
# n-pixel gap around edge.
33
34
def
paintEvent
(
self
,
e
):
35
painter
=
QtGui
.
QPainter
(
self
)
36
37
brush
=
QtGui
.
QBrush
()
38
brush
.
setColor
(
self
.
_background_color
)
39
brush
.
setStyle
(
Qt
.
SolidPattern
)
40
rect
=
QtCore
.
QRect
(
0
,
0
,
painter
.
device
()
.
width
(),
painter
.
device
()
.
he
\
41
ight
())
42
painter
.
fillRect
(
rect
,
brush
)
43
44
# Get current state.
45
parent
=
self
.
parent
()
46
vmin
,
vmax
=
parent
.
minimum
(),
parent
.
maximum
()
47
value
=
parent
.
value
()
48
49
# Define our canvas.
50
d_height
=
painter
.
device
()
.
height
()
-
(
self
.
_padding
*
2
)
51
d_width
=
painter
.
device
()
.
width
()
-
(
self
.
_padding
*
2
)
52
53
# Draw the bars.
54
step_size
=
d_height
/
self
.
n_steps
55
bar_height
=
step_size
*
self
.
_bar_solid_percent
56
bar_spacer
=
step_size
*
(
1
-
self
.
_bar_solid_percent
)
/
2
57
58
# Calculate the y-stop position, from the value in range.
59
pc
=
(
value
-
vmin
)
/
(
vmax
-
vmin
)
60
n_steps_to_draw
=
int
(
pc
*
self
.
n_steps
)
61
62
for
n
in
range
(
n_steps_to_draw
):
63
brush
.
setColor
(
QtGui
.
QColor
(
self
.
steps
[
n
]))
64
rect
=
QtCore
.
QRect
(
65
self
.
_padding
,
66
self
.
_padding
+
d_height
-
((
1
+
n
)
*
step_size
)
+
bar_spacer
,
67
d_width
,
68
bar_height
69
)
70
painter
.
fillRect
(
rect
,
brush
)
71
72
painter
.
end
()
73
74
def
sizeHint
(
self
):
75
return
QtCore
.
QSize
(
40
,
120
)
76
77
def
_trigger_refresh
(
self
):
78
self
.
update
()
79
80
def
_calculate_clicked_value
(
self
,
e
):
81
parent
=
self
.
parent
()
82
vmin
,
vmax
=
parent
.
minimum
(),
parent
.
maximum
()
83
d_height
=
self
.
size
()
.
height
()
+
(
self
.
_padding
*
2
)
84
step_size
=
d_height
/
self
.
n_steps
85
click_y
=
e
.
y
()
-
self
.
_padding
-
step_size
/
2
86
87
pc
=
(
d_height
-
click_y
)
/
d_height
88
value
=
vmin
+
pc
*
(
vmax
-
vmin
)
89
self
.
clickedValue
.
emit
(
value
)
90
91
def
mouseMoveEvent
(
self
,
e
):
92
self
.
_calculate_clicked_value
(
e
)
93
94
def
mousePressEvent
(
self
,
e
):
95
self
.
_calculate_clicked_value
(
e
)
96
97
98
class
PowerBar
(
QtWidgets
.
QWidget
):
99
"""
100
Custom Qt Widget to show a power bar and dial.
101
Demonstrating compound and custom-drawn widget.
102
103
Left-clicking the button shows the color-chooser, while
104
right-clicking resets the color to None (no-color).
105
"""
106
107
colorChanged
=
QtCore
.
pyqtSignal
()
108
109
def
__init__
(
self
,
steps
=
5
,
*
args
,
**
kwargs
):
110
super
()
.
__init__
(
*
args
,
**
kwargs
)
111
112
layout
=
QtWidgets
.
QVBoxLayout
()
113
self
.
_bar
=
_Bar
(
steps
)
114
layout
.
addWidget
(
self
.
_bar
)
115
116
# Create the QDial widget and set up defaults.
117
# - we provide accessors on this class to override.
118
self
.
_dial
=
QtWidgets
.
QDial
()
119
self
.
_dial
.
setNotchesVisible
(
True
)
120
self
.
_dial
.
setWrapping
(
False
)
121
self
.
_dial
.
valueChanged
.
connect
(
self
.
_bar
.
_trigger_refresh
)
122
123
# Take feedback from click events on the meter.
124
self
.
_bar
.
clickedValue
.
connect
(
self
.
_dial
.
setValue
)
125
126
layout
.
addWidget
(
self
.
_dial
)
127
self
.
setLayout
(
layout
)
128
129
def
__getattr__
(
self
,
name
):
130
if
name
in
self
.
__dict__
:
131
return
self
[
name
]
132
133
return
getattr
(
self
.
_dial
,
name
)
134
135
def
setColor
(
self
,
color
):
136
self
.
_bar
.
steps
=
[
color
]
*
self
.
_bar
.
n_steps
137
self
.
_bar
.
update
()
138
139
def
setColors
(
self
,
colors
):
140
self
.
_bar
.
n_steps
=
len
(
colors
)
141
self
.
_bar
.
steps
=
colors
142
self
.
_bar
.
update
()
143
144
def
setBarPadding
(
self
,
i
):
145
self
.
_bar
.
_padding
=
int
(
i
)
146
self
.
_bar
.
update
()
147
148
def
setBarSolidPercent
(
self
,
f
):
149
self
.
_bar
.
_bar_solid_percent
=
float
(
f
)
150
self
.
_bar
.
update
()
151
152
def
setBackgroundColor
(
self
,
color
):
153
self
.
_bar
.
_background_color
=
QtGui
.
QColor
(
color
)
154
self
.
_bar
.
update
()
You should be able to use many of these ideas in creating your own custom widgets. For more examples, take a look at the Learn PyQt widget library — these widgets are all open source and freely available to use in your own projects.
The complete book
Thankyou for downloading this sample of Create Simple GUI Applications
If you like what you see you can purchase the complete book, together with an optional video course, at: https://www.learnpyqt.com/purchase
The complete book contains the following chapters —
- Installation and Getting Started
- Basic Qt Features
- Qt Creator & Qt Designer
- Extended Signals
- Bitmap Graphics, including a mini Paint-clone app
- Custom Widgets, for full customisability in your applications
- Model View Architecture, building MVC applications in PyQt5, including an example Todo app
- Multithreading, supporting concurrent execution in PyQt5 apps
- Example PyQt5 apps, including a Web Browser and Minesweeper Clone
- Packaging and Distribution
- Resources
The full book covers many more aspects of developing with PyQt, from getting started with the Qt Creator to multithreading advanced applications. All purchases come with free updates as the book is developed and expands.
For latest tutorials, tips and code samples see https://www.learnpyqt.com/
Notes
1Not really that simple.↩