Table of Contents
Let’s begin
Hello, I am Thomas Mak.
This is a book with examples and solutions to create practical visual effects on web by using CSS3.
The purpose of this book is to let you use the effect right away. You can directly use the code examples in your web projects.
You can find all the code examples in the Codepen collection:
https://css3effects.com/code
There are 6 chapters of the book. Each chapter is a theme. They are:
- Misc tips and tricks to get started
- Creating shapes
- Drawing with gradients
- Skeuomorphic and depth
- 3D effects
- Animation
In each chapter, there are different sections covering one topic. For example, drawing lines, linear gradient techniques, making realistic buttons.
In each section, we have fixed format:
- First, there are Code snippet for quick take away if you just want to clone the effect. Each code snippet comes with a link to online editor for interactive demo and quick editing.
- Then we have How it works to explain how the code combinations create the effects.
- Next, we have further discussions on When to use the effect which we create different variants. This section allows you to use the code examples into different kind of real world situations.
About Author
Thomas Mak, a.k.a. Makzan on internet, has been working in web design and development industry for more than 15 years. He write several books about multiple player virtual world and HTML5 mini games.
You can find me on internet:
- Website: makzan.net
- Twitter: @makzan
- Github: @makzan
- Codepen: @makzan
Thomas Mak is an award Winning trainer. Thomas Mak won a bronze medal in web design in WorldSkills 2003. Worldskills is an international skills competitions. Later, Thomas returned to WorldSkills as a mentor and has been training candidates to win gold, silver and bronze medal among competitors all over the world since 2009.
Thomas Mak has written several books. He wrote Multiplayer Flash Virtual World, HTML5 Games Development: Beginner’s Guide, HTML5 Games Development Hotshot, Flexbox Website.
Latest Version Update
Version 0.1—Finalizing the chapter headings In this version, I focus on adding chapter headings to finalize the content structure.
What’s expected in next update ?
In next version, I fill in the content and code example links into the book. Then, I can work on each code example and write the explanation and variant usage discussions.
Chapter 1—Misc Tips & Tricks to get started
Here are some tips and tricks that don’t feet into the 5 topics, but still worth sharing.
In this book, we use CSS to create different effects. Often the effect mixes different techniques together, from the most basic properties to the advanced 3D transform and animation setup.
So in this section, we go through some basic techniques that we will use to compose the effects throughout the book.
Pseudo Elements
Pseudo elements are :before
and :after
that create extra elements
Pseudo elements :after
can be used to indicate extra information.
1
[
href
$=
".pdf"
]
:
after
{
2
content
:
' (PDF)'
;
3
}
We can make use of these 2 extra elements for styling purpose. For example, we will create animated underline effects with pseudo elements at chapter 2.
Customizing checkbox and radio button
We can customize the style of checkbox and radio button by using the label, ~
adjacent selector and :checked
pseudo class. This is by hiding the checkbox/radio and make use of the label to toggle the hidden checkbox/radio.

Code snippet
HTML
1
<
input
type
=
'radio'
id
=
'desktop-product'
name
=
'product'
value
=
'desktop'
>
2
<
label
for
=
'desktop-product'
>
Desktop
</
label
>
CSS
1
input
[
type
=
'radio'
]
{
2
display
:
none
;
3
}
4
input
[
type
=
'radio'
]
+
label
{
5
display
:
block
;
6
font-size
:
1
rem
;
7
padding
:
1
rem
.5
rem
;
8
padding-left
:
3
rem
;
9
border
:
1
px
solid
transparent
;
10
}
11
input
[
type
=
'radio'
]
:
checked
+
label
{
12
border-color
:
YELLOWGREEN
;
13
background
:
#fdfefb
url
(
https://css3effects.com/tick.png
)
10
px
50
%
no-repeat
;
14
}
How it works
1
input
[
type
=
checkbox
]
{
2
display
:
none
;
3
}
4
input
[
type
=
checkbox
]~
label
{
5
/* Our normal style here */
6
}
7
input
[
type
=
checkbox
]
:
checked
~
label
{
8
/* Our checked style here */
9
}
Creating a file list with checkbox and radio
Another example, we can use checkbox and radio to create folder toggling and file selection.
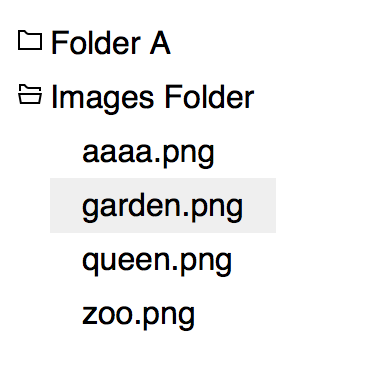
For each folder, their open state is toggled individually. And for file, assuming we allow single file editing, the files will be radio. Otherwise, the files will be checkbox too.
This technique makes use of the browser behavior so we don’t need to program the toggling ourself. We only need to style them up.
Text highlight effects
In this effect, we use the border-radius
to create something unusual.
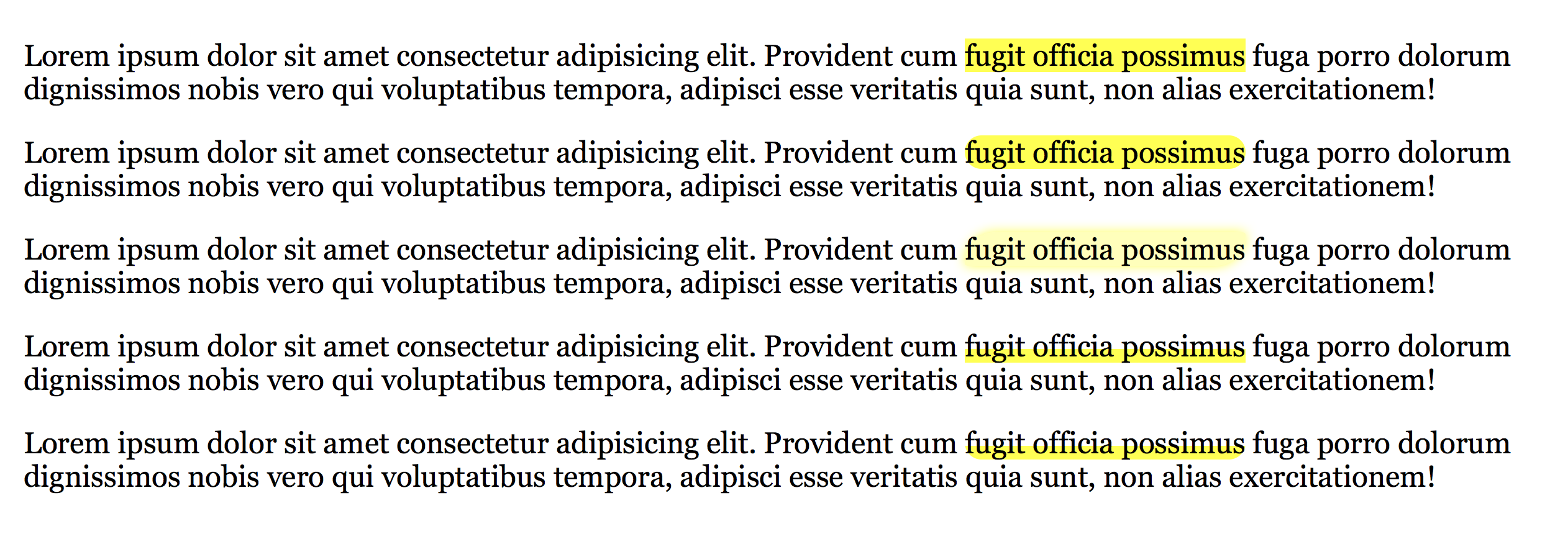
https://css3effects.com/text-highlight
Code snippet
They are all <mark>
tags but with different classes for demo purpose.
CSS
1
.
demo-1
mark
{
2
/* No styles, default mark tag */
3
}
4
5
.
demo-2
mark
{
6
border-radius
:
30
px
;
7
}
8
9
.
demo-3
mark
{
10
background
:
rgba
(
255
,
255
,
0
,
.4
);
11
border-radius
:
60
px
5
px
;
12
box-shadow
:
0
0
10
px
yellow
;
13
}
14
15
.
demo-4
mark
{
16
background
:
linear-gradient
(
to
bottom
,
transparent
60
%
,
yellow
60
%
);
17
}
18
19
.
demo-5
mark
{
20
background
:
linear-gradient
(
to
bottom
,
transparent
60
%
,
yellow
60
%
);
21
border-radius
:
50
px
;
22
}
Box Shadow basic
The most common shadow is a shadow with x-offset, y-offset, blur and color.
1
box-shadow
:
3px
3px
5px
black
;
Code snippet
There are optional values being omitted here. They are inset
and spread
. The inset
allows us to create realistic button switch. It creates an illusion of button that is lower that normal level.
The spread
value allows us to expand or scale down the shadow effect. It is not common but we can use the spread
to create interesting effects.
In chapter 2, Drawing shapes, we will use box-shadow to create pixel art effects. In chapter 4, Skeuomorphic and Design, we will also use box-shadow to create different realistic effects.
Using transition
Usually we put transition in normal selector. In such case the transition applies to both mouse in and out.
By using the transition, all the numeric value are changed over the given transition duration.
Numeric value includes position, dimension, color.
But it the way, it is slow to transit top, left, width and height. We can use transform to move, scale and rotate an element with good performance.
Throughout the book, we’ll go through different techniques, which we enhance them by adding transition.
Chapter 2—Drawing Shapes
We can create shapes via CSS3.
We can draw:
- Circles
- Triangle
- Pie
- Tabs
- Pillow
- Irregular Circles
By using transition
, we can also change the shape when mouse hovers or event occurs.
Drawing triangle
The triangle is done via border-color
.
The size of the triangle depends on the border-width
. The direction of the triangle depends on which side of the border has color while the others has transparent.
Code snippets
The follow code snippet creates a triangle.
1
.
demo
{
2
width
:
0
;
3
height
:
0
;
4
border
:
30
px
solid
transparent
;
5
border-bottom-color
:
gold
;
6
}
The following screenshot shows the result:
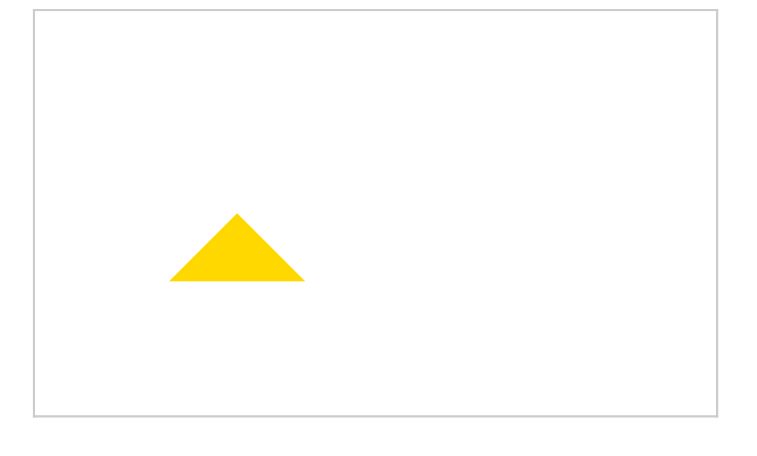
https://css3effects.com/triangle
How it work
This is a trick on the borders. We can define different colors for each border.
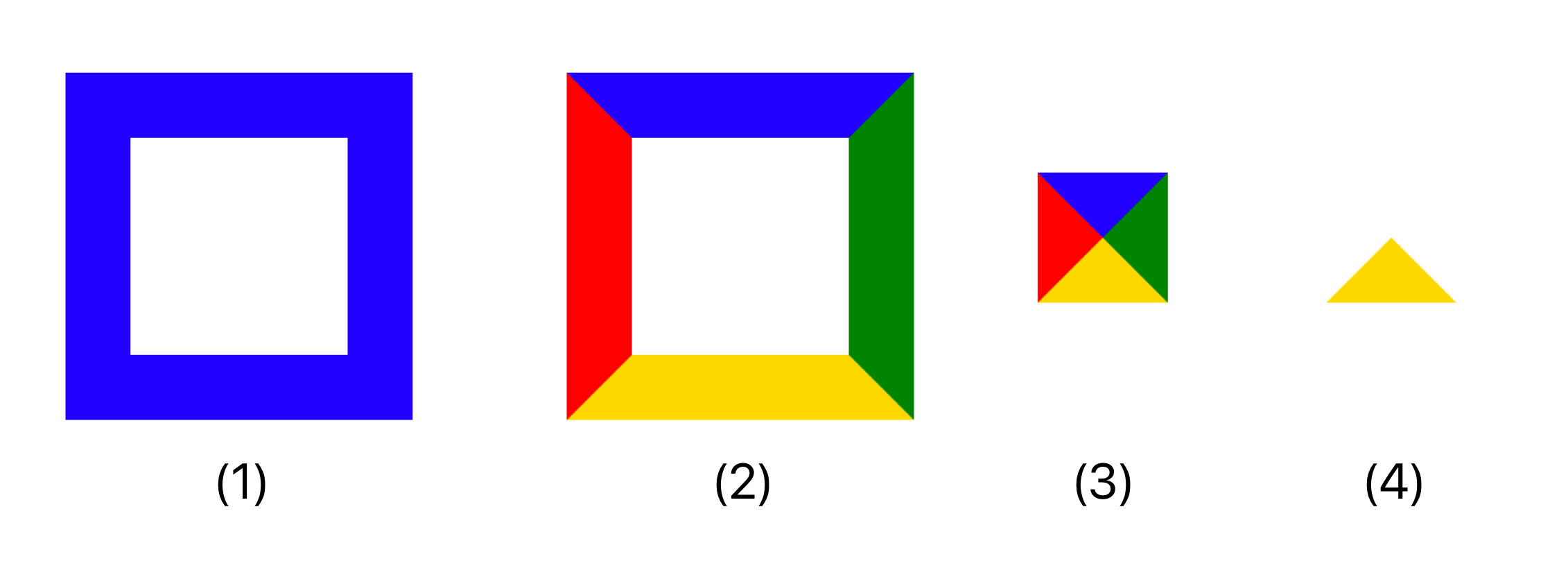
- We make a normal element with border.
- We set different colors to each border.
- We get rid of the width and height.
- We make 3 of the borders transparent.
If we want different direction of the triangle, we can simply set different border to be filled and keep the rest of the borders transparent.
You can also find the interactive steps in the following URL:
https://codepen.io/makzan/pen/qYrbvX
Chat bubble

https://css3effects.com/chat-bubble
1
<
div
class
=
"chat-bubble"
>
2
Hello
,
how
are
you
?
3
</
div
>
CSS
1
.
chat-bubble
{
2
margin-bottom
:
10
px
;
3
background
:
gold
;
4
padding
:
10
px
;
5
position
:
relative
;
6
border-radius
:
5
px
;
7
}
8
.
chat-bubble
:
after
{
9
content
:
''
;
10
11
position
:
absolute
;
12
right
:
30
px
;
13
bottom
:
-20
px
;
14
15
width
:
0
px
;
16
height
:
0
px
;
17
border
:
10
px
solid
transparent
;
18
border-top-color
:
gold
;
19
}
Please note that the pseudo element must have the content
to be rendered by browser. So if we don’t need any content, we still need to set the content:''
to empty.
Drawing circles
We can draw circles with border-radius
on an element with same width and height dimension.
One key point is to make the element a square by setting width and height the same value. The 50% of border-radius
makes this square a circle.
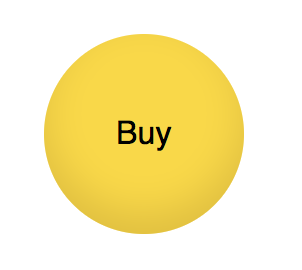
Code snippets
1
.
demo
{
2
width
:
100
px
;
3
height
:
100
px
;
4
background
:
gold
;
5
border-radius
:
50
%
;
6
}
How it works
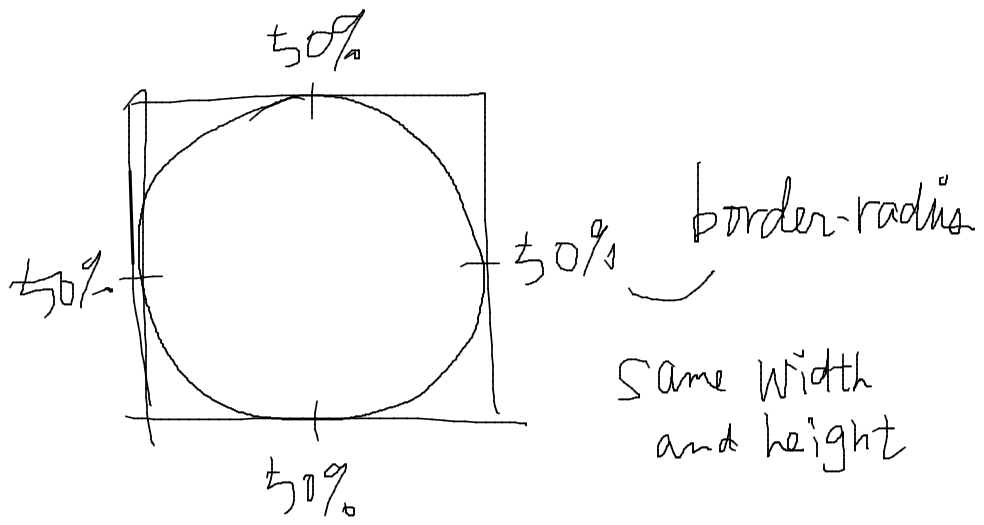
The 50% border-radius allows us to draw a full circle.
we can go beyond that, we can slightly adjust the border radius in 4 corners individually to make the circle feels like doodling.
Code example link:
https://css3effects.com/circle
1
<
div
class
=
"demo"
>
Buy
</
div
>
2
3
<
strong
>
Irregular
circles
</
strong
>
4
5
<
div
class
=
"demo circle2"
>
Buy
</
div
>
6
<
div
class
=
"demo circle3"
>
Buy
</
div
>
7
8
<
strong
>
Try
hover
this
👇
</
strong
>
9
<
div
class
=
"demo circle4"
>
Buy
</
div
>
CSS
1
.
demo
{
2
width
:
100
px
;
3
height
:
100
px
;
4
background
:
gold
;
5
border-radius
:
50
%
;
6
margin
:
2
em
0
;
7
line-height
:
100
px
;
8
text-align
:
center
;
9
cursor
:
pointer
;
10
}
Irregular Circle
1
.
circle2
{
2
border-radius
:
60
%
45
%
50
%
40
%
;
3
}
4
.
circle3
{
5
border-radius
:
45
%
55
%
50
%
60
%
;
6
}
7
8
.
circle4
{
9
border-radius
:
60
%
45
%
50
%
40
%
;
10
transition
:
all
.3
s
ease-out
;
11
}
12
.
circle4
:
hover
{
13
border-radius
:
50
%
55
%
45
%
60
%
;
14
}
Border image
To further enhance the doodling effect, we can use a dash or dotted borders. Furthermore, we can
Drawing pie
By combining the circle and triangle shape, we create a pie shape. It is still the triangle, but we apply border-radius
on it.
Code snippet
1
.
demo
{
2
width
:
0
;
3
height
:
0
;
4
border
:
30
px
solid
transparent
;
5
border-bottom-color
:
gold
;
6
border-radius
:
50
%
;
7
}
Result
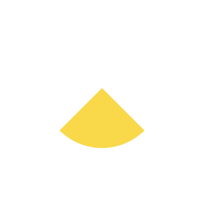
Code demo:
https://css3effects.com/pie
How it works
The size of shape depends on the border width. The width and height is set to zero.
Progress indicator with Pie shape
By combining these effects with pseudo elements, we can create a pie-shape progress indicator.
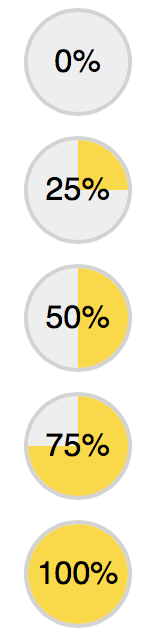
HTML
1
<
div
class
=
"progress p0"
>
0
%</
div
>
2
<
div
class
=
"progress p25"
>
25
%</
div
>
3
<
div
class
=
"progress p50"
>
50
%</
div
>
4
<
div
class
=
"progress p75"
>
75
%</
div
>
5
<
div
class
=
"progress p100"
>
100
%</
div
>
CSS
1
.
progress
{
2
width
:
50
px
;
3
height
:
50
px
;
4
border
:
2
px
solid
lightgray
;
5
border-radius
:
50
%
;
6
position
:
relative
;
7
text-align
:
center
;
8
line-height
:
50
px
;
9
}
10
.
progress
:
before
{
11
content
:
''
;
12
width
:
100
%
;
13
height
:
100
%
;
14
background
:
#eee
;
15
position
:
absolute
;
16
top
:
0
;
17
left
:
0
;
18
border-radius
:
50
%
;
19
z-index
:
-2
;
20
}
21
.
progress
:
after
{
22
content
:
''
;
23
width
:
0
;
24
height
:
0
;
25
border
:
25
px
solid
gold
;
26
position
:
absolute
;
27
top
:
0
;
28
left
:
0
;
29
border-radius
:
50
%
;
30
transform
:
rotate
(
45
deg
);
31
z-index
:
-1
;
32
}
33
.
progress
.
p0
:
after
{
34
border-color
:
transparent
;
35
}
36
.
progress
.
p25
:
after
{
37
border-left-color
:
transparent
;
38
border-bottom-color
:
transparent
;
39
border-right-color
:
transparent
;
40
}
41
.
progress
.
p50
:
after
{
42
border-left-color
:
transparent
;
43
border-bottom-color
:
transparent
;
44
}
45
.
progress
.
p75
:
after
{
46
border-left-color
:
transparent
;
47
}
48
49
.
progress
{
50
margin-top
:
5
px
;
51
margin-bottom
:
10
px
;
52
}
https://codepen.io/makzan/pen/LmMmOr
Drawing pixel art
We can use multiple box-shadows
to draw multiple color blocks. When these blocks are square pixel, they are like pixel art.
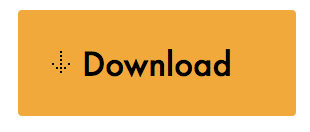
Code example link: https://css3effects.com/pixel-download
Code snippet
HTML
1
<
a
href
=
"#"
class
=
"download button"
>
Download
</
a
>
CSS
1
.
button
{
2
background
:
GOLD
;
3
padding
:
1
em
2
em
;
4
color
:
BLACK
;
5
text-decoration
:
none
;
6
border-radius
:
2
px
;
7
position
:
relative
;
8
}
9
.
button
:
hover
{
10
background
:
ORANGE
;
11
}
12
.
button
:
before
{
13
content
:
''
;
14
width
:
1
px
;
15
height
:
1
px
;
16
background
:
rgba
(
0
,
0
,
0
,
0
);
17
position
:
absolute
;
18
top
:
50
%
;
19
left
:
1.3
em
;
20
transition
:
all
0.3
s
ease-out
;
21
box-shadow
:
0
0
rgba
(
0
,
0
,
0
,
0
),
22
5
px
6
px
rgba
(
0
,
0
,
0
,
0
),
23
0
calc
(
6
px
*
2
)
rgba
(
0
,
0
,
0
,
0
),
24
6
px
6
px
rgba
(
0
,
0
,
0
,
0
),
25
calc
(
6
px
*
2
)
0
rgba
(
0
,
0
,
0
,
0
),
26
-6
px
6
px
rgba
(
0
,
0
,
0
,
0
),
27
calc
(
-6
px
*
3
)
0
rgba
(
0
,
0
,
0
,
0
),
28
0
-6
px
rgba
(
0
,
0
,
0
,
0
),
29
-3
px
calc
(
-6
px
*
2
)
rgba
(
0
,
0
,
0
,
0
),
30
3
px
calc
(
-6
px
*
4
)
rgba
(
0
,
0
,
0
,
0
);
31
}
32
.
button
:
hover
:
before
{
33
background
:
rgba
(
0
,
0
,
0
,
1
);
34
box-shadow
:
0
2
px
,
0
calc
(
2
px
*
2
),
35
2
px
2
px
,
calc
(
2
px
*
2
)
0
,
36
-2
px
2
px
,
calc
(
-2
px
*
2
)
0
,
37
0
-2
px
,
0
calc
(
-2
px
*
2
),
38
0
calc
(
-2
px
*
3
);
39
}
How it works
The box-shadow
allows multiple shadows separated with commas. By using 0px blur shadow on square element, we can create solid color blocks that simulate pixels.
When to use this effect?
Obviously it is unrealistic to create a large master piece of pixel art. But we can use this technique to create tiny pixel buttons.
Creating tab
1
.
tab-demo
li
{
2
border-radius
:
5
px
5
px
0
0
;
3
}
Creating tabs 2
Border radius

Code snippet
HTML
1
<
ul
class
=
"tab"
>
2
<
li
><
a
class
=
"active"
href
=
"#"
>
HTML
</
a
></
li
>
3
<
li
><
a
href
=
"#"
>
CSS
</
a
></
li
>
4
<
li
><
a
href
=
"#"
>
JS
</
a
></
li
>
5
<
li
><
a
href
=
"#"
>
PHP
</
a
></
li
>
6
</
ul
>
CSS
1
.
tab
{
2
list-style
:
none
;
3
margin
:
0
;
4
padding
:
0
;
5
}
6
7
ul
.
tab
li
{
8
display
:
inline
;
9
margin
:
0
;
10
margin-left
:
-10
px
;
11
background
:
none
;
12
}
13
ul
.
tab
a
{
14
padding
:
15
px
;
15
padding-top
:
5
px
;
16
padding-bottom
:
5
px
;
17
color
:
black
;
18
background
:
#efefef
;
19
text-decoration
:
none
;
20
border-top-left-radius
:
20
px
;
21
border-top-right-radius
:
5
px
;
22
border-bottom-left-radius
:
2
px
;
23
border-bottom-right-radius
:
2
px
;
24
/* display: inline-block;
25
transition: all .3s ease-out; */
26
}
27
ul
.
tab
a
:
hover
{
28
text-decoration
:
underline
;
29
box-shadow
:
inset
0
0
25
px
rgba
(
0
,
0
,
0
,
0.2
);
30
}
31
ul
.
tab
li
:
nth-child
(
1
)
a
{
32
background
:
pink
;
33
}
34
ul
.
tab
li
:
nth-child
(
2
)
a
{
35
background
:
salmon
;
36
}
37
ul
.
tab
li
:
nth-child
(
3
)
a
{
38
background
:
gold
;
39
}
40
ul
.
tab
li
:
nth-child
(
4
)
a
{
41
background
:
orange
;
42
}
43
ul
.
tab
a
.
active
{
44
font-weight
:
bold
;
45
}
More tabs variants
Animated underline effect
We can create an animated border by using pseudo-elements to simulate a border.
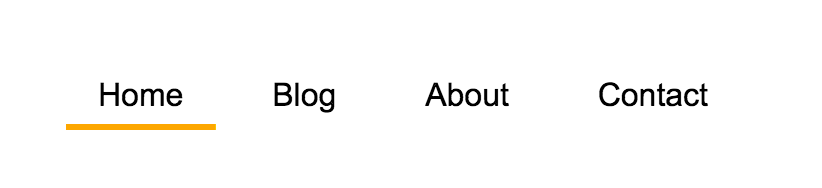
Code snippet
CSS
1
.
demo
{
2
display
:
inline-block
;
3
padding
:
.5
em
1
em
;
4
margin-right
:
.5
em
;
5
position
:
relative
;
6
color
:
black
;
7
text-decoration
:
none
;
8
overflow
:
hidden
;
9
}
10
11
.
demo
:
before
{
12
content
:
''
;
13
position
:
absolute
;
14
width
:
100
%
;
15
height
:
3
px
;
16
bottom
:
0
;
17
left
:
0
;
18
background
:
gold
;
19
transform
:
translateX
(
-100
%
);
20
transition
:
all
.2
s
ease-out
;
21
}
22
23
.
demo
:
before
{
24
background
:
orange
;
25
}
26
27
.
demo
:
hover
:
before
{
28
transform
:
translateX
(
0
%
);
29
}
How it works
https://codepen.io/makzan/pen/LmxVYr
Let’s animate 2 lines
1
*
{
box-sizing
:
border-box
;}
2
.
demo
{
3
display
:
inline-block
;
4
padding
:
.5
em
1
em
;
5
margin-right
:
.5
em
;
6
position
:
relative
;
7
color
:
black
;
8
text-decoration
:
none
;
9
overflow
:
hidden
;
10
}
11
.
demo
:
after
,
12
.
demo
:
before
{
13
content
:
''
;
14
position
:
absolute
;
15
width
:
100
%
;
16
height
:
3
px
;
17
bottom
:
0
;
18
left
:
0
;
19
background
:
gold
;
20
transform
:
translateX
(
-100
%
);
21
transition
:
all
.2
s
ease-out
;
22
}
23
24
.
demo
:
before
{
25
background
:
orange
;
26
}
27
.
demo
:
after
{
28
transition-duration
:
.4
s
;
29
transition-delay
:
.1
s
;
30
}
31
32
.
demo
:
hover
:
before
{
33
transform
:
translateX
(
0
%
);
34
}
35
.
demo
:
hover
:
after
{
36
transform
:
translateX
(
100
%
);
37
}
Float with shape-outside
Chapter 3—Drawing with Gradients
- Lines with linear gradient
- Patterns with gradients
Linear gradient basic
A basic gradient may look boring at first. But we can create many useful effects with gradients.
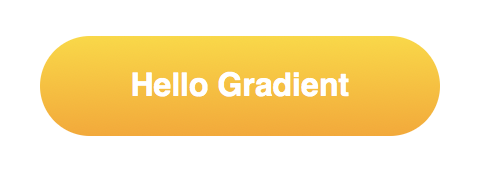
Code example link: https://css3effects.com/gradient
Code snippets
HTML
1
<
div
class
=
"demo"
>
2
Hello
Gradient
3
</
div
>
CSS
1
.
demo
{
2
background
:
linear-gradient
(
gold
,
orange
);
3
}
4
5
.
demo
{
6
width
:
200
px
;
7
height
:
50
px
;
8
border-radius
:
50
px
;
9
line-height
:
50
px
;
10
text-align
:
center
;
11
color
:
white
;
12
font-weight
:
bold
;
13
}
How it works
The most basic usage of gradient is to have two colors that one color blends into the other color.
When to use this effects?
Drawing lines with linear gradient
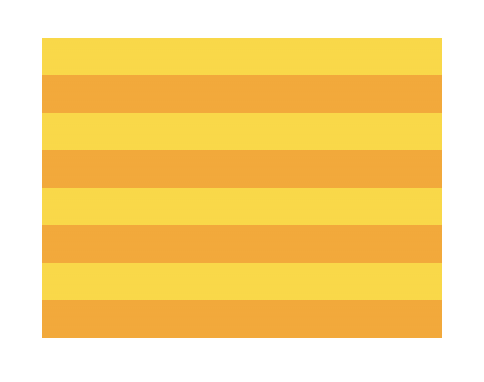
Code example link: https://css3effects.com/gradient-lines
Code snippets
HTML
1
<
div
class
=
"demo"
></
div
>
CSS
1
.
demo
{
2
background
:
linear-gradient
(
to
bottom
,
gold
50
%
,
orange
50
%
);
3
background-size
:
50
px
;
4
}
5
6
.
demo
{
7
width
:
200
px
;
8
height
:
150
px
;
9
}
How it works
When two steps of gradients have the same value, the color changes sharply instead of blending.
When to use this effect?
This is useful when we need to create a line. This lines may not be equal width. For example, it can be a border on the right or at the bottom. It can also be a diagonal line.
Subtle right border hover effects
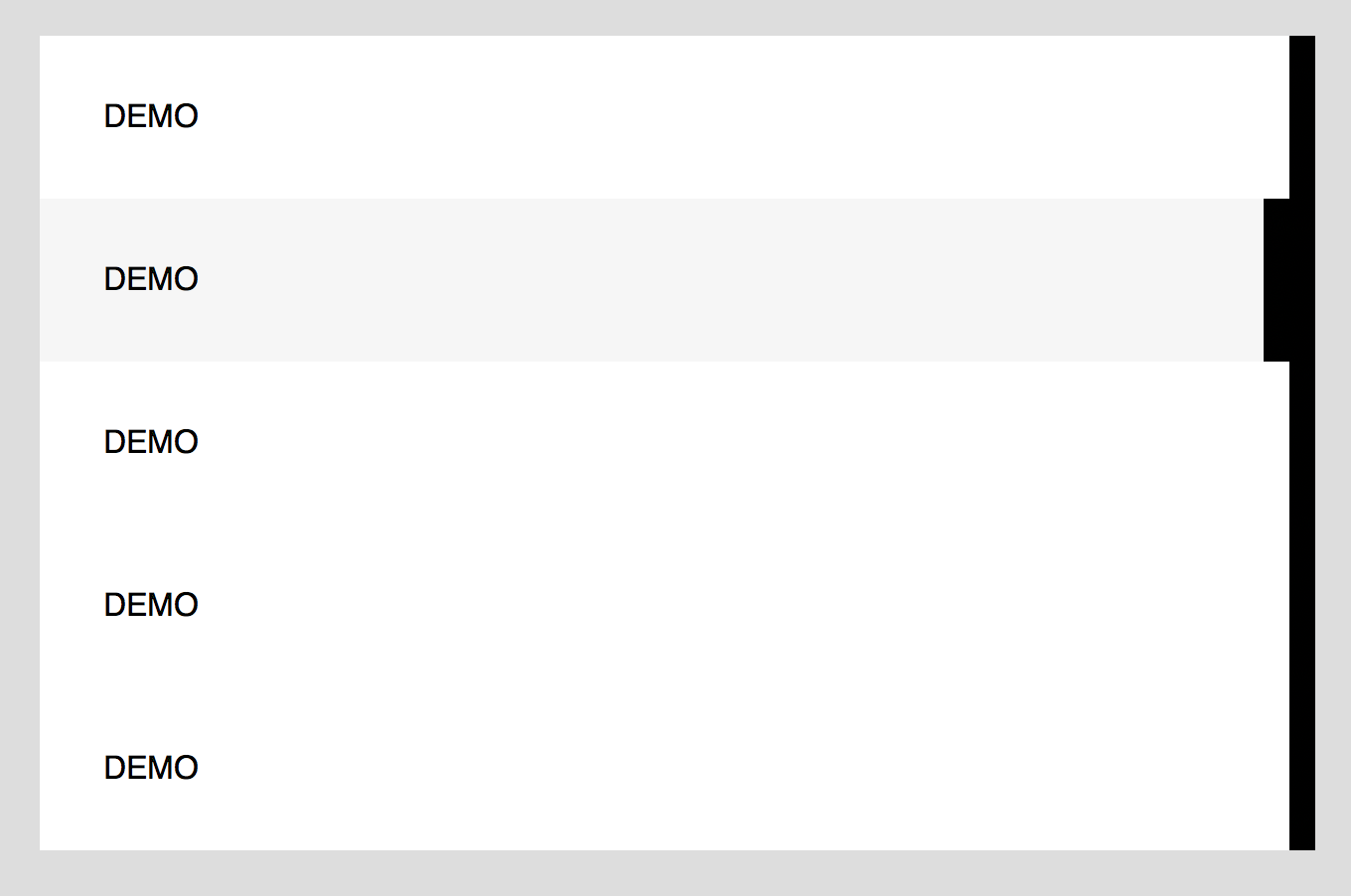
Drawing repeat patterns with gradient
By using linear-gradient
and repeating-linear-gradient
, we can create different kind of pattern backgrounds.
Code snippet
1
.
pattern-1
{
2
background
:
linear-gradient
(
45
deg
,
rgba
(
0
,
100
,
255
,
.2
)
3
px
,
transparent
3
px
);
3
background-size
:
30
px
30
px
;
4
}
How it works
The background-size allows us to make the gradient repeat via default background-repeat option.
More patterns
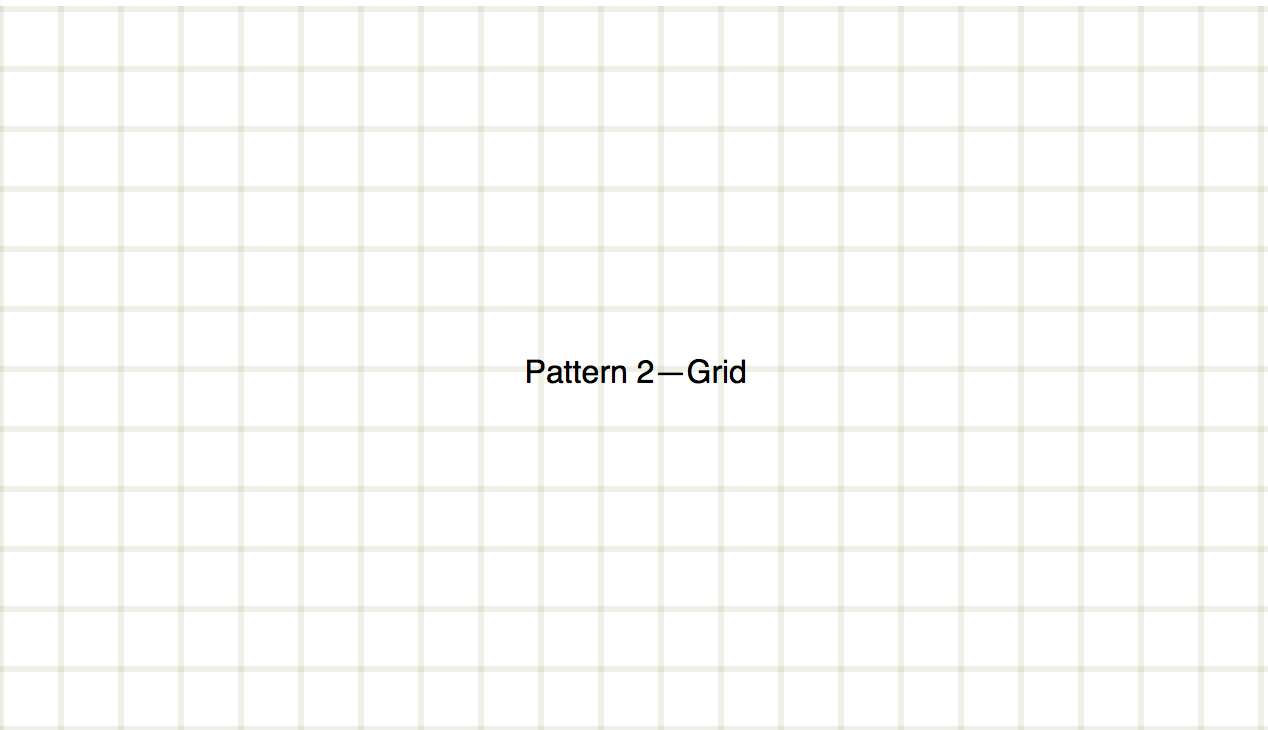
1
.
pattern-2
{
2
background
:
linear-gradient
(
to
right
,
rgba
(
100
,
100
,
0
,
.1
)
3
px
,
transparent
3
px
),
3
linear-gradient
(
to
bottom
,
rgba
(
100
,
100
,
0
,
.1
)
3
px
,
transparent
3
px
);
4
background-size
:
30
px
30
px
;
5
}
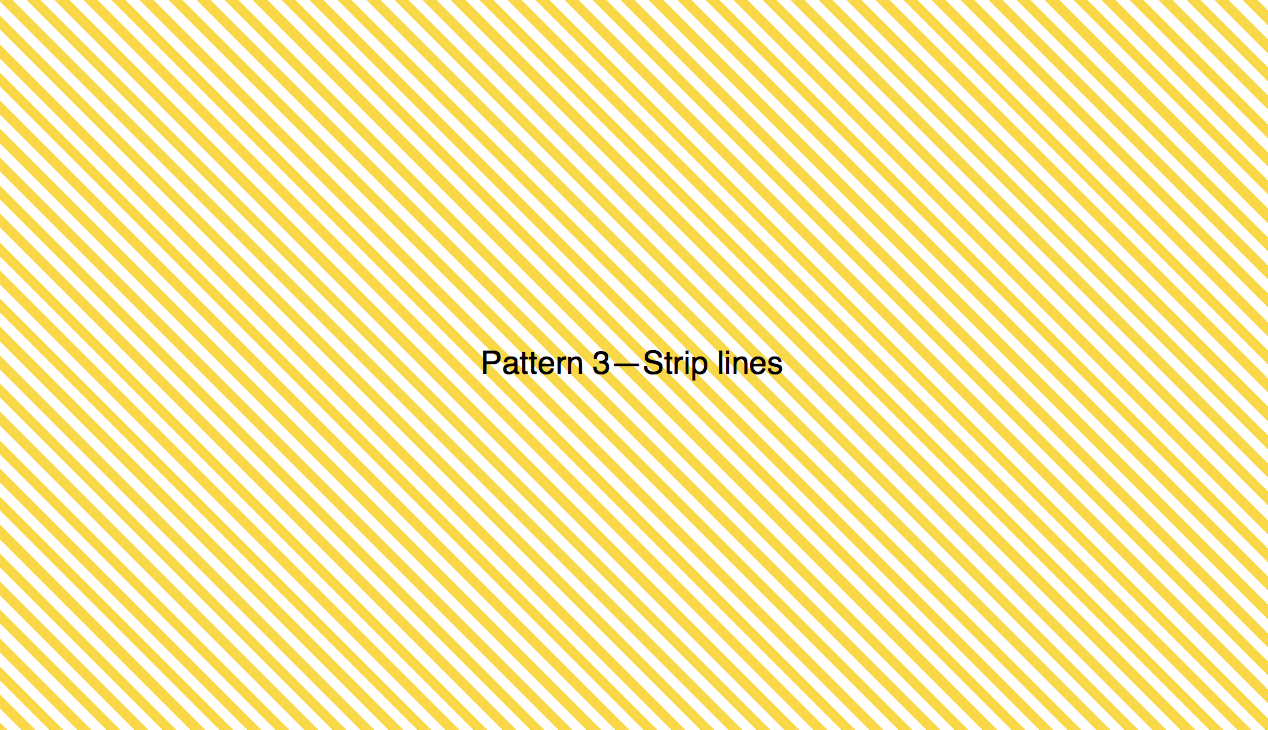
1
.
pattern-3
{
2
--
line-width
:
5
px
;
3
background
:
repeating-linear-gradient
(
45
deg
,
gold
,
gold
var
(
--
line
-
width
),
tra
\
4
nsparent
var
(
--
line
-
width
),
transparent
calc
(
var
(
--
line
-
width
)
*
2
)
);
5
}
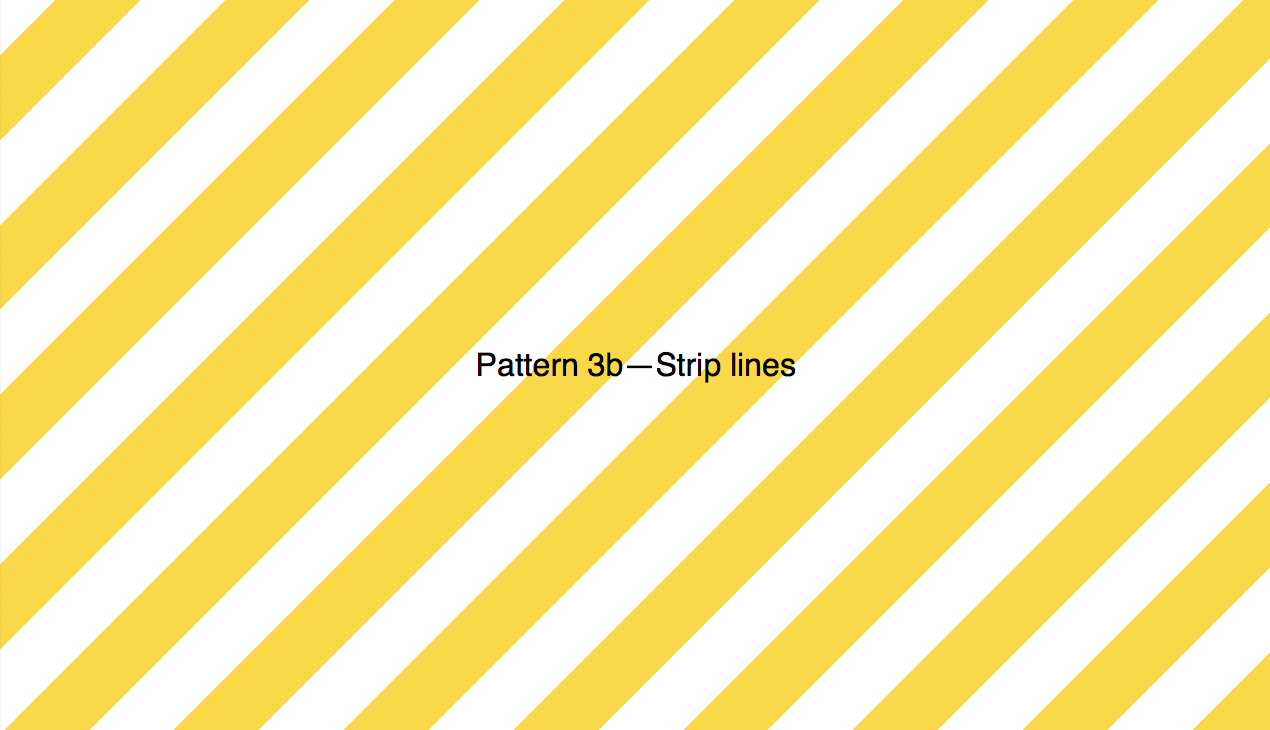
1
.
pattern-3b
{
2
--
line-width
:
30
px
;
3
background
:
repeating-linear-gradient
(
-45
deg
,
gold
,
gold
var
(
--
line
-
width
),
tr
\
4
ansparent
var
(
--
line
-
width
),
transparent
calc
(
var
(
--
line
-
width
)
*
2
)
);
5
}
Radial gradient basic
Code snippets
How it works
Drawing highlight with radial gradient
Code snippets
How it works
Compose with several linear gradient
CSS3 allows multiple backgrounds. Linear gradient is a background. So we can create multiple linear gradients.
Code snippets
How it works
Chapter 4—Skeuomorphic and Depth
- Box shadow
- Growing effects
- Inset shadow
Using shadow to create depth
One basic box shadow usage is to create depth illusion. In user interface, this is particular useful for overlay models and pop-up menus.
Code snippets
How it works
Drawing realistic buttons with box shadow
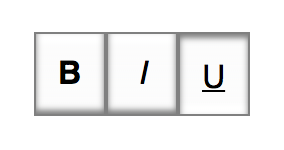
https://css3effects.com/inner-shadow-button
Code snippets
HTML
1
<
div
class
=
"demo"
>
2
<
a
href
=
"#"
class
=
"bold"
>
B
</
a
>
3
<
a
href
=
"#"
class
=
"italic"
>
I
</
a
>
4
<
a
href
=
"#"
class
=
"underline active"
>
U
</
a
>
5
</
div
>
CSS
1
/* Main style */
2
.
demo
a
{
3
box-shadow
:
inset
0
-2
px
3
px
rgba
(
0
,
0
,
0
,
.5
);
4
background
:
#fcfcfc
linear-gradient
(
to
bottom
,
white
3
px
,
#fcfcfc
6
px
);
5
}
6
.
demo
a
.
active
,
7
.
demo
a
:
active
{
8
box-shadow
:
inset
0
2
px
3
px
rgba
(
0
,
0
,
0
,
.5
);
9
line-height
:
45
px
;
10
background
:
#fcfcfc
linear-gradient
(
to
top
,
white
3
px
,
#fcfcfc
6
px
);
11
}
12
13
/* Making the button */
14
.
demo
a
{
15
display
:
inline-block
;
16
17
width
:
34
px
;
18
height
:
40
px
;
19
border
:
1
px
solid
grey
;
20
text-align
:
center
;
21
line-height
:
40
px
;
22
text-decoration
:
none
;
23
color
:
black
;
24
float
:
left
;
25
}
26
.
demo
a
.
bold
{
27
font-weight
:
bold
;
28
}
29
.
demo
a
.
italic
{
30
font-style
:
italic
;
31
}
32
.
demo
a
.
underline
{
33
text-decoration
:
underline
;
34
}
How it works
Light source
In real world, light casts shadow on surfaces. Assuming the light is from the top. The shadow at the bottom creates an elevation feeling. The shadow at the top create a concave effects.
Drawing realistic paper with box shadow and gradient
Code snippets
How it works
Drawing realistic notebook with box shadow and gradient
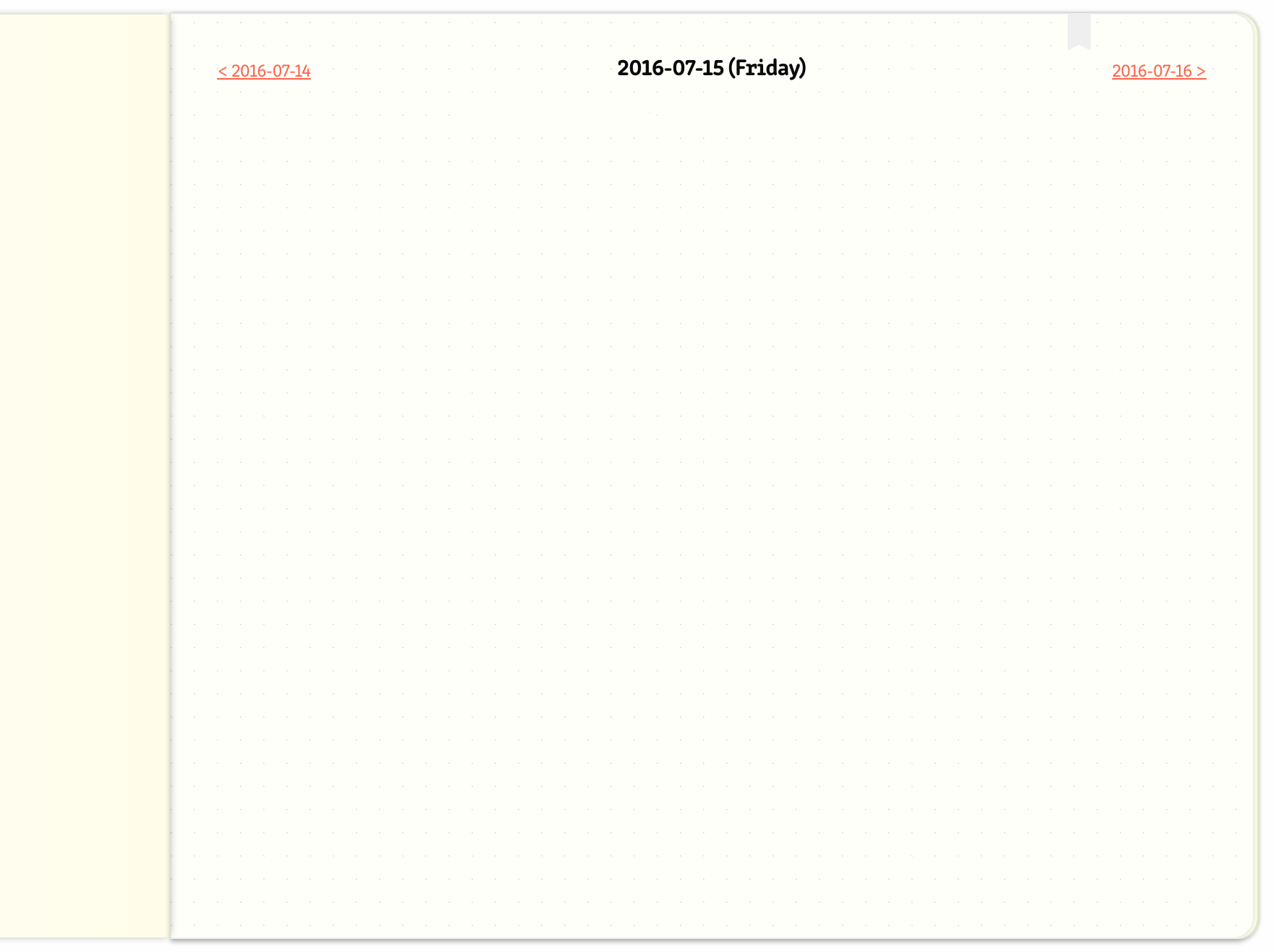
Code snippets
How it works
Grow effect with text shadow
By using text-shadow
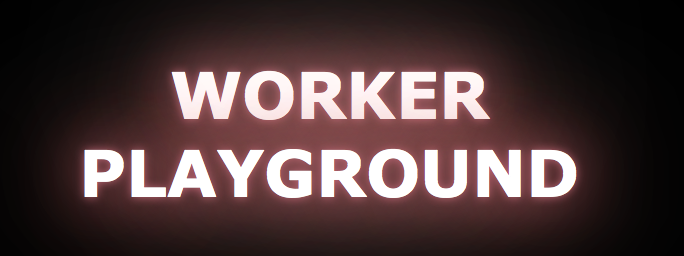
Code snippets
CSS
1
text-shadow
:
0
0
5px
rgb
(
206
,
97
,
117
),
0
0
90px
white
,
0
0
30px
rgb
(
206
,
97
,
117
),
\
2
0
0
50px
red
;
How it works
Chapter 5—3D Effects
3D effects main use the following properties
- transform: translate3d
Pop-up effects
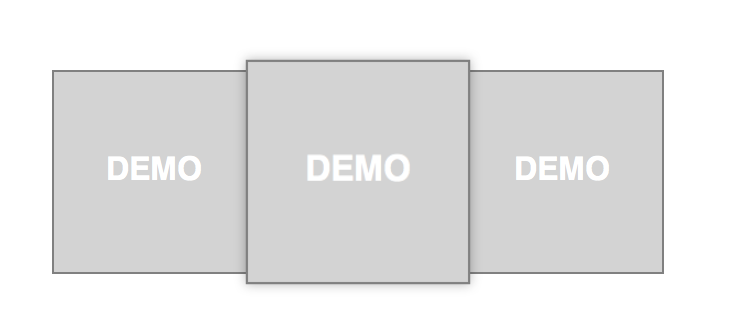
3D Button
We can use transform
, rotateX
and :hover
to create a 3D rotating button effect.

Code snippets
1
<
div
class
=
'button3d'
>
2
<
a
href
=
'#'
class
=
'button'
>
Hover
me
</
a
>
3
</
div
>
CSS
1
a
{
2
text-decoration
:
none
;
3
color
:
#222
;
4
}
5
6
.
button3d
{
7
perspective
:
600
px
;
8
cursor
:
pointer
;
9
10
.button
{
11
display
:
block
;
12
padding
:
1
rem
;
13
background
:
GOLD
;
14
text-align
:
center
;
15
16
transition
:
all
0.6
s
ease-out
;
17
}
18
19
&
:
hover
.
button
{
20
transform
:
rotateX
(
360
deg
);
21
transition
:
all
0.3
s
ease-out
;
22
}
23
24
}
Code example link: https://codepen.io/makzan/pen/Alytd
How it works
The container is to prevent the transition stops and struggle when the element is between hover and non-hover state.
Perspective
Usually we set the perspective value to be between 300 and 800.
3D Card Flipping Effect
By using backface-visibility:hidden
and 3D rotation, we can create a card flipping effect.
This is done by using 2 elements that acts as the .front.face
and .back.face
. They are put into a .card
container.
Code snippets
https://codepen.io/makzan/pen/vxCdA
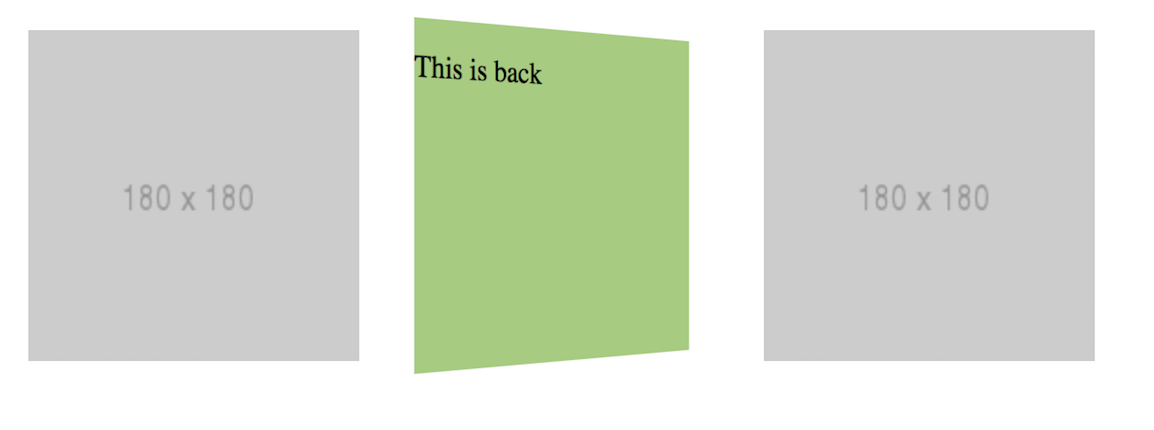
How it works
1
<
div
class
=
'card'
>
2
<
div
class
=
'front face'
>
3
<
img
src
=
'http://placehold.it/180x180'
/>
4
</
div
>
5
<
div
class
=
"back face"
>
6
<
p
>
This
is
back
</
p
>
7
</
div
>
8
</
div
>
CSS
1
.
card
{
2
width
:
180
px
;
3
height
:
180
px
;
4
position
:
relative
;
5
perspective
:
700
px
;
6
7
float
:
left
;
8
margin
:
10
px
;
9
}
10
.
card
:
hover
.
front
{
11
transform
:
rotateY
(
-180
deg
);
12
}
13
.
card
:
hover
.
back
{
14
transform
:
rotateY
(
0
deg
);
15
}
16
.
card
:
hover
.
face
{
17
transition
:
all
0.3
s
ease-out
;
18
}
19
.
face
{
20
position
:
absolute
;
21
top
:
0
;
22
left
:
0
;
23
width
:
100
%
;
24
height
:
100
%
;
25
backface-visibility
:
hidden
;
26
transition
:
all
0.8
s
ease-out
;
27
}
28
.
front
{
29
transform
:
rotateY
(
0
deg
);
30
}
31
.
back
{
32
background
:
#9dcc78
;
33
transform
:
rotateY
(
180
deg
);
34
}
Greeting card opening
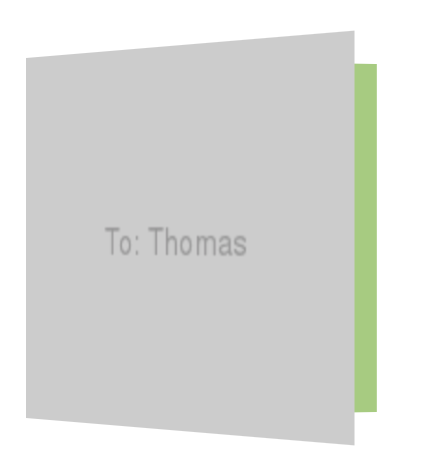
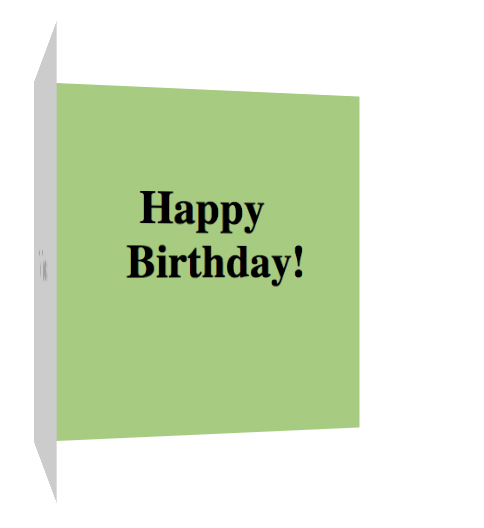
Parallax 3D Rotation
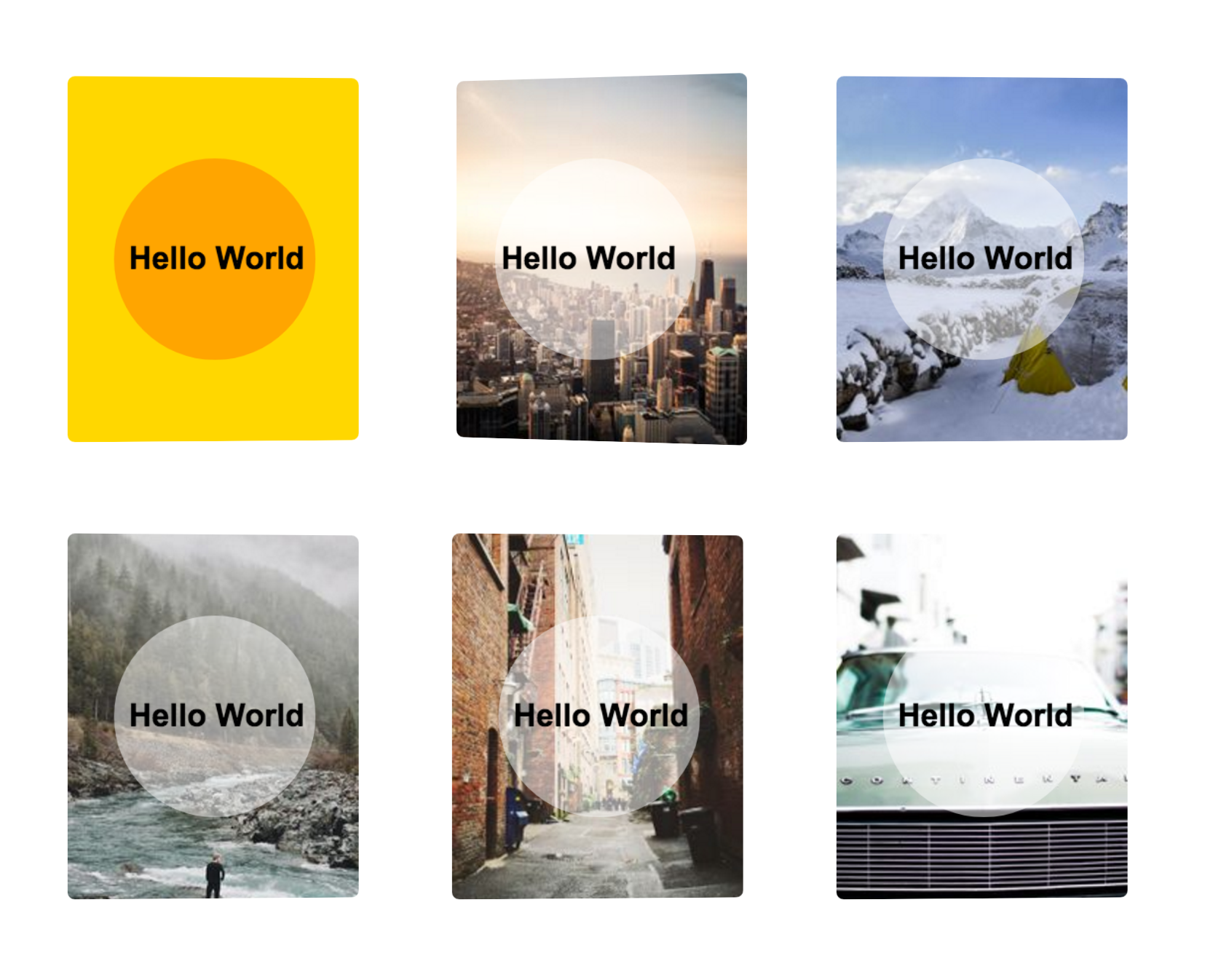
https://css3effects.com/parallax-card
Code snippets
HTML
1
<
div
class
=
"demo photo"
>
2
<
div
class
=
"card-content"
>
3
<
div
class
=
"bg-object"
></
div
>
4
<
div
class
=
"title"
>
Hello
World
</
div
>
5
</
div
>
6
</
div
>
CSS
1
.
demo
{
2
width
:
200
px
;
3
height
:
250
px
;
4
5
float
:
left
;
6
7
margin
:
2
em
;
8
9
perspective
:
300
px
;
10
}
11
.
card-content
{
12
width
:
100
%
;
13
height
:
100
%
;
14
background
:
gold
;
15
display
:
flex
;
16
align-items
:
center
;
17
justify-content
:
center
;
18
transform
:
rotateY
(
1
deg
);
19
transition
:
all
0.3
s
ease-out
;
20
21
border-radius
:
5
px
;
22
23
transform-style
:
preserve-3d
;
24
}
25
.
demo
:
hover
.
card-content
{
26
transform
:
rotateY
(
-4
deg
);
27
}
28
.
bg-object
{
29
background
:
orange
;
30
width
:
120
px
;
31
height
:
120
px
;
32
position
:
absolute
;
33
border-radius
:
50
%
;
34
transform
:
translateZ
(
40
px
);
35
}
36
37
.
title
{
38
transform
:
translateZ
(
80
px
);
39
font-weight
:
bold
;
40
}
41
42
/* Photo Card */
43
.
demo
.
photo
img
{
44
position
:
absolute
;
45
width
:
100
%
;
46
height
:
100
%
;
47
object-fit
:
cover
;
48
border-radius
:
5
px
;
49
}
50
.
demo
.
photo
.
bg-object
{
51
background
:
rgba
(
255
,
255
,
255
,
.5
);
52
}
How it works
The key to create parallax effect:
- a container
- the element to rotate, with preserve-3d
- the children elements with translate-z
to remember the coordinate, I use left hand like this.
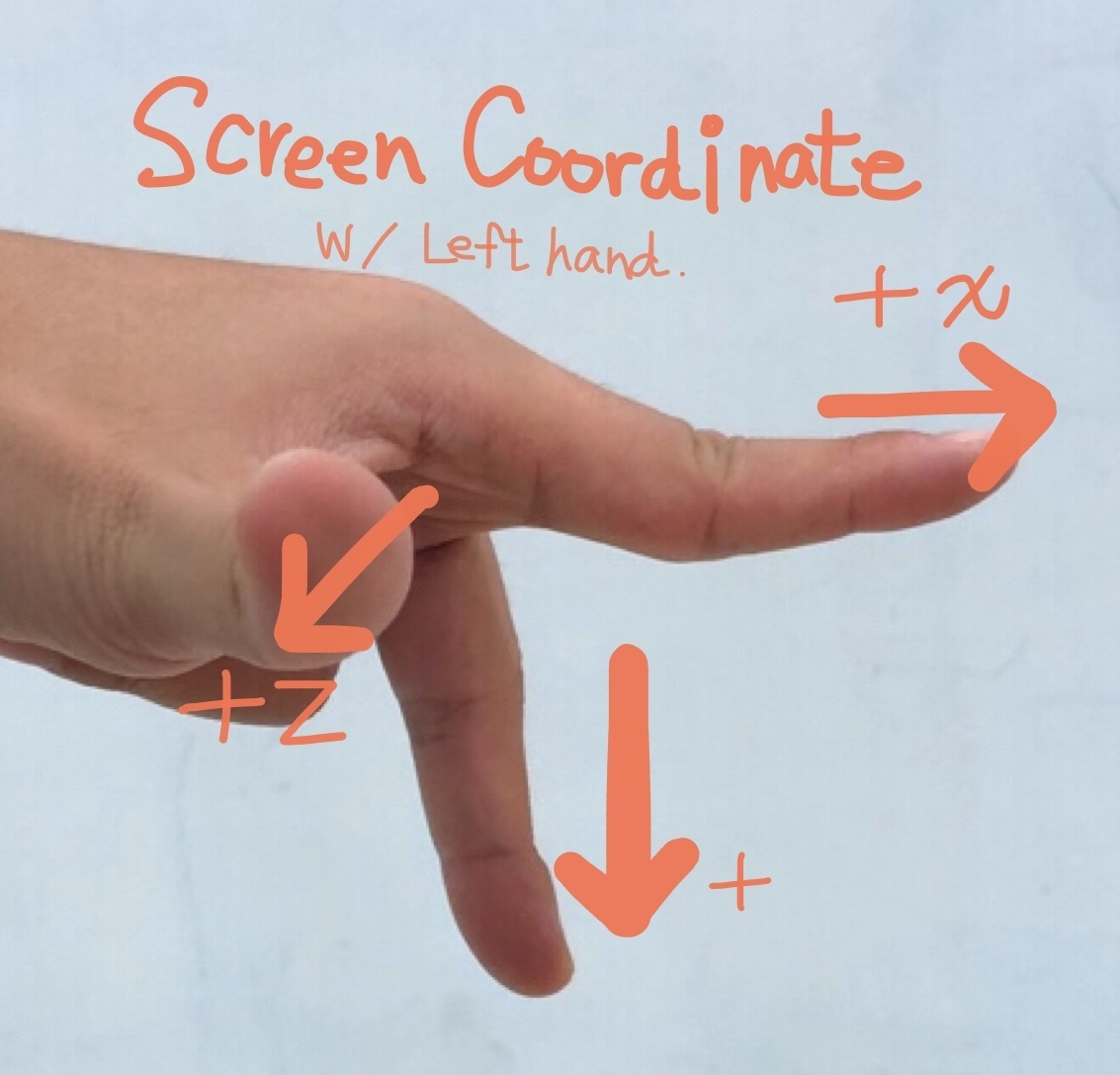
- The index finger is x-axis.
- The middle finger is y-axis.
- The thumb is z-index, pointing out of the screen.
And they all points to the positive direction.
Cube Rotation
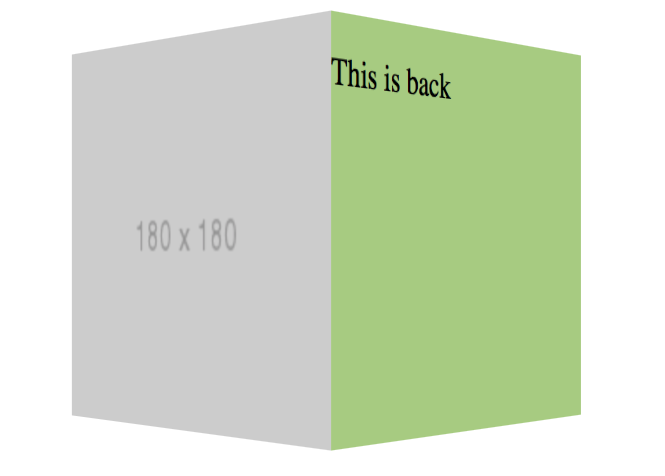
https://css3effects.com/cube
Code snippets
HTML
1
<
div
class
=
'box-scene'
>
2
<
div
class
=
'box'
>
3
<
div
class
=
'front face'
>
4
<
img
src
=
'http://placehold.it/180x180/'
alt
=
''
>
5
</
div
>
6
<
div
class
=
"side face"
>
7
<
p
>
This
is
back
</
p
>
8
</
div
>
9
</
div
>
10
</
div
>
CSS
1
.
box-scene
{
2
perspective
:
700
px
;
3
width
:
400
px
;
4
height
:
200
px
;
5
margin
:
auto
;
6
7
z-index
:
999
;
8
}
9
.
box-scene
:
hover
.
box
{
10
transform
:
rotateY
(
-90
deg
);
11
}
12
.
box
{
13
width
:
180
px
;
14
height
:
180
px
;
15
position
:
relative
;
16
17
transform-style
:
preserve-3d
;
18
19
transition
:
all
0.4
s
ease-out
;
20
transform-origin
:
90
px
90
px
-90
px
;
21
22
/* float: left; */
23
margin
:
30
px
auto
;
24
25
26
}
27
28
.
face
{
29
position
:
absolute
;
30
width
:
100
%
;
31
height
:
100
%
;
32
backface-visibility
:
visible
;
33
34
transform-origin
:
0
0
;
35
}
36
.
front
{
37
transform
:
rotateY
(
0
deg
);
38
z-index
:
2
;
39
background
:
#d9d9d9
;
40
}
41
.
side
{
42
background
:
#9dcc78
;
43
transform
:
rotateY
(
90
deg
);
44
z-index
:
1
;
45
left
:
180
px
;
46
}
How it works
The preserve 3d setting
3D Book Cover
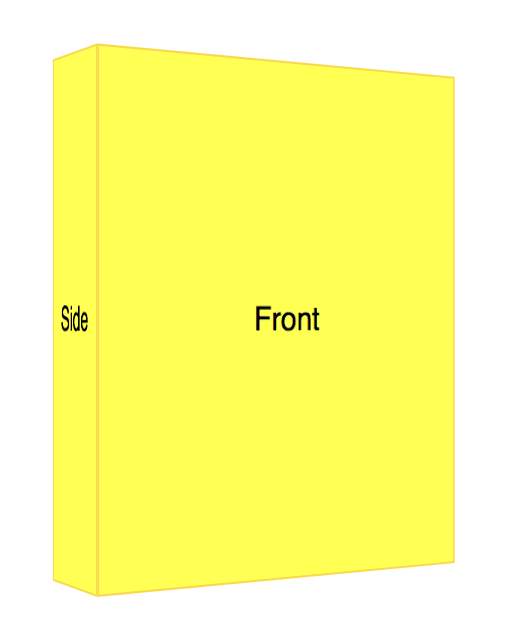
https://css3effects/book-cover
HTML
1
<
div
class
=
"scene"
>
2
<
div
class
=
"cube"
>
3
<
div
class
=
"front face"
>
Front
</
div
>
4
<
div
class
=
"side face"
>
Side
</
div
>
5
<
div
class
=
"back face"
>
Back
</
div
>
6
</
div
>
7
</
div
>
CSS
1
.
scene
{
2
perspective
:
800
px
;
3
width
:
200
px
;
4
height
:
250
px
;
5
}
6
.
cube
{
7
width
:
200
px
;
8
height
:
250
px
;
9
position
:
relative
;
10
11
transform
:
rotate3d
(
0
,
1
,
0
,
0
deg
);
12
transform-style
:
preserve-3d
;
13
transform-origin
:
50
%
50
%
-25
px
;
14
15
transition
:
all
0.3
s
ease-out
;
16
}
17
.
scene
:
hover
.
cube
{
18
transform
:
rotate3d
(
0
,
1
,
0
,
30
deg
);
19
}
20
.
scene
:
active
.
cube
{
21
transform
:
rotate3d
(
0
,
1
,
0
,
180
deg
);
22
transition-duration
:
0.4
s
;
23
}
24
.
face
{
25
border
:
1
px
solid
GOLD
;
26
background-color
:
rgba
(
255
,
255
,
0
,
1
);
27
backface-visibility
:
hidden
;
28
width
:
100
%
;
29
height
:
100
%
;
30
position
:
absolute
;
31
top
:
0
;
32
left
:
0
;
33
text-align
:
center
;
34
line-height
:
250
px
;
35
}
36
.
front
.
face
{
37
z-index
:
0
;
38
}
39
.
side
.
face
{
40
transform
:
translate3d
(
-100
%
,
0
,
0
)
rotate3d
(
0
,
1
,
0
,
-90
deg
);
41
transform-origin
:
100
%
50
%
;
42
z-index
:
0
;
43
width
:
50
px
;
44
}
45
.
back
.
face
{
46
transform
:
translate3d
(
0
,
0
,
-50
px
)
rotate3d
(
0
,
1
,
0
,
180
deg
);
47
}
Constructing 3D Scene
Code snippets
How it works
Parallax Scene
Code snippets
How it works
https://codepen.io/makzan/pen/NxMyLM
Chapter 6—Animation
Transition with transform
http://codepen.io/makzan/pen/xOpoWd
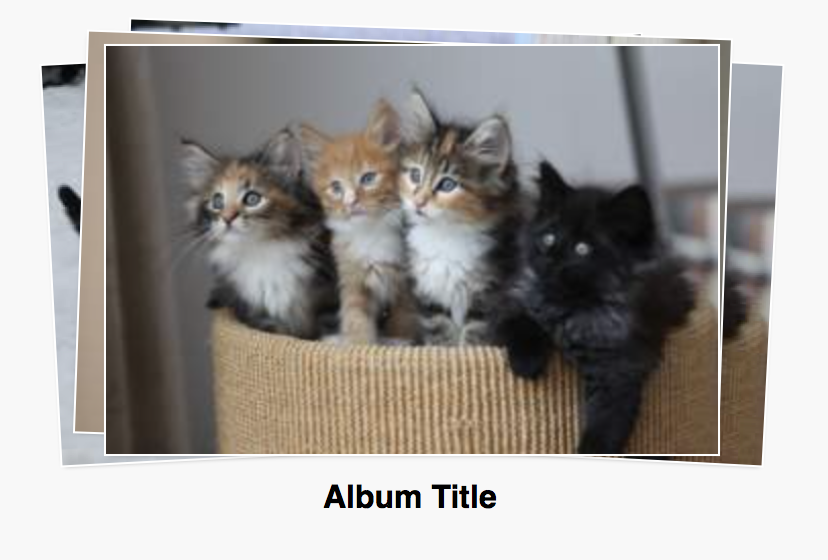
Code snippets
HTML
1
<
div
class
=
'photo-stack'
>
2
<
img
src
=
'http://placekitten.com/300/200'
>
3
<
img
src
=
'http://placekitten.com/300/200?image=2'
>
4
<
img
src
=
'http://placekitten.com/300/200?image=3'
>
5
<
img
src
=
'http://placekitten.com/300/200?image=4'
>
6
<
img
src
=
'http://placekitten.com/300/200?image=5'
>
7
<
p
>
Album
Title
</
p
>
8
</
div
>
CSS
1
.
photo-stack
{
2
position
:
relative
;
3
margin
:
auto
;
4
width
:
300
px
;
5
height
:
250
px
;
6
}
7
.
photo-stack
>
p
{
8
position
:
absolute
;
9
width
:
100
%
;
10
bottom
:
0
;
11
left
:
0
;
12
text-align
:
center
;
13
font-weight
:
bold
;
14
z-index
:
1000
;
15
}
16
.
photo-stack
img
{
17
position
:
absolute
;
18
top
:
0
;
19
left
:
0
;
20
border
:
1
px
solid
white
;
21
box-shadow
:
0
1
px
3
px
-2
px
rgba
(
0
,
0
,
0
,
.5
);
22
transition
:
all
0.3
s
ease-out
;
23
}
24
.
photo-stack
img
:
nth-child
(
1
)
{
25
z-index
:
999
;
26
}
27
.
photo-stack
img
:
nth-child
(
2
)
{
28
transform
:
rotate3d
(
0
,
0
,
1
,
3
deg
);
29
}
30
.
photo-stack
img
:
nth-child
(
3
)
{
31
transform
:
rotate3d
(
0
,
0
,
1
,
-3
deg
);
32
}
33
.
photo-stack
img
:
nth-child
(
4
)
{
34
transform
:
rotate3d
(
0
,
0
,
1
,
2
deg
);
35
}
36
37
.
photo-stack
:
hover
img
:
nth-child
(
1
)
{
38
transform
:
scale
(
1.02
);
39
}
40
.
photo-stack
:
hover
img
:
nth-child
(
2
)
{
41
transform
:
translate3d
(
10
%
,
0
,
0
)
rotate3d
(
0
,
0
,
1
,
3
deg
);
42
}
43
.
photo-stack
:
hover
img
:
nth-child
(
3
)
{
44
transform
:
translate3d
(
-10
%
,
0
,
0
)
rotate3d
(
0
,
0
,
1
,
-3
deg
);
45
}
46
.
photo-stack
:
hover
img
:
nth-child
(
4
)
{
47
transform
:
translate3d
(
2
%
,
-5
%
,
0
)
rotate3d
(
0
,
0
,
1
,
2
deg
);
48
}
49
.
photo-stack
:
hover
img
:
nth-child
(
5
)
{
50
transform
:
translate3d
(
-5
%
,
-2
%
,
0
)
rotate3d
(
0
,
0
,
1
,
2
deg
);
51
}
Key frame animation
Code snippets
How it works
Pause in between animation
Code snippets
How it works
Sprite sheet animation
Code snippets
How it works
Circular motion lines
We can use the techniques from Pie drawing to create circular motion lines.
At the end of the section, we will have the following effect created.
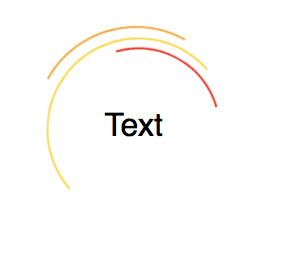
Code snippet
1
.
demo
{
2
border-radius
:
50
%
;
3
border
:
1
px
solid
transparent
;
4
border-top-color
:
orange
;
5
width
:
100
px
;
6
height
:
100
px
;
7
}
How it works
Let’s animate the motion line
1
@
keyframes
keep-rotating
{
2
0
%
{
transform
:
rotateZ
(
0
)}
3
100
%
{
transform
:
rotateZ
(
360
deg
)}
4
}
5
.
demo
{
6
border-radius
:
50
%
;
7
border
:
1
px
solid
transparent
;
8
border-top-color
:
orange
;
9
width
:
100
px
;
10
height
:
100
px
;
11
animation
:
1000
ms
keep-rotating
infinite
;
12
}
We can put 2 more lines there by using pseudo-elements.
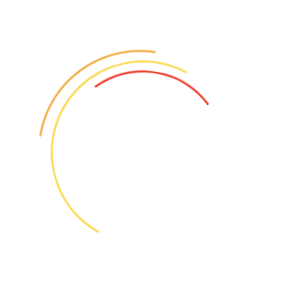
Let’s animate them all
1
@
keyframes
keep-rotating
{
2
0
%
{
transform
:
rotateZ
(
0
)}
3
100
%
{
transform
:
rotateZ
(
360
deg
)}
4
}
5
.
demo
{
6
border-radius
:
50
%
;
7
border
:
1
px
solid
transparent
;
8
border-top-color
:
orange
;
9
width
:
100
px
;
10
height
:
100
px
;
11
animation
:
1000
ms
keep-rotating
infinite
;
12
animation-timing-function
:
linear
;
13
position
:
relative
;
14
}
15
.
demo
:
after
{
16
content
:
''
;
17
position
:
absolute
;
18
width
:
80
%
;
19
height
:
80
%
;
20
left
:
calc
(
50
%
-
80
%
/
2
);
21
top
:
calc
(
50
%
-
80
%
/
2
);
22
border
:
1
px
solid
transparent
;
23
border-top-color
:
red
;
24
border-radius
:
50
%
;
25
transform
:
rotateZ
(
45
deg
)
26
}
27
.
demo
:
before
{
28
content
:
''
;
29
position
:
absolute
;
30
width
:
90
%
;
31
height
:
90
%
;
32
left
:
calc
(
50
%
-
90
%
/
2
);
33
top
:
calc
(
50
%
-
90
%
/
2
);
34
border
:
1
px
solid
transparent
;
35
border-top-color
:
gold
;
36
border-left-color
:
gold
;
37
border-radius
:
50
%
;
38
transform
:
rotateZ
(
20
deg
)
39
}
Wrapper with text
The following won’t work:
1
<
div
class
=
"animated lines demo"
>
Text
</
div
>
If we want to put a text at the center of the motion lines, you’ll find the text rotate with the lines as well.
That’s because we are rotating the .demo
element. The text is inside the .demo
element.
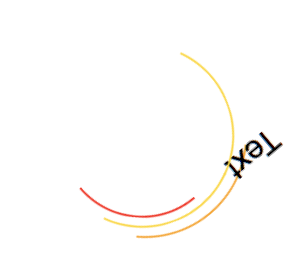
1
<
div
class
=
"demo-container"
>
2
<
div
class
=
"animated lines demo"
></
div
>
3
<
p
>
Text
</
p
>
4
</
div
>
Extra CSS for positioning:
1
.
demo-container
{
2
width
:
100
px
;
3
height
:
100
px
;
4
position
:
relative
;
5
display
:
flex
;
6
}
7
.
demo-container
.
demo
{
8
position
:
absolute
;
9
top
:
0
;
10
left
:
0
;
11
width
:
100
%
;
12
height
:
100
%
;
13
}
14
.
demo-container
p
{
15
margin
:
auto
;
16
}
One element solution
1
<
div
class
=
"text-with-motion-line two-lines demo2"
>
Text
</
div
>
The CSS that use pseudo-element to create the motion lines, instead of rotating the element itself.
1
.
demo2
{
2
display
:
flex
;
3
align-items
:
center
;
4
justify-content
:
center
;
5
border
:
none
;
6
7
width
:
100
px
;
8
height
:
100
px
;
9
position
:
relative
;
10
}
11
12
.
text-with-motion-line
.
two-lines
:
after
,
13
.
text-with-motion-line
:
before
{
14
content
:
''
;
15
width
:
100
%
;
16
height
:
100
%
;
17
position
:
absolute
;
18
top
:
0
;
19
left
:
0
;
20
border-radius
:
50
%
;
21
border
:
1
px
solid
transparent
;
22
border-top-color
:
orange
;
23
24
animation
:
1000
ms
keep-rotating
infinite
;
25
animation-timing-function
:
linear
;
26
}
27
.
text-with-motion-line
.
two-lines
:
after
{
28
border-top-color
:
gold
;
29
width
:
90
%
;
30
height
:
90
%
;
31
left
:
calc
(
50
%
-
90
%
/
2
);
32
top
:
calc
(
50
%
-
90
%
/
2
);
33
animation-delay
:
0.15
s
;
34
}
From the example, you can find that we can have variants on the same effect. Sometimes we can create the effect by using one elements and its pseudo elements. Sometimes we may need extra elements and wrapper to achieve the result. Either way, we want to achieve the result effect and make it as elegant as possible, but if we can’t make it elegant, that’s okay to move on with a result with not-so-good HTML structure.
Password input transition
Code snippets
How it works
Circular motions
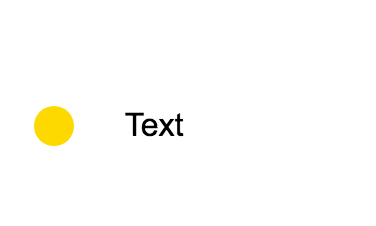
https://codepen.io/makzan/pen/PeWJdq
Code snippets
1
<
div
class
=
"demo debug"
>
2
<
div
class
=
"orbit"
></
div
>
3
<
span
>
Text
</
span
>
4
</
div
>
CSS
1
.
demo
{
2
width
:
100
px
;
3
height
:
100
px
;
4
position
:
relative
;
5
display
:
flex
;
6
align-items
:
center
;
7
justify-content
:
center
;
8
}
9
10
.
demo
.
orbit
{
11
width
:
100
%
;
12
height
:
100
%
;
13
transition
:
all
.3
s
ease-out
;
14
position
:
absolute
;
15
top
:
0
;
16
left
:
0
;
17
border-radius
:
50
%
;
18
}
19
20
.
demo
.
orbit
:
after
{
21
--
size
:
20
%
;
22
content
:
''
;
23
position
:
absolute
;
24
width
:
var
(
--
size
);
25
height
:
var
(
--
size
);
26
top
:
calc
(
50
%
-
var
(
--
size
)
/
2
);
27
left
:
calc
(
-1
*
var
(
--
size
)
/
2
);
28
border-radius
:
50
%
;
29
background
:
gold
;
30
}
31
32
.
demo
:
hover
.
orbit
{
33
transform
:
rotateZ
(
540
deg
);
34
}
How it works
Circular loading animation
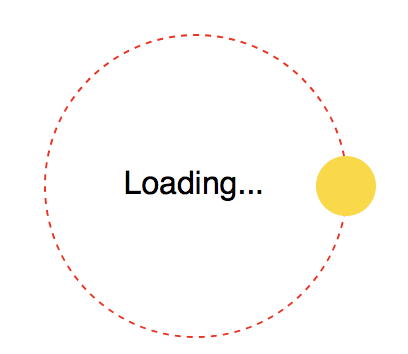
https://css3effects.com/circular-loading
HTML
1
<
div
class
=
"demo debug"
>
2
<
div
class
=
"orbit"
></
div
>
3
<
span
>
Loading
...</
span
>
4
</
div
>
CSS
1
.
demo
{
2
width
:
150
px
;
3
height
:
150
px
;
4
position
:
relative
;
5
display
:
flex
;
6
align-items
:
center
;
7
justify-content
:
center
;
8
}
9
10
.
demo
.
orbit
{
11
width
:
100
%
;
12
height
:
100
%
;
13
transition
:
all
.3
s
ease-out
;
14
position
:
absolute
;
15
top
:
0
;
16
left
:
0
;
17
border-radius
:
50
%
;
18
}
19
20
.
demo
.
orbit
:
after
{
21
--
size
:
20
%
;
22
content
:
''
;
23
position
:
absolute
;
24
width
:
var
(
--
size
);
25
height
:
var
(
--
size
);
26
top
:
calc
(
50
%
-
var
(
--
size
)
/
2
);
27
left
:
calc
(
-1
*
var
(
--
size
)
/
2
);
28
border-radius
:
50
%
;
29
background
:
gold
;
30
}
31
32
.
demo
:
hover
.
orbit
{
33
transform
:
rotateZ
(
540
deg
);
34
}
35
36
/* Debug Style */
37
.
debug
.
demo
.
orbit
{
38
border
:
1
px
dashed
red
;
39
}
Summary
In this book, we created different visual effects on web browser by using CSS3 techniques.
Thank you
Thanks for reading this book. Enjoy the CSS3 visual effects.
Detailed Version History
- 2018-02-20: Version 0.1.0—Initialize the book
- 2018-04-28: Version 0.1.1—Confirmed 80% of the sections headings. Writing code examples in Codepen collection: https://codepen.io/collection/DVpWNq/
- 2018-05-20: Version 0.1.2—Setup book domain css3effects.com.