Table of Contents
Introduction
Result
objects represent the outcome of an operation, removing the need to check for null. Operations that succeed produce results encapsulating a success value; operations that fail produce results with a failure value. Success and failure can be represented by whatever types make the most sense for each operation.
Results in a Nutshell
In Java, methods that can fail typically do so by throwing exceptions. Then, exception-throwing methods are called from inside a try
block to handle errors in a separate catch
block.
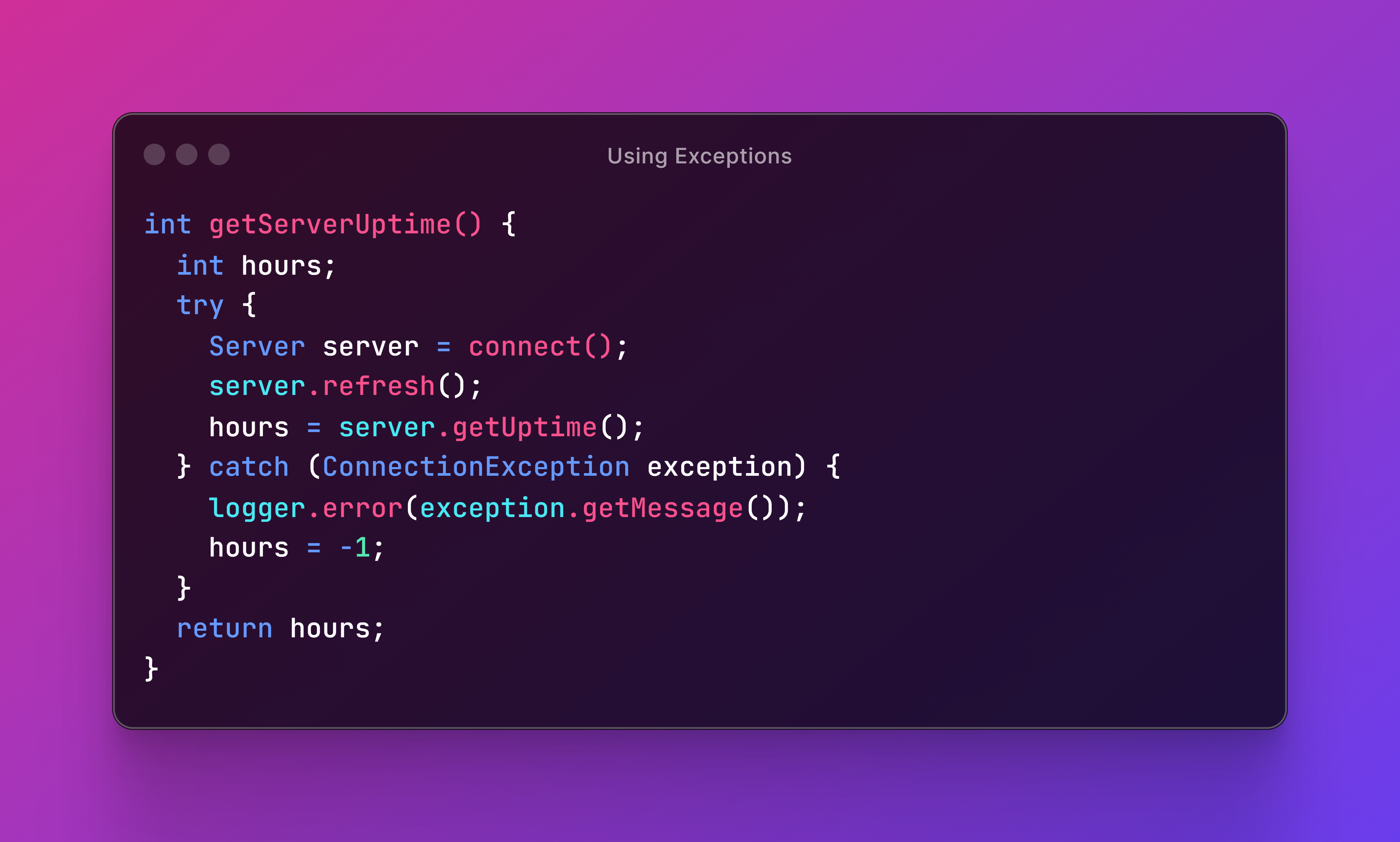
This approach is lengthy, and that’s not the only problem — it’s also very slow.
![]() |
Conventional wisdom says exceptional logic shouldn’t be used for normal program flow. Results make us deal with expected error situations explicitly to enforce good practices and make our programs run faster. |
Let’s now look at how the above code could be refactored if connect()
returned a Result
object instead of throwing an exception.
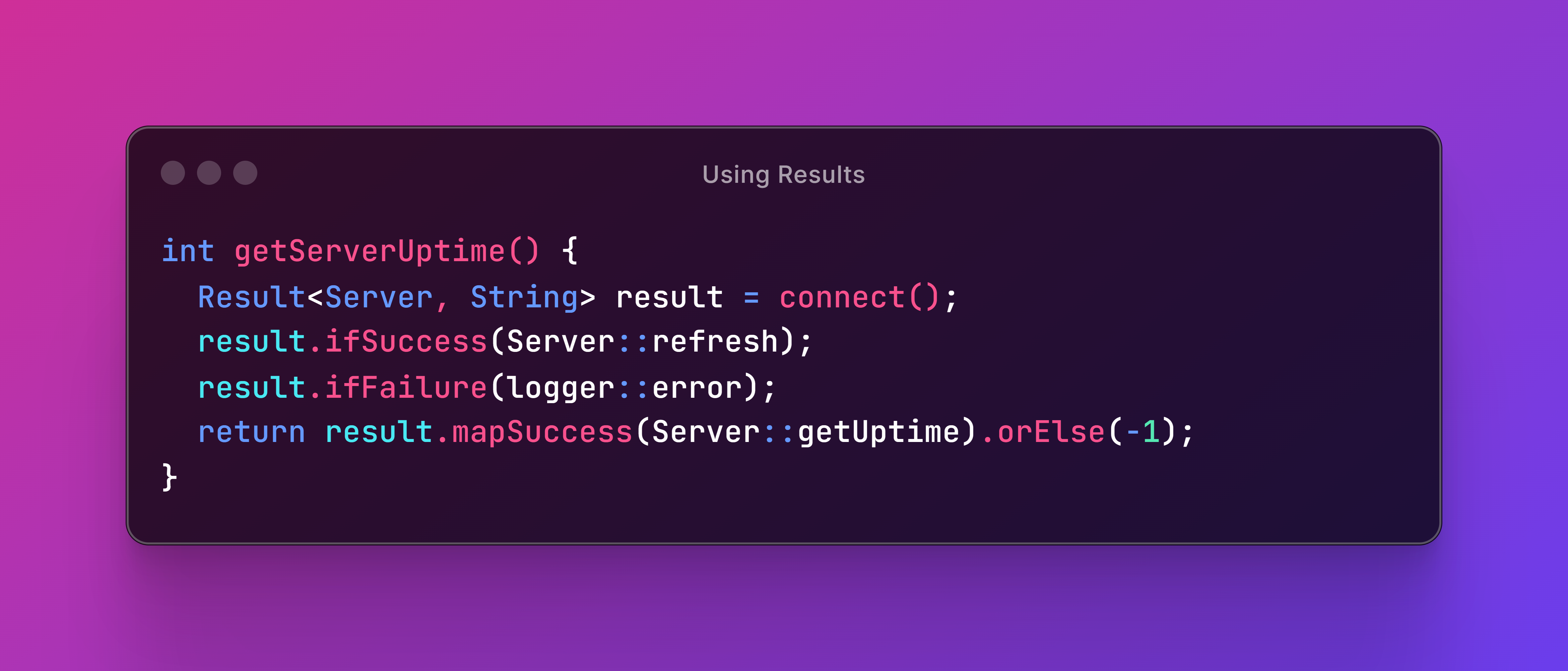
In the example above, we used only 4 lines of code to replace the 10 that worked for the first one. But we can effortlessly make it shorter by chaining methods. In fact, since we were returning -1
just to signal that the underlying operation failed, we are better off returning a Result
object upstream. This will allow us to compose operations on top of getServerUptime()
just like we did with connect()
.
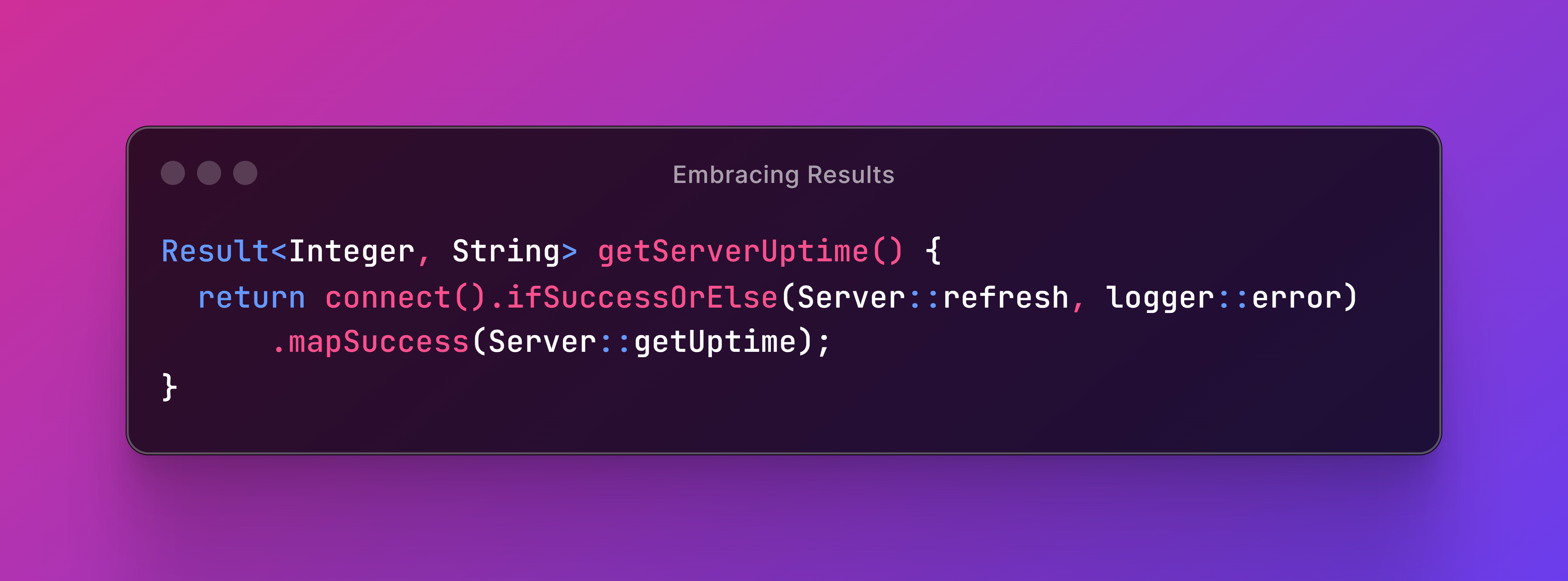
Result
objects are immutable, providing thread safety without the need for synchronization. This makes them ideal for multi-threaded applications, ensuring predictability and eliminating side effects.
Getting Started
How to get up and running with Results in no time
The best way to think of Results is as a super-powered version of Java’s Optionals.
Result
builds upon the familiar concept of Optional
, enhancing it with the ability to represent both success and failure states.
Result API
Results provide the same methods as Optionals, plus additional ones to handle failure states effectively.
Optional |
Result |
---|---|
isPresent |
hasSuccess |
isEmpty |
hasFailure |
get |
getSuccess |
getFailure |
|
orElse |
orElse |
orElseGet |
orElseMap |
stream |
streamSuccess |
streamFailure |
|
ifPresent |
ifSuccess |
ifFailure |
|
ifPresentOrElse |
ifSuccessOrElse |
filter |
filter |
recover |
|
map |
mapSuccess |
mapFailure |
|
map |
|
flatMap |
flatMapSuccess |
or |
flatMapFailure |
flatMap |
Why Results over Optionals?
Optional
class is useful for representing values that might be present or absent, eliminating the need for null checks. However, Optionals fall short when it comes to error handling because they do not convey why a value is lacking. Result
addresses this limitation by encapsulating both successful values and failure reasons, offering a more expressive way to reason about what went wrong.
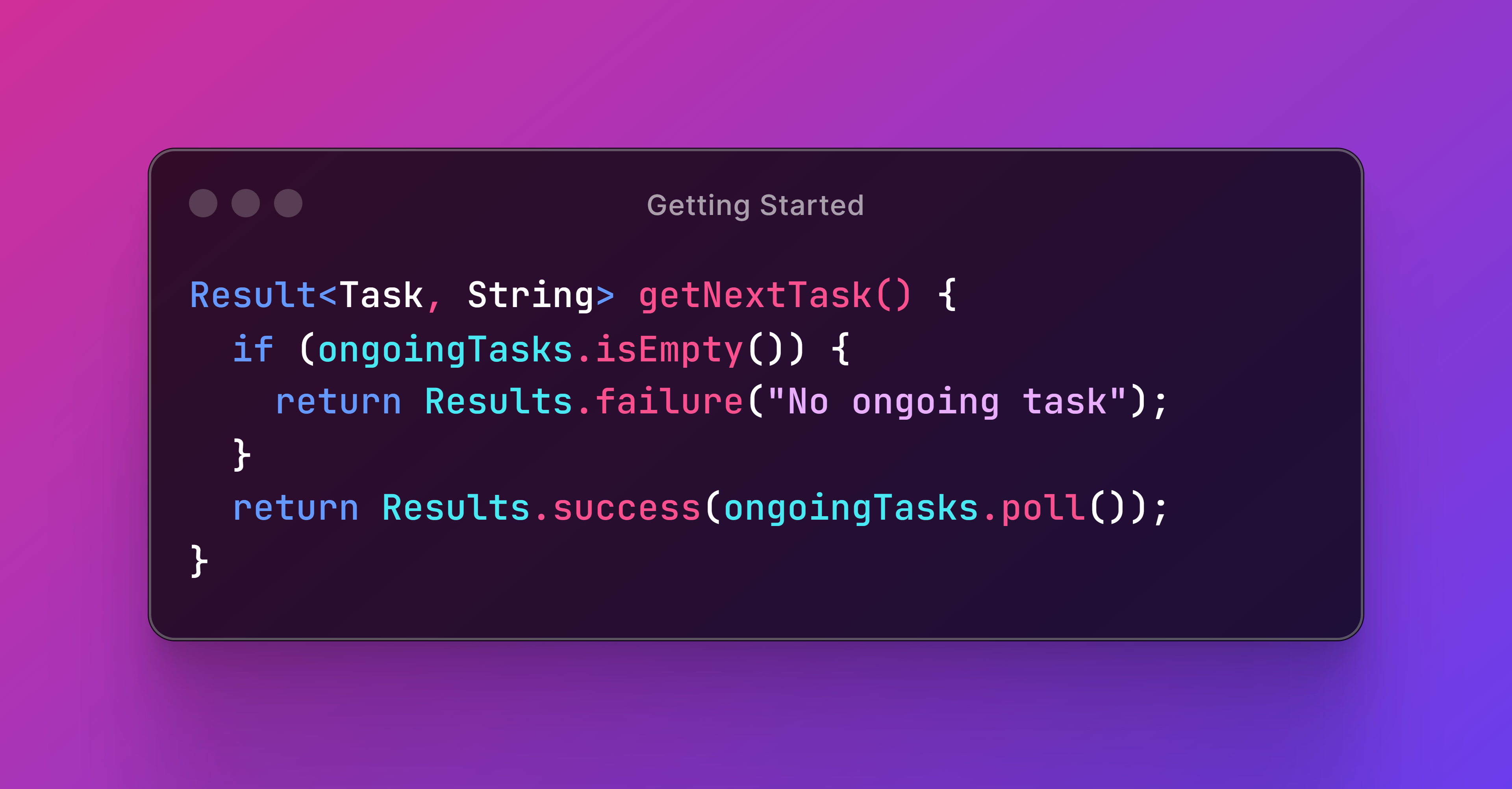
By leveraging Results, you can unleash a powerful tool for error handling that goes beyond the capabilities of traditional Optionals, leading to more robust and maintainable Java code.
Adding Result to Your Build
How to add Result as a dependency to your build
This library adheres to Pragmatic Versioning to communicate the backwards compatibility of each version.
The latest releases are available in Maven Central.
Artifact Coordinates
Add this Maven dependency to your build:
Group ID | Artifact ID | Version |
---|---|---|
com.leakyabstractions |
result |
1.0.0.0 |
Maven Central provides snippets for different build tools to declare this dependency.
Maven
To use Result
, we can add a Maven dependency to our project.
<dependencies>
<dependency>
<groupId>com.leakyabstractions</groupId>
<artifactId>result</artifactId>
<version>1.0.0.0</version>
</dependency>
</dependencies>
Gradle
We can also add Result
as a Gradle dependency.
dependencies {
implementation("com.leakyabstractions:result:1.0.0.0")
}
This is the most common configuration for projects using Result
internally. If we were building a library that exposed Result
in its public API, we should use api
instead of implementation
.
Conclusion
We learned how to add the library to your project using either Maven or Gradle. By including the correct dependencies, you’re now ready to start leveraging the power of Results in your applications.
Creating Results
How to instantiate new Result objects
There are several ways to create result objects.
Successful Results
A successful result contains a non-null value produced by an operation when everything works as intended. We can use Results.success
to create a new instance.
1 @Test
2 void testSuccess() {
3 // When
4 Result<Integer, ?> result = Results.success(200);
5 // Then
6 assertTrue(result::hasSuccess);
7 assertFalse(result::hasFailure);
8 }
Note that we can invoke hasSuccess
or hasFailure
to check whether a result is successful or failed (more on this in the next chapter).
Failed Results
On the other hand, a failed result holds a value representing the problem that prevented the operation from completing. We can use Results.failure
to create a new one.
1 @Test
2 void testFailure() {
3 // When
4 Result<?, String> result = Results.failure("The operation failed");
5 // Then
6 assertTrue(result::hasFailure);
7 assertFalse(result::hasSuccess);
8 }
Failure values cannot be null
either.
Results Based on Nullable Values
When we need to create results that depend on a possibly null value, we can use Results.ofNullable
. If the first argument is null
then the second one will be used to create a failed result.
1 @Test
2 void testOfNullable() {
3 // Given
4 String string1 = "The operation succeeded";
5 String string2 = null;
6 // When
7 Result<String, Integer> result1 = Results.ofNullable(string1, 404);
8 Result<String, Integer> result2 = Results.ofNullable(string2, 404);
9 // Then
10 assertTrue(result1::hasSuccess);
11 assertTrue(result2::hasFailure);
12 }
The second argument can be either a failure value or a function that produces a failure value.
Results Based on Optionals
We can also use Results.ofOptional
to create results that depend on an Optional
value. If the first argument is an empty optional, then the second one will be used to create a failed result.
1 @Test
2 void testOfOptional() {
3 // Given
4 Optional<BigDecimal> optional1 = Optional.of(BigDecimal.ONE);
5 Optional<BigDecimal> optional2 = Optional.empty();
6 // When
7 Result<BigDecimal, Integer> result1 = Results.ofOptional(optional1, -1);
8 Result<BigDecimal, Integer> result2 = Results.ofOptional(optional2, -1);
9 // Then
10 assertTrue(result1::hasSuccess);
11 assertTrue(result2::hasFailure);
12 }
The second argument can be a Supplier
too.
Results Based on Callables
Finally, if we have a task that may either return a success value or throw an exception, we can encapsulate it as a result using Results.ofCallable
so we don’t need to use a try-catch block.
1 String task1() {
2 return "OK";
3 }
4
5 String task2() throws Exception {
6 throw new Exception("Whoops!");
7 }
8
9 @Test
10 void testOfCallable() {
11 // When
12 Result<String, Exception> result1 = Results.ofCallable(this::task1);
13 Result<String, Exception> result2 = Results.ofCallable(this::task2);
14 // Then
15 assertTrue(result1::hasSuccess);
16 assertTrue(result2::hasFailure);
17 }
This method enables compatibility with legacy or third-party code that uses exceptions to indicate operation failure.
Conclusion
We’ve covered how to create new instances of Result
using various factory methods provided by the Results
class. Each method serves a specific purpose, allowing you to select the most suitable one based on the situation.
Basic Usage
How to solve simple use-case scenarios
In this section, we’ll cover foundational use cases, including checking the status of a result, unwrapping the value inside a result, and taking different actions based on success or failure.
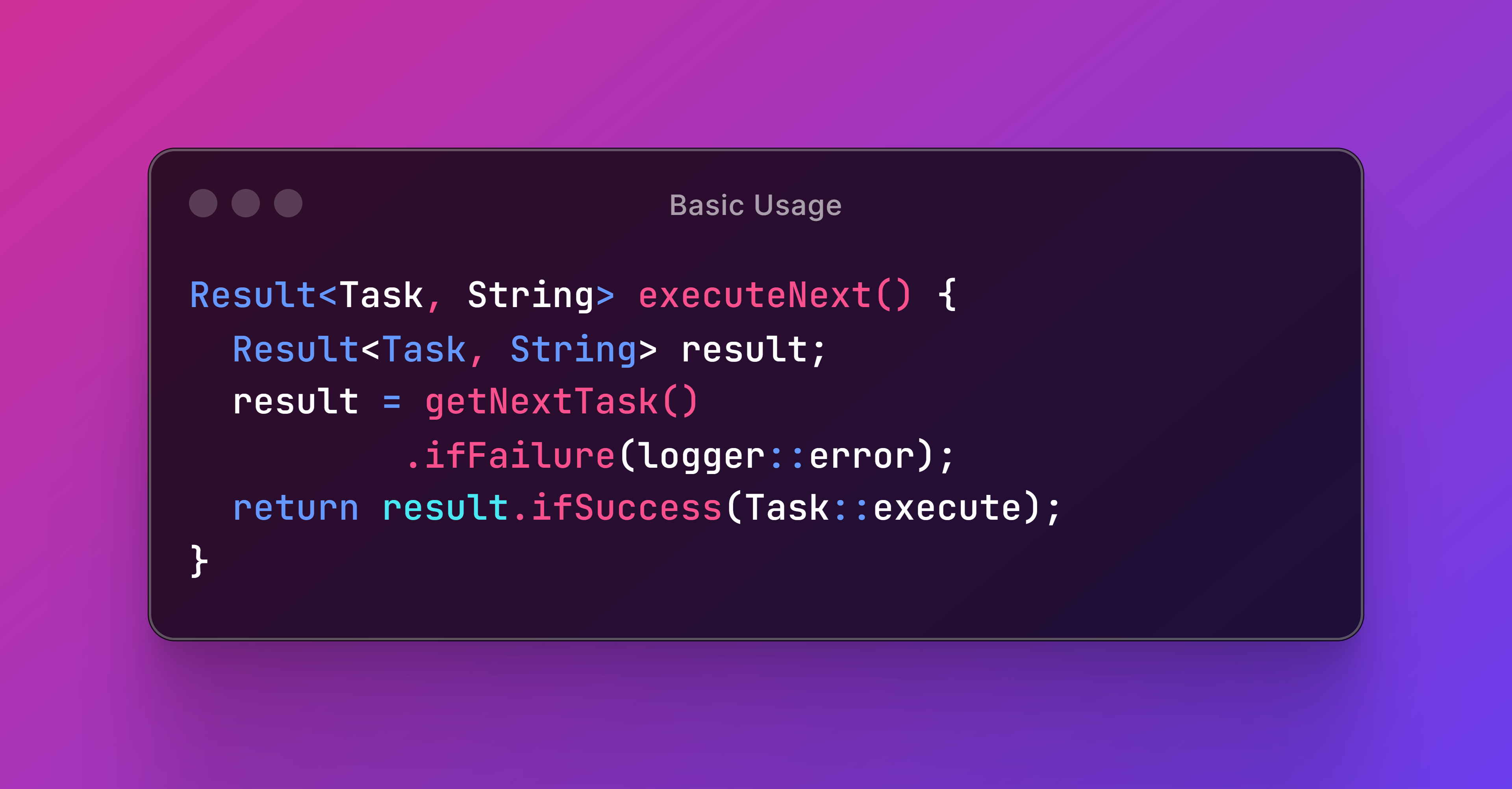
These basics will help you handle errors more cleanly and efficiently without cluttering your code with try-catch blocks.
Checking Success or Failure
How to find out if the operation succeded or failed
As we discovered earlier, we can easily determine if a given Result
instance is successful or not.
Checking Success
We can use hasSuccess
to obtain a boolean
value that represents whether a result is successful.
1 @Test
2 void testHasSuccess() {
3 // When
4 boolean result1HasSuccess = success(1024).hasSuccess();
5 boolean result2HasSuccess = failure(1024).hasSuccess();
6 // Then
7 assertTrue(result1HasSuccess);
8 assertFalse(result2HasSuccess);
9 }
Checking Failure
We can also use hasFailure
to find out if a result contains a failure value.
1 @Test
2 void testHasFailure() {
3 // When
4 boolean result1HasFailure = success(512).hasFailure();
5 boolean result2HasFailure = failure(512).hasFailure();
6 // Then
7 assertFalse(result1HasFailure);
8 assertTrue(result2HasFailure);
9 }
Conclusion
We discussed how to determine the state of a Result object using hasSuccess
and hasFailure
. These methods provide a straightforward way to identify the outcome of an operation, helping you make decisions based on the outcome.
Unwrapping Values
How to get values out of Result objects
In essence, a Result
object is just a container that wraps a success or a failure value for us. Therefore, sometimes you are going to want to get that value out of the container.
As useful as this may seem, we will soon realize that we won’t be doing it very often.
Unwrapping Success
The most basic way to retrieve the success value wrapped inside a result is by using getSuccess
. This method will return an optional success value, depending on whether the result was actually successful or not.
1 @Test
2 void testGetSuccess() {
3 // Given
4 Result<?, ?> result1 = success("SUCCESS");
5 Result<?, ?> result2 = failure("FAILURE");
6 // Then
7 Optional<?> success1 = result1.getSuccess();
8 Optional<?> success2 = result2.getSuccess();
9 // Then
10 assertEquals("SUCCESS", success1.get());
11 assertTrue(success2::isEmpty);
12 }
Unwrapping Failure
Similarly, we can use getFailure
to obtain the failure value held by a Result
object.
1 @Test
2 void testGetFailure() {
3 // Given
4 Result<?, ?> result1 = success("SUCCESS");
5 Result<?, ?> result2 = failure("FAILURE");
6 // Then
7 Optional<?> failure1 = result1.getFailure();
8 Optional<?> failure2 = result2.getFailure();
9 // Then
10 assertTrue(failure1::isEmpty);
11 assertEquals("FAILURE", failure2.get());
12 }
Unlike Optional’s get
, these methods are null-safe. However, in practice, we will not be using them frequently. Especially, since there are more convenient ways to get the success value out of a result.
Using Alternative Success
We can use orElse
to provide an alternative success value that must be returned when the result is unsuccessful.
1 @Test
2 void testGetOrElse() {
3 // Given
4 Result<String, String> result1 = success("IDEAL");
5 Result<String, String> result2 = failure("ERROR");
6 String alternative = "OTHER";
7 // When
8 String value1 = result1.orElse(alternative);
9 String value2 = result2.orElse(alternative);
10 // Then
11 assertEquals("IDEAL", value1);
12 assertEquals("OTHER", value2);
13 }
Note that alternative success values can be null
.
Mapping Failure
The orElseMap
method is similar to Optional’s orElseGet
, but it takes a mapping Function
instead of a Supplier
. The function will receive the failure value to produce the alternative success value.
1 @Test
2 void testGetOrElseMap() {
3 // Given
4 Result<String, Integer> result1 = success("OK");
5 Result<String, Integer> result2 = failure(1024);
6 Result<String, Integer> result3 = failure(-256);
7 Function<Integer, String> mapper = x -> x > 0 ? "HI" : "LO";
8 // When
9 String value1 = result1.orElseMap(mapper);
10 String value2 = result2.orElseMap(mapper);
11 String value3 = result3.orElseMap(mapper);
12 // Then
13 assertEquals("OK", value1);
14 assertEquals("HI", value2);
15 assertEquals("LO", value3);
16 }
Although probably not the best practice, the mapping function may return null
.
Streaming Success or Failure
Finally, we can use streamSuccess
and streamFailure
to wrap the value held by an instance of Result
into a possibly-empty Stream
object.
1 @Test
2 void testStreamSuccess() {
3 // Given
4 Result<?, ?> result1 = success("Yes");
5 Result<?, ?> result2 = failure("No");
6 // When
7 Stream<?> stream1 = result1.streamSuccess();
8 Stream<?> stream2 = result2.streamSuccess();
9 // Then
10 assertEquals("Yes", stream1.findFirst().orElse(null));
11 assertNull(stream2.findFirst().orElse(null));
12 }
13
14 @Test
15 void testStreamFailure() {
16 // Given
17 Result<?, ?> result1 = success("Yes");
18 Result<?, ?> result2 = failure("No");
19 // When
20 Stream<?> stream1 = result1.streamFailure();
21 Stream<?> stream2 = result2.streamFailure();
22 // Then
23 assertNull(stream1.findFirst().orElse(null));
24 assertEquals("No", stream2.findFirst().orElse(null));
25 }
Conclusion
We explored various ways to retrieve values from results. Using these methods you can efficiently access the underlying data within a Result object, whether it’s a success or a failure.
Conditional Actions
How to handle success and failure scenarios
We’ll now delve into a set of methods that allow you to take conditional actions based on the state of a result. They provide a cleaner and more expressive way to handle success and failure scenarios, eliminating the need for lengthy if/else blocks.
Handling Success
We can use ifSuccess
to specify an action that must be executed if the result represents a successful outcome. This method takes a consumer function that will be applied to the success value wrapped by the result.
1 @Test
2 void testIfSuccess() {
3 // Given
4 List<Object> list = new ArrayList<>();
5 Result<Integer, String> result = success(100);
6 // When
7 result.ifSuccess(list::add);
8 // Then
9 assertEquals(100, list.getFirst());
10 }
In this example, ifSuccess
ensures that the provided action (adding the success value to the list) is only executed if the parsing operation is successful.
Handling Failure
On the other hand, we can use ifFailure
method to define an action that must be taken when the result represents a failure. This method also takes a Consumer
that will be applied to the failure value inside the result.
1 @Test
2 void testIfFailure() {
3 // Given
4 List<Object> list = new ArrayList<>();
5 Result<Integer, String> result = failure("ERROR");
6 // When
7 result.ifFailure(list::add);
8 // Then
9 assertEquals("ERROR", list.getFirst());
10 }
Here, ifFailure
ensures that the provided action (adding the failure value to the list) is only executed if the parsing operation fails.
Handling Both Scenarios
Finally, ifSuccessOrElse
allows you to specify two separate actions: one for when the operation succeeded and another for when it failed. This method takes two consumer functions: the first for handling the success case and the second for handling the failure case.
1 @Test
2 void testIfSuccessOrElse() {
3 // Given
4 List<Object> list1 = new ArrayList<>();
5 List<Object> list2 = new ArrayList<>();
6 Result<Long, String> result1 = success(100L);
7 Result<Long, String> result2 = failure("ERROR");
8 // When
9 result1.ifSuccessOrElse(list1::add, list1::add);
10 result2.ifSuccessOrElse(list2::add, list2::add);
11 // Then
12 assertEquals(100L, list1.getFirst());
13 assertEquals("ERROR", list2.getFirst());
14 }
In this example, ifSuccessOrElse
simplifies conditional logic by providing a single method to handle both success and failure scenarios, making the code more concise and readable.
Conclusion
We explained how to handle success and failure scenarios using these three methods. They provide a powerful way to perform conditional actions based on the state of a Result, streamlining your error handling and making your code more readable and maintainable.
Advanced Usage
How to take Result objects to the next level
While understanding the basics provides a solid foundation, the true potential of result objects is unlocked through their functional capabilities. Mastering these techniques enables concise and readable error handling by leveraging the power of monadic composition.
![]() |
The most idiomatic approach to handling results involves screening them and applying various mapping and flat-mapping methods to transform and compose behavior. |
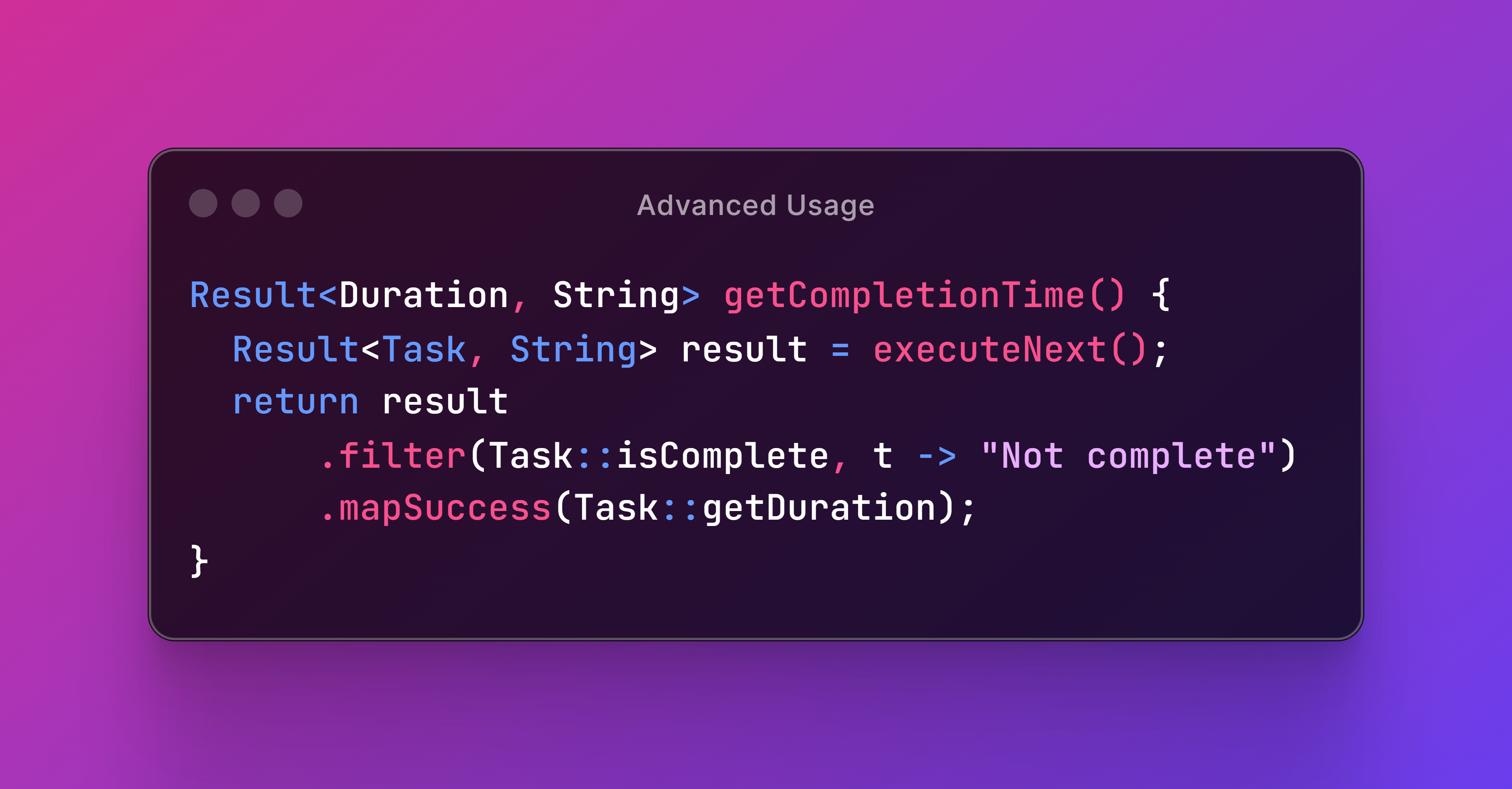
This section will guide you through these powerful tools, demonstrating how to manipulate results effectively so you can craft more robust and maintainable Java applications.
Screening Results
How to reject success values and accept failure values
Screening mechanisms provide greater flexibility in handling edge cases and enable more robust error recovery strategies.
The following methods allow you to run inline tests on the wrapped value of a result to dynamically transform a success into a failure or a failure into a success.
Validating Success
The filter
method allows you to transform a success into a failure based on certain conditions. It takes two parameters:
This can be used to enforce additional validation constraints on success values.
1 @Test
2 void testFilter() {
3 // Given
4 Result<Integer, String> result = success(1);
5 // When
6 Result<Integer, String> filtered = result.filter(x -> x % 2 == 0, x -> "It's odd");
7 // Then
8 assertTrue(filtered.hasFailure());
9 }
In this example, we use a lambda expression to validate that the success value inside result
is even. Since the number is odd, it transforms the result into a failure.
![]() |
Note that it is illegal for the mapping function to return |
Recovering From Failure
The recover
method allows you to transform a failure into a success based on certain conditions. It also receives two parameters:
- A
Predicate
to determine if the failure value is recoverable. - A mapping
Function
that will produce a success value from the acceptable failure value.
This method is useful for implementing fallback mechanisms or recovery strategies, ensuring the application logic remains resilient and adaptable.
1 @Test
2 void testRecover() {
3 // Given
4 Result<Integer, String> result = failure("OK");
5 // When
6 Result<Integer, String> filtered = result.recover("OK"::equals, String::length);
7 // Then
8 assertTrue(filtered.hasSuccess());
9 }
In this example, we use method references to check if the failure value equals OK
and then transform the result into a success.
Conclusion
We covered how to filter out unwanted success values and accept failure values using filter
and recover
. These methods enable you to refine results based on specific criteria, ensuring that only the relevant values are processed down the line.
Transforming Results
How to transform values wrapped inside Results
Transforming result objects is a key feature that enables you to compose complex operations in a clean and functional style. There are two primary techniques used for these transformations.
Mapping Results
Mapping involves applying a function to the value inside a result to produce a new result object.
Mapping Success Values
We can use mapSuccess
to apply a function to the success value of a result, transforming it into a new success value. If the result is a failure, it remains unchanged.
1 @Test
2 void testMapSuccess() {
3 // Given
4 Result<String, ?> result = success("HELLO");
5 // When
6 Result<Integer, ?> mapped = result.mapSuccess(String::length);
7 // Then
8 assertEquals(5, mapped.orElse(null));
9 }
In this example, we wrap a String
inside a Result
object and invoke mapSuccess
to calculate its length and wrap it inside a new Result
object.
Mapping Failure Values
Next up, we can use mapFailure
to apply a function to the failure value, transforming it into a new one. If the result is a success, it remains unchanged.
1 @Test
2 void testMapFailure() {
3 // Given
4 Result<?, BigDecimal> result = failure(ONE);
5 // When
6 Result<?, Boolean> mapped = result.mapFailure(TWO::equals);
7 // Then
8 assertFalse(mapped.getFailure().orElse(null));
9 }
Here, we invoke mapFailure
to transform the failure type of the result from String
to Boolean
for demonstration purposes.
Mapping Both Success and Failure
The map
method simultaneously handles both success and failure cases by applying two separate functions: one for transforming the success value and one for transforming the failure value.
1 @Test
2 void testMap() {
3 // Given
4 Result<String, BigDecimal> result1 = success("HELLO");
5 Result<String, BigDecimal> result2 = failure(ONE);
6 // When
7 Result<Integer, Boolean> mapped1 = result1.map(String::length, TWO::equals);
8 Result<Integer, Boolean> mapped2 = result2.map(String::length, TWO::equals);
9 // Then
10 assertEquals(5, mapped1.orElse(null));
11 assertFalse(mapped2.getFailure().orElse(null));
12 }
Flat-Mapping Results
Flat-mapping is used to chain operations that return results themselves, flattening the nested structures into a single result object. This allows you to transform a success into a failure, or a failure into a success.
To illustrate flat-mapping concepts, the next examples will follow a familiar “pet store” theme. This involves three Java types: Pet
, PetError
, and PetStore
. These types will help us demonstrate the effective use of flat-mapping methods.
1 enum PetError {NOT_FOUND, NO_CONFIG}
2
3 record Pet(long id, String name) {
4
5 static final Pet DEFAULT = new Pet(0, "Default pet");
6 static final Pet ROCKY = new Pet(1, "Rocky");
7 static final Pet GARFIELD = new Pet(2, "Garfield");
8 }
9
10 record PetStore(Pet... pets) {
11
12 PetStore() {
13 this(Pet.ROCKY, Pet.GARFIELD);
14 }
15
16 Result<Pet, PetError> find(long id) {
17 Optional<Pet> found = stream(pets).filter(pet -> pet.id() == id).findAny();
18 return Results.ofOptional(found, NOT_FOUND);
19 }
20
21 Result<Pet, PetError> getDefaultPet(PetError error) {
22 return error == NO_CONFIG ? success(Pet.DEFAULT) : failure(error);
23 }
24
25 Result<Long, PetError> getDefaultPetId(PetError error) {
26 return getDefaultPet(error).mapSuccess(Pet::id);
27 }
28 }
With these types defined, we’ll explore how to use various flat-mapping methods to transform result objects and manage pet-related operations in our imaginary pet store.
Flat-Mapping Successful Results
Use flatMapSuccess
to chain an operation that returns a result object. This method applies a mapping function to the success value, replacing the original result with the new one returned by the function. If the result is a failure, it remains unchanged.
1 @Test
2 void testFlatMapSuccess() {
3 // Given
4 PetStore store = new PetStore();
5 Result<Long, PetError> result = success(100L);
6 // When
7 Result<Pet, PetError> mapped = result.flatMapSuccess(store::find);
8 // Then
9 assertEquals(NOT_FOUND, mapped.getFailure().orElse(null));
10 }
This example starts with a successful result containing a wrong pet ID (not found in the pet store). When we flat-map it with the store’s find
method reference, the final result contains a pet error.
Flat-Mapping Failed Results
Use flatMapFailure
to chain a result-bearing operation. This method also replaces the original result with the new one returned by the mapping function. If the result is a success, it remains unchanged.
1 @Test
2 void testFlatMapFailure() {
3 // Given
4 PetStore store = new PetStore();
5 Result<Long, PetError> result = failure(NO_CONFIG);
6 // When
7 Result<Long, PetError> mapped = result.flatMapFailure(store::getDefaultPetId);
8 // Then
9 assertEquals(Pet.DEFAULT.id(), mapped.orElse(null));
10 }
Here we start with a failed result containing a pet error. When we flat-map it with the store’s getDefaultPetId
method reference, the final result contains the ID of the default pet in the store.
Flat-Mapping Both Success and Failure
The flatMap
method handles both success and failure cases by applying the appropriate function based on the status of the original result.
1 @Test
2 void testFlatMap() {
3 // Given
4 PetStore store = new PetStore();
5 Result<Long, PetError> result1 = success(100L);
6 Result<Long, PetError> result2 = failure(NO_CONFIG);
7 // When
8 Result<Pet, PetError> mapped1 = result1.flatMap(store::find, store::getDefaultPet);
9 Result<Pet, PetError> mapped2 = result2.flatMap(store::find, store::getDefaultPet);
10 // Then
11 assertEquals(NOT_FOUND, mapped1.getFailure().orElse(null));
12 assertEquals(Pet.DEFAULT, mapped2.orElse(null));
13 }
This example starts with a successful result containing a wrong pet ID (not found in the pet store). When we flat-map it with the store’s find
method reference, the final result contains a pet error.
Here we start with a failed result containing a pet error. When we flat-map it with the store’s getDefaultPetId
method reference, the final result contains the ID of the default pet in the store.
Conclusion
We demonstrated how to transform results in a concise and functional manner, enhancing the clarity and flexibility of your error-handling and data-processing logic.
Recap
Level up and lessons learned
Congratulations on reaching the end of this guide! By now, you should have a solid understanding of how to use results in your Java applications effectively. Here’s a brief recap of what you’ve learned:
- Getting Started: You learned how to integrate result objects into your codebase and instantiate new ones.
- Basic Usage: You explored foundational operations like checking statuses, unwrapping values, and executing conditional actions based on result status, enabling you to respond dynamically to success and failure scenarios.
- Advanced Usage: You delved into more sophisticated techniques like screening results to transform successes and failures based on conditions, and leveraging mapping and flat-mapping methods to compose behaviors in a functional style.
For more details on the Result API, you can read the Javadoc reference documentation.
Next, we’ll introduce additional resources where you can further enhance your understanding and skills. Let’s continue expanding your knowledge!
Add-Ons
Boosting results with enhanced capabilities
Add-ons are optional, yet powerful extensions to the Result library, designed to provide extra features that can be integrated on demand.
These small, focused libraries provide a modular approach to extending the core functionalities of Results without adding unnecessary complexity.
- Lazy results allow us to defer expensive calculations until absolutely necessary.
- Fluent assertions for Result allow us to write expressive tests using AssertJ.
- The Jackson datatype module for Result allows us to serialize and deserialize results using Jackson.
- The Micronaut serialization support for Result allows us to serialize and deserialize results using Micronaut.
Lazy Results
How to defer expensive calculations with Results
Lazy results optimize performance by deferring costly operations until absolutely necessary. They behave like regular results, but only execute the underlying operation when an actual check for success or failure is performed.
How to Use this Add-On
Add this Maven dependency to your build:
Group ID | Artifact ID | Version |
---|---|---|
com.leakyabstractions |
result-lazy |
1.0.0.0 |
Maven Central provides snippets for different build tools to declare this dependency.
Creating Lazy Results
We can use LazyResults.ofSupplier
to create a lazy result.
1 Supplier<Result<Integer, String>> supplier = () -> success(123);
2 Result<Integer, String> lazy = LazyResults.ofSupplier(supplier);
While suppliers can return a fixed success or failure, lazy results shine when they encapsulate time-consuming or resource-intensive operations.
1 /* Represents the operation we may omit */
2 Result<Long, Exception> expensiveCalculation(AtomicLong timesExecuted) {
3 long counter = timesExecuted.incrementAndGet();
4 return success(counter);
5 }
This sample method simply increments and returns a counter for brevity. However, in a typical scenario, this would involve an I/O operation.
Skipping Expensive Calculations
The advantage of lazy results is that they defer invoking the provided Supplier
for as long as possible. Despite this, you can screen and transform them like any other result without losing their laziness.
1 @Test
2 void shouldSkipExpensiveCalculation() {
3 AtomicLong timesExecuted = new AtomicLong();
4 // Given
5 Result<Long, Exception> lazy = LazyResults
6 .ofSupplier(() -> expensiveCalculation(timesExecuted));
7 // When
8 Result<String, Exception> transformed = lazy.mapSuccess(Object::toString);
9 // Then
10 assertNotNull(transformed);
11 assertEquals(0L, timesExecuted.get());
12 }
In this example, the expensive calculation is omitted because the lazy result is never fully evaluated. This test demonstrates that a lazy result can be transformed while maintaining laziness, ensuring that the expensive calculation is deferred.
Triggering Result Evaluation
Finally, when it’s time to check whether the operation succeeds or fails, the lazy result will execute it. This is triggered by using any of the terminal methods, such as hasSuccess
.
1 @Test
2 void shouldExecuteExpensiveCalculation() {
3 AtomicLong timesExecuted = new AtomicLong();
4 // Given
5 Result<Long, Exception> lazy = LazyResults
6 .ofSupplier(() -> expensiveCalculation(timesExecuted));
7 // When
8 Result<String, Exception> transformed = lazy.mapSuccess(Object::toString);
9 boolean success = transformed.hasSuccess();
10 // Then
11 assertTrue(success);
12 assertEquals(1L, timesExecuted.get());
13 }
Here, the expensive calculation is executed because the lazy result is finally evaluated.
Handling Success and Failure Eagerly
By default, ifSuccess
, ifFailure
, and ifSuccessOrElse
are treated as terminal methods. This means they eagerly evaluate the result and then perform an action based on its status.
1 @Test
2 void shouldHandleSuccessEagerly() {
3 AtomicLong timesExecuted = new AtomicLong();
4 AtomicLong consumerExecuted = new AtomicLong();
5 Consumer<Long> consumer = x -> consumerExecuted.incrementAndGet();
6 // Given
7 Result<Long, Exception> lazy = LazyResults
8 .ofSupplier(() -> expensiveCalculation(timesExecuted));
9 // When
10 lazy.ifSuccess(consumer);
11 // Then
12 assertEquals(1L, timesExecuted.get());
13 assertEquals(1L, consumerExecuted.get());
14 }
In this test, we don’t explicitly unwrap the value or check the status, but since we want to consume the success value, we need to evaluate the lazy result first.
Furthermore, even if we wanted to handle the failure scenario, we would still need to evaluate the lazy result.
1 @Test
2 void shouldHandleFailureEagerly() {
3 AtomicLong timesExecuted = new AtomicLong();
4 AtomicLong consumerExecuted = new AtomicLong();
5 Consumer<Exception> consumer = x -> consumerExecuted.incrementAndGet();
6 // Given
7 Result<Long, Exception> lazy = LazyResults
8 .ofSupplier(() -> expensiveCalculation(timesExecuted));
9 // When
10 lazy.ifFailure(consumer);
11 // Then
12 assertEquals(1L, timesExecuted.get());
13 assertEquals(0L, consumerExecuted.get());
14 }
In this other test, we use ifFailure
instead of ifSuccess
. Since the lazy result is evaluated to a success, the failure consumer is never executed.
Handling Success and Failure Lazily
When these conditional actions may also be skipped along with the expensive calculation, we can encapsulate them into a LazyConsumer
instead of a regular Consumer
. All we need to do is to create the consumer using LazyConsumer.of
. Lazy consumers will preserve the laziness until a terminal method is eventually used on the result.
1 @Test
2 void shouldHandleSuccessLazily() {
3 AtomicLong timesExecuted = new AtomicLong();
4 AtomicLong consumerExecuted = new AtomicLong();
5 Consumer<Long> consumer = LazyConsumer
6 .of(x -> consumerExecuted.incrementAndGet());
7 // Given
8 Result<Long, Exception> lazy = LazyResults
9 .ofSupplier(() -> expensiveCalculation(timesExecuted));
10 // When
11 lazy.ifSuccess(consumer);
12 // Then
13 assertEquals(0L, timesExecuted.get());
14 assertEquals(0L, consumerExecuted.get());
15 }
Here, we use a lazy consumer with ifSuccess
so the expensive calculation is skipped because the lazy result is never fully evaluated.
Conclusion
We learned how to defer expensive calculations until absolutely necessary. By leveraging lazy results, you can optimize performance by avoiding unnecessary computations and only evaluating the operation’s outcome when needed.
Fluent Assertions
How to assert Result objects fluently
You can use fluent assertions for Result objects to enhance the readability and expressiveness of your unit tests. These assertions are based on AssertJ, an open-source Java library that offers a fluent API for writing assertions in test cases.
![]() |
How to Use this Add-On
Add this Maven dependency to your build:
Group ID | Artifact ID | Version |
---|---|---|
com.leakyabstractions |
result-assertj |
1.0.0.0 |
Maven Central provides snippets for different build tools to declare this dependency.
Asserting Result Objects
You can use ResultAssertions.assertThat
in your tests to create fluent assertions for result objects.
1 import static com.leakyabstractions.result.assertj.ResultAssertions.assertThat;
2
3 @Test
4 void testAssertThat() {
5 // When
6 final Result<Integer, String> result = success(0);
7 // Then
8 assertThat(0).isZero();
9 assertThat(result).hasSuccess(0);
10 }
If, for any reason, you cannot statically import assertThat
, you can use ResultAssert.assertThatResult
instead.
1 import static com.leakyabstractions.result.assertj.ResultAssert.assertThatResult;
2 import static org.assertj.core.api.Assertions.assertThat;
3
4 @Test
5 void testAssertThatResult() {
6 // When
7 final Result<Integer, String> result = success(0);
8 // Then
9 assertThat(0).isZero();
10 assertThatResult(result).hasSuccess(0);
11 }
Conclusion
We covered how to use fluent assertions for Results. This approach allows you to write clear and expressive tests, enhancing the maintainability of your unit tests while ensuring that Result objects behave as expected.
Jackson Module
How to serialize Result objects with Jackson
When using Result objects with Jackson we might run into some problems. The Jackson datatype module for Result solves them by making Jackson treat results as if they were ordinary objects.
![]() |
Jackson is a Java library for JSON parsing and generation. It is widely used for converting Java objects to JSON and vice versa, making it essential for handling data in web services and RESTful APIs. |
How to Use this Add-On
Add this Maven dependency to your build:
Group ID | Artifact ID | Version |
---|---|---|
com.leakyabstractions |
result-jackson |
1.0.0.0 |
Maven Central provides snippets for different build tools to declare this dependency.
Test Scenario
Let’s start by creating a class ApiResponse
containing one ordinary and one Result
field.
1 public class ApiResponse {
2
3 @JsonProperty String version;
4 @JsonProperty Result<String, String> result;
5
6 // Constructors, getters and setters omitted
7 }
Problem Overview
Then we will take a look at what happens when we try to serialize and deserialize ApiResponse
objects.
Serialization Problem
Now, let’s instantiate an ApiResponse
object.
1 ApiResponse response = new ApiResponse();
2 response.setVersion("v1");
3 response.setResult(success("Perfect"));
And finally, let’s try serializing it using an object mapper.
1 ObjectMapper objectMapper = new ObjectMapper();
2 String json = objectMapper.writeValueAsString(response);
We’ll see that now we get an InvalidDefinitionException
.
Java 8 optional type `java.util.Optional<java.lang.String>` not supported by default:
add Module "com.fasterxml.jackson.datatype:jackson-datatype-jdk8" to enable handling
While this may look strange, it’s the expected behavior. When Jackson examined the result object, it invoked getSuccess
and received an optional string value. But Jackson will not handle JDK 8 datatypes like Optional
unless you register the appropriate modules.
1 @Test
2 void testSerializationProblem() {
3 // Given
4 ApiResponse response = new ApiResponse("v1", success("Perfect"));
5 // Then
6 ObjectMapper objectMapper = new ObjectMapper();
7 InvalidDefinitionException error = assertThrows(InvalidDefinitionException.class,
8 () -> objectMapper.writeValueAsString(response));
9 assertTrue(error.getMessage().startsWith(
10 "Java 8 optional type `java.util.Optional<java.lang.String>` not supported"));
11 }
This is Jackson’s default serialization behavior. But we’d like to serialize the result
field like this:
{
"version": "v1",
"result": {
"failure": null,
"success": "Perfect"
}
}
Deserialization Problem
Now, let’s reverse our previous example, this time trying to deserialize a JSON object into an ApiResponse
.
1 String json = "{\"version\":\"v2\",\"result\":{\"success\":\"OK\"}}";
2 ObjectMapper objectMapper = new ObjectMapper();
3 objectMapper.readValue(json, ApiResponse.class);
We’ll see that we get another InvalidDefinitionException
. Let’s inspect the stack trace.
Cannot construct instance of `com.leakyabstractions.result.api.Result`
(no Creators, like default constructor, exist):
abstract types either need to be mapped to concrete types,
have custom deserializer, or contain additional type information
This behavior again makes sense. Essentially, Jackson cannot create new result objects because Result
is an interface, not a concrete type.
1 @Test
2 void testDeserializationProblem() {
3 // Given
4 String json = "{\"version\":\"v2\",\"result\":{\"success\":\"OK\"}}";
5 // Then
6 ObjectMapper objectMapper = new ObjectMapper();
7 InvalidDefinitionException error = assertThrows(InvalidDefinitionException.class,
8 () -> objectMapper.readValue(json, ApiResponse.class));
9 assertTrue(error.getMessage().startsWith(
10 "Cannot construct instance of `com.leakyabstractions.result.api.Result`"));
11 }
Solution Implementation
What we want, is for Jackson to treat Result
values as JSON objects that contain either a success
or a failure
value. Fortunately, there’s a Jackson module that can solve this problem.
Registering the Jackson Datatype Module for Result
Once we have added Result-Jackson as a dependency, all we need to do is register ResultModule
with our object mapper.
1 ObjectMapper objectMapper = new ObjectMapper();
2 objectMapper.registerModule(new ResultModule());
Alternatively, you can also make Jackson auto-discover the module.
1 objectMapper.findAndRegisterModules();
Regardless of the chosen registration mechanism, once the module is registered all functionality is available for all normal Jackson operations.
Serializing Results
Now, let’s try and serialize our ApiResponse
object again:
1 @Test
2 void serializeSuccessfulResult() throws Exception {
3 // Given
4 ApiResponse response = new ApiResponse("v3", success("All good"));
5 // When
6 ObjectMapper objectMapper = new ObjectMapper();
7 objectMapper.registerModule(new ResultModule());
8 String json = objectMapper.writeValueAsString(response);
9 // Then
10 assertTrue(json.contains("v3"));
11 assertTrue(json.contains("All good"));
12 }
If we look at the serialized response, we’ll see that this time the result
field contains a null failure
value and a non-null success
value:
{
"version": "v3",
"result": {
"failure": null,
"success": "All good"
}
}
Next, we can try serializing a failed result.
1 @Test
2 void serializeFailedResult() throws Exception {
3 // Given
4 ApiResponse response = new ApiResponse("v4", failure("Oops"));
5 // When
6 ObjectMapper objectMapper = new ObjectMapper();
7 objectMapper.findAndRegisterModules();
8 String json = objectMapper.writeValueAsString(response);
9 // Then
10 assertTrue(json.contains("v4"));
11 assertTrue(json.contains("Oops"));
12 } // End
We can verify that the serialized response contains a non-null failure
value and a null success
value.
{
"version": "v4",
"result": {
"failure": "Oops",
"success": null
}
}
Deserializing Results
Now, let’s repeat our tests for deserialization. If we read our ApiResponse
again, we’ll see that we no longer get an InvalidDefinitionException
.
1 @Test
2 void deserializeSuccessfulResult() throws Exception {
3 // Given
4 String json = "{\"version\":\"v5\",\"result\":{\"success\":\"Yay\"}}";
5 // When
6 ObjectMapper objectMapper = new ObjectMapper().findAndRegisterModules();
7 ApiResponse response = objectMapper.readValue(json, ApiResponse.class);
8 // Then
9 assertEquals("v5", response.getVersion());
10 assertEquals("Yay", response.getResult().orElse(null));
11 }
Finally, let’s repeat the test again, this time with a failed result. We’ll see that yet again we don’t get an exception, and in fact, have a failed result.
1 @Test
2 void deserializeFailedResult() throws Exception {
3 // Given
4 String json = "{\"version\":\"v6\",\"result\":{\"failure\":\"Nay\"}}";
5 // When
6 ObjectMapper objectMapper = new ObjectMapper().findAndRegisterModules();
7 ApiResponse response = objectMapper.readValue(json, ApiResponse.class);
8 // Then
9 assertEquals("v6", response.getVersion());
10 assertEquals("Nay", response.getResult().getFailure().orElse(null));
11 }
Conclusion
We learned how to serialize and deserialize Result objects using Jackson, demonstrating how the provided datatype module enables Jackson to treat Results as ordinary objects.
Micronaut Serialization
How to serialize Result objects with Micronaut
When using Result objects with Micronaut, we might run into some problems. The Micronaut serialization support for Result solves them by making Micronaut treat results as Serdeable
(so they can be serialized and deserialized).
![]() |
Micronaut is a modern, JVM-based framework for building lightweight microservices and serverless applications. It focuses on fast startup times and low memory usage. Although not as widely adopted as Spring Boot, it has gained popularity for its performance and innovative features. |
How to Use this Add-On
Add this Maven dependency to your build:
Group ID | Artifact ID | Version |
---|---|---|
com.leakyabstractions |
result-micronaut-serde |
1.0.0.0 |
Maven Central provides snippets for different build tools to declare this dependency.
Test Scenario
Let’s start by creating a record ApiOperation
containing one ordinary and one Result field.
1 /** Represents an API operation */
2 @Serdeable
3 public record ApiOperation(String name, Result<String, String> result) {
4 }
Problem Overview
We will take a look at what happens when we try to serialize and deserialize ApiOperation
objects with Micronaut.
Serialization Problem
Now, let’s create a Micronaut controller that returns an instance of ApiOperation
containing a successful result.
1 @Controller("/operations")
2 public class ApiController {
3
4 @Get("/last")
5 ApiOperation lastOperation() {
6 return new ApiOperation("setup", Results.success("Perfect"));
7 }
8 }
And finally, let’s run the application and try the /operations/last
endpoint we just created.
curl 'http://localhost:8080/operations/last'
We’ll see that we get a Micronaut CodecException
caused by a SerdeException
.
No serializable introspection present for type Success.
Consider adding Serdeable. Serializable annotate to type Success.
Alternatively if you are not in control of the project's source code,
you can use @SerdeImport(Success.class) to enable serialization of this type.
Although this may look strange, it’s actually what we should expect. Even though we annotated ApiOperation
as @Serdeable
, Micronaut doesn’t know how to serialize result objects yet, so the data structure cannot be serialized.
1 @Test
2 void testSerializationProblem(ObjectMapper objectMapper) {
3 // Given
4 ApiOperation op = new ApiOperation("setup", success("Perfect"));
5 // Then
6 SerdeException error = assertThrows(SerdeException.class,
7 () -> objectMapper.writeValueAsString(op));
8 assertTrue(error.getMessage().startsWith(
9 "No serializable introspection present for type Success."));
10 }
This is Micronaut’s default serialization behavior. But we’d like to serialize the result
field like this:
{
"name": "setup",
"result": {
"failure": null,
"success": "Perfect"
}
}
Deserialization Problem
Now, let’s reverse our previous example, this time trying to receive an ApiOperation
as the body of a POST
request.
1 @Controller("/operations")
2 public class ApiController {
3
4 @Post("/notify")
5 Map<String, String> notify(@Body ApiOperation op) {
6 return op.result()
7 .mapSuccess(s -> Map.of("message", op.name() + " succeeded: " + s))
8 .orElseMap(f -> Map.of("error", op.name() + " failed: " + f));
9 }
10 }
We’ll see that now we get an IntrospectionException
. Let’s inspect the stack trace.
No bean introspection available for type
[interface com.leakyabstractions.result.api.Result].
Ensure the class is annotated with
io.micronaut.core.annotation.Introspected
This behavior again makes sense. Essentially, Micronaut cannot create new result objects, because Result
is not annotated as @Introspected
or @Serdeable
.
1 @Test
2 void testDeserializationProblem(ObjectMapper objectMapper) {
3 // Given
4 String json = """
5 {"name":"renew","result":{"success":"OK"}}""";
6 // Then
7 IntrospectionException error = assertThrows(IntrospectionException.class,
8 () -> objectMapper.readValue(json, ApiOperation.class));
9 String errorMessage = error.getMessage(); // Extract error message
10 // Verify error message
11 assertTrue(errorMessage.startsWith("No bean introspection available " +
12 "for type [interface com.leakyabstractions.result.api.Result]."));
13 } // End
Solution Implementation
What we want, is for Micronaut to treat Result values as JSON objects that contain either a success
or a failure
value. Fortunately, there’s an easy way to solve this problem.
Adding the Serde Imports to the Classpath
All we need to do now is add Result-Micronaut-Serde as a Maven dependency. Once the @SerdeImport
is in the classpath, all functionality is available for all normal Micronaut operations.
Serializing Results
Now, let’s try and serialize our ApiOperation
object again.
1 @Test
2 void serializeSuccessfulResult(ObjectMapper objectMapper)
3 throws IOException {
4 // Given
5 ApiOperation op = new ApiOperation("clean", success("All good"));
6 // When
7 String json = objectMapper.writeValueAsString(op);
8 // Then
9 assertEquals("""
10 {"name":"clean","result":{"success":"All good"}}""", json);
11 }
If we look at the serialized response, we’ll see that this time the result
field contains a success
field.
{
"name": "clean",
"result": {
"failure": null,
"success": "All good"
}
}
Next, we can try serializing a failed result.
1 @Test
2 void serializeFailedResult(ObjectMapper objectMapper)
3 throws IOException {
4 // Given
5 ApiOperation op = new ApiOperation("build", failure("Oops"));
6 // When
7 String json = objectMapper.writeValueAsString(op);
8 // Then
9 assertEquals("""
10 {"name":"build","result":{"failure":"Oops"}}""", json);
11 }
We can verify that the serialized response contains a non-null failure
value and a null success
value:
{
"name": "build",
"result": {
"failure": "Oops",
"success": null
}
}
Deserializing Results
Now, let’s repeat our tests for deserialization. If we read our ApiOperation
again, we’ll see that we no longer get an IntrospectionException
.
1 @Test
2 void deserializeSuccessfulResult(ObjectMapper objectMapper)
3 throws IOException {
4 // Given
5 String json = """
6 {"name":"check","result":{"success":"Yay"}}""";
7 // When
8 ApiOperation response = objectMapper.readValue(json, ApiOperation.class);
9 // Then
10 assertEquals("check", response.name());
11 assertEquals("Yay", response.result().orElse(null));
12 }
Finally, let’s repeat the test again, this time with a failed result. We’ll see that yet again we don’t get an exception, and in fact, have a failed result.
1 @Test
2 void deserializeFailedResult(ObjectMapper objectMapper)
3 throws IOException {
4 // Given
5 String json = """
6 {"name":"start","result":{"failure":"Nay"}}""";
7 // When
8 ApiOperation response = objectMapper.readValue(json, ApiOperation.class);
9 // Then
10 assertEquals("start", response.name());
11 assertEquals("Nay", response.result().getFailure().orElse(null));
12 }
Conclusion
We learned how to serialize and deserialize Result objects using Micronaut, demonstrating how the provided @SerdeImport
enables Micronaut to treat Results as Serdeable
objects.
Other Resources
Supplementary materials and guidance
Finally, we’ll explore an assortment of resources, designed to provide further context and support for working with the Result library.
Topics include hassle-free dependency management, performance benchmarks, demo projects that demonstrate integration with popular frameworks, and details on the library’s licensing terms.
Bill of Materials
How to declare dependencies without having to worry about version numbers
Tracking multiple add-on versions for your project can quickly become cumbersome. In that situation, you can use the convenient Result Library Bill of Materials to centralize and align their versions. This ensures compatibility and simplifies dependency maintenance.
![]() |
Maven’s Bill of Materials POMs are special POM files that group dependency versions known to be valid and tested to work together, reducing the chances of having version mismatches. |
The basic idea is that instead of specifying a version number for each Result library in your project, you can use this BOM to get a complete set of consistent versions.
How to Use this Add-On
Add this Maven dependency to your build:
Group ID | Artifact ID | Version |
---|---|---|
com.leakyabstractions |
result-bom |
1.0.0.0 |
You can find the latest version of the BOM in Maven Central.
Maven
To import the BOM using Maven, use the following:
<!-- Import the BOM -->
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.leakyabstractions</groupId>
<artifactId>result-bom</artifactId>
<version>1.0.0.0</version>
<scope>import</scope>
<type>pom</type>
</dependency>
</dependencies>
</dependencyManagement>
<!-- Define dependencies without version numbers -->
<dependencies>
<dependency>
<groupId>com.leakyabstractions</groupId>
<artifactId>result</artifactId>
</dependency>
<dependency>
<groupId>com.leakyabstractions</groupId>
<artifactId>result-assertj</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
Gradle
To import the BOM using Gradle, use the following:
dependencies {
// Import the BOM
implementation platform("com.leakyabstractions:result-bom:1.0.0.0")
// Define dependencies without version numbers
implementation("com.leakyabstractions:result")
testImplementation("com.leakyabstractions:result-assertj")
}
Conclusion
We discussed the benefits of using the Bill of Materials for managing dependencies in your project. With the BOM, you can eliminate the hassle of manually specifying version numbers, ensuring consistency and compatibility across all Result libraries.
Benchmarks
Measuring performance to find out how fast Results are
Throughout these guides, we have mentioned that throwing Java exceptions is slow. But… how slow? According to our benchmarks, throwing an exception is several orders of magnitude slower than returning a failed result. This proves that using exceptional logic just to control normal program flow is a bad idea.
We should throw exceptions sparingly, even more so when developing performance-critical applications.
Benchmarking Result Library
This library comes with a set of benchmarks that compare performance when using results versus when using exceptions.
Simple Scenarios
The first scenarios compare the most basic usage: a method that returns a String
or fails, depending on a given int
parameter:
Using Exceptions
1 public String usingExceptions(int number) throws SimpleException {
2 if (number < 0) throw new SimpleException(number);
3 return "ok";
4 }
Using Results
1 public Result<String, SimpleFailure> usingResults(int number) {
2 if (number < 0) return Results.failure(new SimpleFailure(number));
3 return Results.success("ok");
4 }
Complex Scenarios
The next scenarios do something a little bit more elaborate: a method invokes the previous method to retrieve a String
; if successful, then converts it to upper case; otherwise transforms the “simple” error into a “complex” error.
Using Exceptions
1 public String usingExceptions(int number) throws ComplexException {
2 try {
3 return simple.usingExceptions(number).toUpperCase();
4 } catch (SimpleException e) {
5 throw new ComplexException(e);
6 }
7 }
Using Results
1 public Result<String, ComplexFailure> usingResults(int number) {
2 return simple.usingResults(number).map(String::toUpperCase, ComplexFailure::new);
3 }
Conclusion
We provided insights into the Result library’s performance through benchmarking. While our metrics corroborate that most codebases could benefit from using this library instead of throwing exceptions, its main goal is to help promote best practices and implement proper error handling.
![]() |
To address performance concerns, benchmark your applications to gain reusable insights. These should guide your decisions on selecting frameworks and libraries. |
Demo Projects
Check out some REST APIs that consume and produce Result objects
To help you become familiar with this library, you can explore two demo projects that showcase how to handle and serialize Result
objects within popular frameworks like Spring Boot and Micronaut. Each project provides a working example of a “pet store” web service that exposes a REST API for managing pets. They are based on Swagger Petstore Sample and you can interact with them using Swagger-UI.
![]() |
|
These projects illustrate how to develop powerful APIs using Result objects. Follow the examples to create resilient web services that elegantly handle success and failure scenarios.
Spring Boot Demo Project
Take a look at a Spring Boot-based REST API leveraging Result objects
This demo project demonstrates how to handle and serialize Result
objects within a Spring Boot application. It provides a working example of a “pet store” web service that exposes a REST API for managing pets.
Generating the Project
The project was generated via Spring Initializr including features: web and cloud-feign.
Adding Serialization Support
Then Jackson datatype module for Result objects was manually added as a dependency to serialize and deserialize Result
objects.
dependencies {
// ...
implementation platform('com.leakyabstractions:result-bom:1.0.0.0')
implementation 'com.leakyabstractions:result'
implementation 'com.leakyabstractions:result-jackson'
}
We use a @Bean
to register the datatype module.
1 @Configuration
2 public class JacksonConfig {
3 @Bean
4 public Module registerResultModule() {
5 return new ResultModule();
6 }
7 }
API Responses
API responses contain a Result
field, encapsulating the outcome of the requested operation.
1 public class ApiResponse<S> {
2
3 @JsonProperty String version;
4 @JsonProperty Instant generatedOn;
5 @JsonProperty Result<S, ApiError> result;
6 }
Results have different success types, depending on the specific endpoint. Failures will be encapsulated as instances of ApiError
.
Controllers
Controllers return instances of ApiResponse
that will be serialized to JSON by Spring Boot.
1 @RestController
2 public class PetController {
3 // ...
4 @GetMapping("/pet")
5 ApiResponse<Collection<Pet>> list(@RequestHeader("X-Type") RepositoryType type) {
6 log.info("List all pets in {} pet store", type);
7 return response(locate(type)
8 .flatMapSuccess(PetRepository::listPets)
9 .ifSuccess(x -> log.info("Listed {} pet(s) in {}", x.size(), type))
10 .ifFailure(this::logError));
11 }
12 }
Since failures are expressed as ApiError
objects, endpoints invariably return HTTP status 200
.
Running the Application
The application can be built and run with Gradle.
./gradlew bootRun
This will start a stand-alone server on port 8080.
Testing the Server
Once started, you can interact with the API.
curl -s -H 'x-type: local' http://localhost:8080/pet/0
You should see a JSON response like this:
{
"version": "1.0",
"result": {
"success":{
"id": 0,
"name": "Rocky",
"status": "AVAILABLE"
}
}
}
Using Swagger-UI
You can navigate to http://localhost:8080/
to inspect the API using an interactive UI
Micronaut Demo Project
Take a look at a Micronaut-based REST API leveraging Result objects
This demo project demonstrates how to handle and serialize Result
objects within a Micronaut application. It provides a working example of a “pet store” web service that exposes a REST API for managing pets.
Generating the Project
The project was generated via Micronaut Launch including features: annotation-api, http-client, openapi, serialization-jackson, swagger-ui, toml, and validation.
Adding Serialization Support
Then Micronaut Serialization for Result objects was manually added as a dependency to serialize and deserialize Result
objects.
1 dependencies {
2 // ...
3 implementation(platform("com.leakyabstractions:result-bom:1.0.0.0"))
4 implementation("com.leakyabstractions:result")
5 implementation("com.leakyabstractions:result-micronaut-serde")
6 }
That’s all we need to do to make Micronaut treat results as Serdeable
.
API Responses
API responses contain a Result
field, encapsulating the outcome of the requested operation.
1 @Serdeable
2 public class ApiResponse<S> {
3
4 @JsonProperty String version;
5 @JsonProperty Instant generatedOn;
6 @JsonProperty Result<S, ApiError> result;
7 }
Results have different success types, depending on the specific endpoint. Failures will be encapsulated as instances of ApiError
.
Controllers
Controllers return instances of ApiResponse
that will be serialized to JSON by Micronaut:
1 @Controller
2 public class PetController {
3 // ...
4 @Get("/pet")
5 ApiResponse<Collection<Pet>> list(@Header("X-Type") RepositoryType type) {
6 log.info("List all pets in {} pet store", type);
7 return response(locate(type)
8 .flatMapSuccess(PetRepository::listPets)
9 .ifSuccess(x -> log.info("Listed {} pet(s) in {}", x.size(), type))
10 .ifFailure(this::logError));
11 }
12 }
Since failures are expressed as ApiError
objects, endpoints invariably return HTTP status 200
.
Running the Application
The application can be built and run with Gradle.
./gradlew run
This will start a stand-alone server on port 8080.
Testing the Server
Once started, you can interact with the API.
curl -s -H 'x-type: local' http://localhost:8080/pet/0
You should see a JSON response like this:
{
"version": "1.0",
"result": {
"success":{
"id": 0,
"name": "Rocky",
"status": "AVAILABLE"
}
}
}
Using Swagger-UI
You can navigate to http://localhost:8080/
to inspect the API using an interactive UI.
License
Feel free to tweak and share — no strings attached
This library is licensed under the Apache License, Version 2.0 (the “License”); you may not use it except in compliance with the License.
You may obtain a copy of the License at https://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on an “AS IS” BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and limitations under the License.
Permitted
- Commercial Use: You may use this library and derivatives for commercial purposes.
- Modification: You may modify this library.
- Distribution: You may distribute this library.
- Patent Use: This license provides an express grant of patent rights from contributors.
- Private Use: You may use and modify this library without distributing it.
Required
- License and Copyright Notice: If you distribute this library you must include a copy of the license and copyright notice.
- State Changes: If you modify and distribute this library you must document changes made to this library.
Forbidden
- Trademark use: This license does not grant any trademark rights.
- Liability: The library author cannot be held liable for damages.
- Warranty: This library is provided without any warranty.