Table of Contents
JavaScript
The Console’s log
method allows us to print JavaScript data, of any kind, to the Console. Before we dig into all of the cool tricks you can do with the log
method, try the following examples to see what different types of data look like in the Console.
Strings
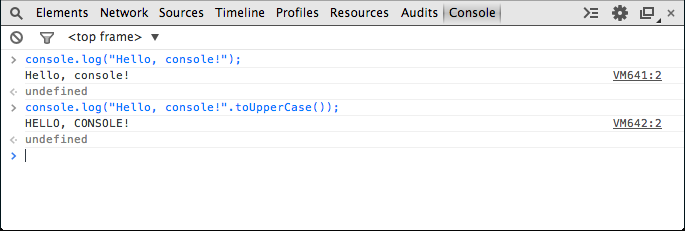
1
console
.
log
(
"Hello, console!"
);
2
// Hello, console!
3
4
console
.
log
(
"Hello, console!"
.
toUpperCase
());
5
// HELLO, CONSOLE!
Numbers
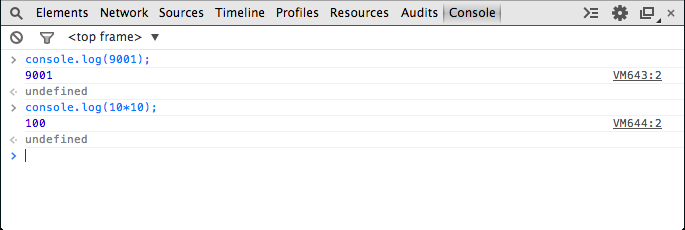
1
console
.
log
(
9001
);
2
// 9001
3
4
console
.
log
(
10
*
10
);
5
// 100
Booleans
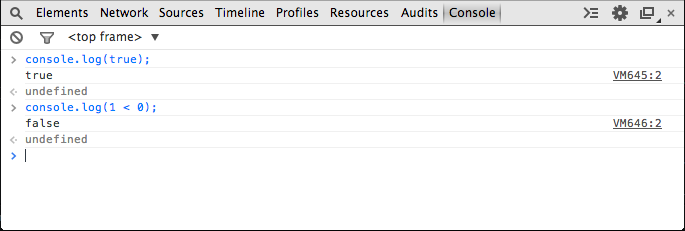
1
console
.
log
(
true
);
2
// true
3
4
console
.
log
(
1
<
0
);
5
// false
Arrays
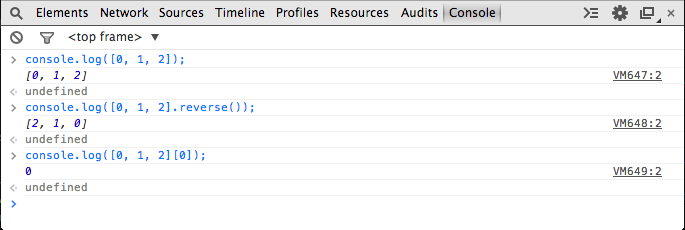
1
console
.
log
([
0
,
1
,
2
]);
2
// [0, 1, 2]
3
4
console
.
log
([
0
,
1
,
2
].
reverse
());
5
// [2, 1, 0]
6
7
console
.
log
([
0
,
1
,
2
][
0
]);
8
// 0
If a logged array contains objects, the child objects will be represented as expandable objects in the console.
For example, this:
1
function
myObject
()
{
2
this
.
foo
=
"bar"
;
3
}
4
5
var
myArray
=
[];
6
7
for
(
var
i
=
0
;
i
<
5
;
i
++
)
{
8
myArray
[
i
]
=
new
myObject
();
9
};
10
11
console
.
log
(
myArray
);
Returns this:
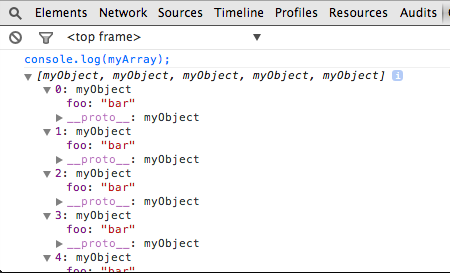
Objects
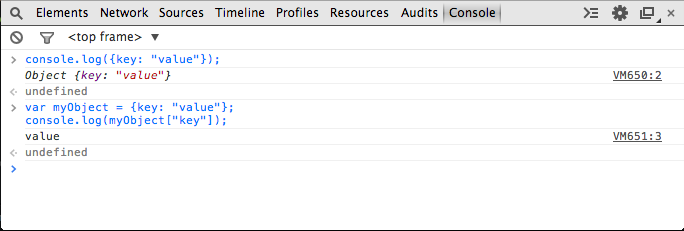
1
console
.
log
({
key
:
"value"
});
2
// Object {key: "value"}
3
4
var
myObject
=
{
key
:
"value"
};
5
console
.
log
(
myObject
[
"key"
]);
6
// value
Functions
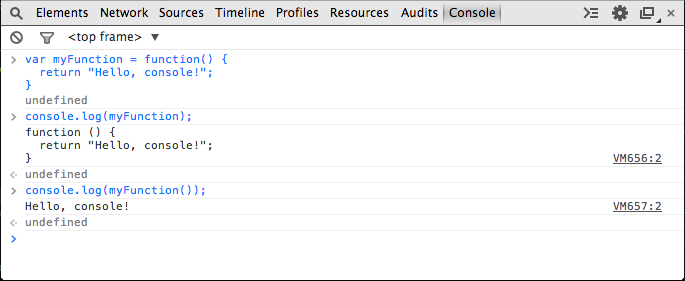
1
var
myFunction
=
function
()
{
2
return
"Hello, console!"
;
3
}
4
5
console
.
log
(
myFunction
);
6
// function () {
7
// return "Hello, console!";
8
// }
9
10
console
.
log
(
myFunction
());
11
// Hello, console!
DOM
Since we’re working in the browser, it’s only sensible that the Console can interact with the DOM. There are two ways to log DOM elements to the Console:
Expandable DOM Trees

1
console
.
log
(
document
);
2
// #document
3
4
console
.
log
(
document
.
getElementById
(
"id"
));
5
// <div id="id">…</div>
6
7
console
.
log
(
document
.
getElementsByTagName
(
"p"
));
8
// [<p>…</p>, <p>…</p>, <p>…</p>]
The examples above will display the selected elements as expandable object, similar to inspecting elements in the Elements panel and mousing over an element in the console will highlight the corresponding element in the DOM.
JavaScript Representations

1
console
.
dir
(
document
.
getElementsByTagName
(
"p"
));
2
// HTMLCollection[3]
3
// 0: p
4
// accessKey: ""
5
// align: ""
6
// attributes: NamedNodeMap
7
// ...
8
// 1: p
9
// 2: p
Using the dir
method instead of log
displays elements as expandable JavaScript objects. This provides tremendous insight into the state of elements in the DOM, and you should really give it a try to see just how much information is logged.
Errors
warn
1
console
.
warn
(
"I'm warning you!"
);
The warn
method displays a yield sign to the left of the text in Chrome and is a purely cosmetic variant of log
.
error
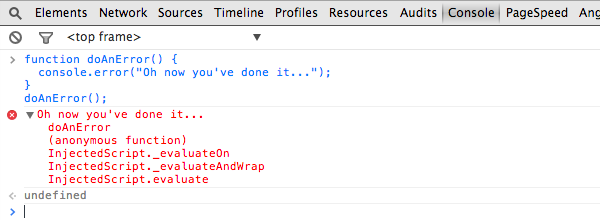
1
function
doAnError
()
{
2
console
.
error
(
"Oh now you've done it..."
);
3
}
4
doAnError
();
The error
method is similar to the log
method, but it includes a stack trace.
Testing
The Console’s assert
method lets you run tests in the browser with a very simple syntax.
1
console
.
assert
(
true
===
false
,
"True is not false!"
);
2
// Assertion failed: True is not false!
The assert
method accepts two arguments: an expression to be evaluated and an object to be logged when the expression is false.
A Somewhat Practical Example
1
var
anchors
=
document
.
getElementsByTagName
(
"a"
);
2
for
(
var
i
=
anchors
.
length
-
1
;
i
>=
0
;
i
--
)
{
3
console
.
assert
(
anchors
[
i
].
title
,
anchors
[
i
],
"is missing a title!"
);
4
};
5
// Assertion failed: <a href="/">Home</a> is missing a title!
In this example, we’re looping over all anchors on a page, checking for titles, and logging the assertion failure along with an expandable DOM object for each each failure.
Tables
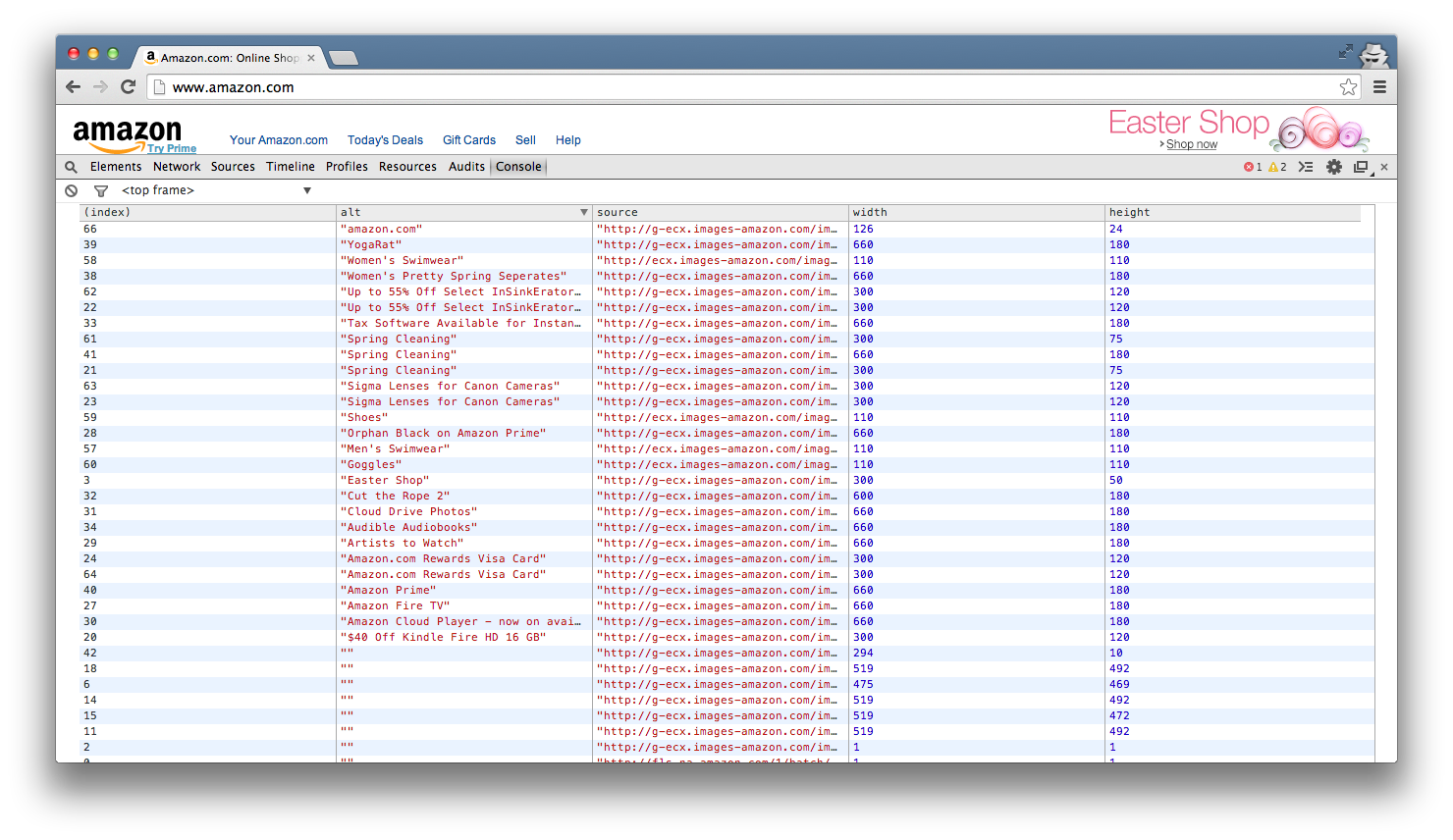
1
function
Image
(
alt
,
source
,
width
,
height
)
{
2
this
.
alt
=
alt
;
3
this
.
source
=
source
;
4
this
.
width
=
width
;
5
this
.
height
=
height
;
6
}
7
8
var
imageElements
=
document
.
getElementsByTagName
(
"img"
);
9
10
var
images
=
{};
11
12
for
(
var
i
=
imageElements
.
length
-
1
;
i
>=
0
;
i
--
)
{
13
var
img
=
imageElements
[
i
];
14
images
[
i
]
=
new
Image
(
img
.
alt
,
img
.
src
,
img
.
width
,
img
.
height
);
15
};
16
17
console
.
table
(
images
,
[
"alt"
,
"source"
,
"width"
,
"height"
]);