Table of Contents
- About
- Introduction to PowerShell for Unix people
- commands summary
- commands detail - a
- commands detail - b
- commands detail - c
- commands detail - d
- commands detail - e
- commands detail - f
- commands detail - g
- commands detail - h
- commands detail - i
- commands detail - j
- commands detail - k
- commands detail - l
- commands detail - m
- commands detail - n
- commands detail - o
- commands detail - p
- commands detail - q
- commands detail - r
- commands detail - s
- commands detail - t
- commands detail - u
- commands detail - v
- commands detail - w
- commands detail - x
- commands detail - y
- commands detail - z
- commands detail - non-alphabetical
- Todo
About
Principal author: Matt Penny
This e-book is intended as a ‘Quick Start’ guide to PowerShell for people who already know Bash or one of the other Unix shells.
The book has 3 elements:
- an introductory chapter which covers some PowerShell concepts
- a summary list of PowerShell equivalents of Unix commands in one e-book chapter
- a detailed discussion of Powershell equivalents of Unix commands, organised in the alphabetical order of the unix command
This guide is released under the Creative Commons Attribution-NoDerivs 3.0 Unported License. The authors encourage you to redistribute this file as widely as possible, but ask that you do not modify the document.
Was this book helpful? The author(s) kindly ask(s) that you make a tax-deductible (in the US; check your laws if you live elsewhere) donation of any amount to The DevOps Collective to support their ongoing work.
Check for Updates! Our ebooks are often updated with new and corrected content. We make them available in three ways:
- Our main, authoritative GitHub organization, with a repo for each book. Visit https://github.com/devops-collective-inc/
- Our GitBook page, where you can browse books online, or download as PDF, EPUB, or MOBI. Using the online reader, you can link to specific chapters. Visit https://www.gitbook.com/@devopscollective
- On LeanPub, where you can download as PDF, EPUB, or MOBI (login required), and “purchase” the books to make a donation to DevOps Collective. You can also choose to be notified of updates. Visit https://leanpub.com/u/devopscollective
GitBook and LeanPub have slightly different PDF formatting output, so you can choose the one you prefer. LeanPub can also notify you when we push updates. Our main GitHub repo is authoritative; repositories on other sites are usually just mirrors used for the publishing process. GitBook will usually contain our latest version, including not-yet-finished bits; LeanPub always contains the most recent “public release” of any book.
Introduction to PowerShell for Unix people
The point of this section is to outline a few areas which I think *nix people should pay particular attention to when learning Powershell.
Resources for learning PowerShell
A full introduction to PowerShell is beyond the scope of this e-book. My recommendations for an end-to-end view of PowerShell are:
- Learn Windows PowerShell in a Month of Lunches - Written by powershell.org’s Don Jones and Jeffery Hicks, I would guess that this is the book that most people have used to learn Powershell.
- Microsoft Virtual Academy’s two PowerShell ‘Jump Start’ videos Getting Started with PowerShell and Advanced Tools & Scripting with PowerShell
unix-like aliases
PowerShell is a friendly environment for Unix people to work in. Many of the concepts are similar, and the PowerShell team have built in a number of Powershell aliases that look like unix commands. So, you can, for example type:
1
ls
….and get this:
1
Directory: C:\temp
2
Mode LastWriteTime Length Name
3
---- ------------- ------ ----
4
-a--- 22/02/2015 16:51 25773 all_the_details.md
5
-a--- 20/02/2015 07:31 3390 commands-summary.md
These can be quite useful when you’re switching between shells, although I found that it can be irritating when the ‘muscle-memory’ kicks in and you find yourself typing ls -ltr
in PowerShell and get an error.
the pipeline
The PowerShell pipeline is much the same as the Bash shell pipeline. The output of one command is piped to another one with the ‘|
’ symbol.
The big difference between piping in the two shells is that in the unix shells you are piping text, whereas in PowerShell you are piping objects.
This sounds like it’s going to be a big deal, but it’s not really.
In practice, if you wanted to get a list of process names, in bash you might do this:
1
ps -ef | cut -c 49-70
…whereas In PowerShell you would do this:
1
get-process | select ProcessName
In Bash you are working with characters, or tab-delimited fields. In PowerShell you work with field names, which are known as ‘properties’.
You can determine the properties of a Powershell object with the command get-member
get-help, get-command, get-member
get-member
When you run a PowerShell command, such as get-history
only a subset of the get-history
output is returned to the screen.
In the case of get-history
, by default two properties are shown - ‘Id’ and ‘Commandline’…
1
$ get-history
2
3
Id CommandLine
4
-- -----------
5
1
dir -recurse c:\t
emp
…but get-history has 4 other properties which you might or might not be interested in:
1
$ get-history |
select
*
2
3
Id : 1
4
CommandLine : dir -recurse c:\t
emp
5
ExecutionStatus : Completed
6
StartExecutionTime : 06
/05/2015 13
:46:56
7
EndExecutionTime : 06
/05/2015 13
:47:07
The disparity between what is shown and what is available is even greater for more complex entities like ‘process’. By default, on my screen, get-process
shows 8 columns, but there are actually over 50 properties (as well as 20 or so methods) available.
The full range of what you can return from a PowerShell command is given by the get-member
command[2].
To run get-member
, you pipe the output of the command you’re interested in to it, for example:
1
get-process | get-member
….or, more typically:
1
get-process | gm
get-member
is one of the ‘trinity’ of ‘help’-ful commands:
- get-member
- get-help
- get-command
get-help
get-help
is similar to the Unix man
[3].
So if you type get-help get-process
, you’ll get this:
1
NAME
2
Get-Process
3
4
SYNOPSIS
5
Gets the processes that are running on the local computer or a remote computer.
6
7
8
SYNTAX
9
Get-Process [[-Name] <String[]>] [-ComputerName <String[]>] [-FileVersionInfo] [\
10
-Module] [<CommonParameters>]
11
12
Get-Process [-ComputerName <String[]>] [-FileVersionInfo] [-Module] -Id <Int32[]\
13
> [<CommonParameters>]
14
15
Get-Process [-ComputerName <String[]>] [-FileVersionInfo] [-Module] -InputObject\
16
<Process[]> [<CommonParameters>]
17
18
19
DESCRIPTION
20
The Get-Process cmdlet gets the processes on a local or remote computer.
21
22
Without parameters, Get-Process gets all of the processes on the local computer.\
23
You can also specify a particular
24
process by process name or process ID (PID) or pass a process object through the\
25
pipeline to Get-Process.
26
27
By default, Get-Process returns a process object that has detailed information a\
28
bout the process and supports
29
methods that let you start and stop the process. You can also use the parameters\
30
of Get-Process to get file
31
version information for the program that runs in the process and to get the modu\
32
les that the process loaded.
33
34
35
RELATED LINKS
36
Online Version: http://go.microsoft.com/fwlink/?LinkID=113324
37
Debug-Process
38
Get-Process
39
Start-Process
40
Stop-Process
41
Wait-Process
42
43
REMARKS
44
To see the examples, type: "get-help Get-Process -examples".
45
For more information, type: "get-help Get-Process -detailed".
46
For technical information, type: "get-help Get-Process -full".
47
For online help, type: "get-help Get-Process -online"
There are a couple of wrinkles which actually make the PowerShell ‘help’ even more help-ful.
- you get basic help by typing
get-help
, more help by typingget-help -full
and…probably the best bit as far as I’m concerned…you can cut to the chase by typingget-help -examples
- there are lots of ‘
about_
’ pages. These cover concepts, new features (in for exampleabout_Windows_Powershell_5.0
) and subjects which dont just relate to one particular command. You can see a full list of the ‘about’ topics by typingget-help about
- get-help works like
man -k
orapropos
. If you’re not sure of the command you want to see help on, just typehelp process
and you’ll see a list of all the help topics that talk about processes. If there was only one it would just show you that topic - Comment-based help. When you write your own commands you can (and should!) use the comment-based help functionality. You follow a loose template for writing a comment header block, and then this becomes part of the get-help subsystem. It’s good.
get-command
If you don’t want to go through the help system, and you’re not sure what command you need, you can use get-command
.
I use this most often with wild-cards either to explore what’s available or to check on spelling.
For example, I tend to need to look up the spelling of ConvertTo-Csv
on a fairly regular basis. PowerShell commands have a very good, very intuitive naming convention of a verb followed by a noun (for example, get-process
, invoke-webrequest
), but I’m never quite sure where ‘to’ and ‘from’ go for the conversion commands.
To quickly look it up I can type:
get-command *csv*
… which returns:
1
$ get-command *csv*
2
3
CommandType Name ModuleName
4
----------- ---- ----------
5
Alias epcsv -> Export-Csv
6
Alias ipcsv -> Import-Csv
7
Cmdlet ConvertFrom-Csv Microsoft.PowerShell.Utility
8
Cmdlet ConvertTo-Csv Microsoft.PowerShell.Utility
9
Cmdlet Export-Csv Microsoft.PowerShell.Utility
10
Cmdlet Import-Csv Microsoft.PowerShell.Utility
11
Application ucsvc.exe
12
Application vmicsvc.exe
Functions
Typically PowerShell coding is done in the form of functions[4]. What you do to code and write a function is this:
Create a function in a plain text .ps1 file[5]
1
gvim say-HelloWorld.ps1
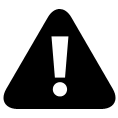
…then source the function when they need it
1
$ . .\s
ay-HelloWorld.ps1
…then run it
1
$ say-helloworld
2
Hello, World
Often people autoload their functions in their $profile
or other startup script, as follows:
1
write-verbose "About to load functions"
2
foreach ($FUNC in $(dir $FUNCTION_DIR\*.ps1))
3
{
4
write-verbose "Loading $FUNC.... "
5
. $FUNC.FullName
6
}
Footnotes
[1] If you wanted the equivalent of ls -ltr
you would use gci | sort lastwritetime
. ‘gci’ is an alias for ‘get-childitem’, ‘sort’ is an alias for ‘sort-object’.
[2] Another way of returning all of the properties of an object is to use ‘select *‘…so in this case you could type get-process | select *
[3] There is actually a built-in alias man
which tranlates to get-help
, so you can just type man
if you’re pining for Unix.
[4] See the following for more detail on writing functions rather than scripts: http://blogs.technet.com/b/heyscriptingguy/archive/2011/06/26/don-t-write-scripts-write-powershell-functions.aspx
[5] I’m using ‘gvim’ here, but notepad would work just as well. PowerShell has a free ‘scripting environment’ called PowerShell ISE, but you don’t have to use it if you dont want to.
commands summary
| Unix | Powershell | | —–|————| | alias (set aliases) | set-alias | | alias (show aliases) | get-alias | | apropos | get-help | basename | dir | select name | cal | See commands detail | | cd | cd | | clear | clear-host | | date | get-date | | date -s | set-date | | df -k | Get-WMIObject Win32_LogicalDisk | ft -a | | diff | Compare-Object -ReferenceObject (Get-Content file1) -DifferenceObject (Get-Content file2) | | dirname | dir | select directory | | du | See commands detail | | echo | write-output | | echo -n | write-host -nonewline | | | egrep -i sql | | where {[Regex]::Ismatch($_.name.tolower(), “sql”) } | | egrep -i | select-string | | egrep | select-string -casesensitive | | egrep -v | select-string -notmatch | | env | Get-ChildItem Env: | fl or get-variable | | errpt | get-eventlog | | export PS1=”$ “ | function prompt {“$ “ } | | find | dir whatever -recurse | | for (start, stop, step) | for ($i = 1; $i -le 5; $i++) {whatever} | | head | gc file.txt | select-object -first 10 | | history | get-history | | history | egrep -i ls | history | select commandline | where commandline -like ‘ls’ | fl | | hostname | hostname | | if-then-else | if ( condition ) { do-this } elseif { do-that } else {do-theother} | | if [ -f “$FileName” ] | if (test-path $FileName) | | kill | stop-process | | less | more| | locate | no equivalent but see link | | ls | get-childitem OR gci OR dir OR ls | | ls -a | ls -force | | lsusb | gwmi Win32_USBControllerDevice | | mailx | send-mailmessage | | man | get-help | | more | more| | mv | rename-item | | pg | more| | ps -ef | get-process | | ps -ef | grep oracle | get-process oracle | | pwd | get-location | | read | read-host | | rm | remove-item | | script | start-transcript | | sleep | start-sleep | | sort | sort-object | | sort -uniq | get-unique | | tail | gc file.txt | select-object -last 10 | | tail -f | gc -tail 10 -wait file.txt | | time | measure-command | | touch - create an empty file | set-content -Path ./file.txt -Value $null | | touch - update the modified date | set-itemproperty -path ./file.txt -name LastWriteTime -value $(get-date) | | wc -l | gc ./file.txt | measure-object | select count | | whoami | [Security.Principal.WindowsIdentity]::GetCurrent() | select name | | whence or type | No direct equivalent, but see link | | unalias | remove-item -path alias:aliasname | | uname -m | Get-WmiObject -Class Win32_ComputerSystem | select manufacturer, model | | uptime | get-wmiobject -class win32_operatingsystem | select LastBootUpTime | | \ (line continuation) | ` (a backtick) |
commands detail - a
alias (list all the aliases)
The Powershell equivalent of typing alias
at the bash prompt is:
1
get-alias
alias (set an alias)
At it’s simplest, the powershell equivalent of the unix ‘alias’ when it’s used to set an alias is ‘set-alias’
1
set-alias ss select-string
However, there’s a slight wrinkle….
In unix, you can do this
1
alias bdump="cd /u01/app/oracle/admin/$ORACLE_SID/bdump/"
If you try doing this in Powershell, it doesn’t work so well. If you do this:
1
set-alias cdtemp "cd c:\temp"
2
cdtemp
…then you get this error:
1
cdtemp
:
The
term
'cd c:\temp'
is
not
recognized
as
the
name
of
a
cmdlet
,
function
,
\
2
script
file
,
or
operable
program
.
Check
the
spelling
of
the
name
,
or
if
a
path
was
i
\
3
ncluded
,
verify
that
the
path
is
correct
and
try
again
.
4
5
At
line
:
1
char
:
1
6
7
+
cdtemp
8
9
+
~~~~~~
10
11
+
CategoryInfo
:
ObjectNotFound
:
(
cd
c
:\
temp
:
String
)
[],
12
13
CommandNotFoundException
14
15
+
FullyQualifiedErrorId
:
CommandNotFoundException
A way around this is to create a function instead:
1
remove
-
item
-
path
alias
:cdtemp
2
3
function
cdtemp
{
cd
c
:
\
temp
}
You could then create an alias for the function:
1
set-alias cdt cdtemp
apropos
apropos
is one of my favourite bash commands, not so much for what it does…but because I like the word ‘apropos’.
I’m not sure it exists on all flavours of *nix, but in bash apropos
returns a list of all the man pages which have something to do with what you’re searching for. If apropos isn’t implemented on your system you can use man -k
instead.
Anyway on bash, if you type:
1
apropos process
…then you get:
1
AF_LOCAL [unix] (7) - Sockets for local interprocess communication
2
3
AF_UNIX [unix] (7) - Sockets for local interprocess communication
4
5
Apache2::Process (3pm) - Perl API for Apache process record
6
7
BSD::Resource (3pm) - BSD process resource limit and priority functions
8
9
CPU_CLR [sched_setaffinity] (2) - set and get a process's CPU affinity mask
10
11
CPU_ISSET [sched_setaffinity] (2) - set and get a process's CPU affinity mask
12
13
CPU_SET [sched_setaffinity] (2) - set and get a process's CPU affinity mask
14
15
CPU_ZERO [sched_setaffinity] (2) - set and get a process's CPU affinity mask
16
17
GConf2 (rpm) - A process-transparent configuration system
The Powershell equivalent of apropos
or man -k
is simply get-help
1
get-help process
2
3
Name Category Module Synopsis
4
5
---- -------- ------ --------
6
7
get-dbprocesses Function Get processes for a particul...
8
9
show-dbprocesses Function Show processes for a particu...
10
11
Debug-Process Cmdlet Microso... Debugs one or more processes...
12
13
Get-Process Cmdlet Microso... Gets the processes that are ...
This is quite a nice feature of PowerShell compared to Bash. If get-help
in Powershell shell scores a ‘direct hit’ (i.e. you type something like get-help debug-process
) it will show you the help for that particular function. If you type something more vague, it will show you a list of all the help pages you might be interested in.
By contrast if you typed man process
at the Bash prompt, you’d just get
1
No manual entry for process
commands detail - b
basename
A rough PowerShell equivalent for the unix basename is:
1
dir <whatever> | select name
This depends on the file actually existing, whereas basename doesn’t care.
A more precise (but perhaps less concise) alternative[1] is:
1
[System.IO.Path]::GetFileName('c:\temp\double_winners.txt')
Notes
[1] I found [System.IO.Path]::GetFileName
after reading Power Tips of the Day - Useful Path Manipulations Shortcuts, which has some other useful commands
commands detail - c
cal
There’s no one-liner equivalent for the Linux cal
, but there’s a useful script, with much of the cal
functionality here :
http://www.vistax64.com/powershell/17834-unix-cal-command.html
cd
The PowerShell equivalent of cd
is:
1
Set-Location
…although there is a builtin PowerShell alias cd
which points at set-location
cd ~
cd ~
moves you to your home folder in both unix and Powershell.
clear
The unix clear
command clears your screen. The Powershell equivalent to the unix clear
is
1
clear-host
PowerShell also has built-in alias clear
for clear-host
.
However, it’s possibly worth noting that the behaviour of the two commands is slightly different between the two environments.
In my Linux environment, running putty, clear
gives you a blank screen by effectively scrolling everything up, which means you can scroll it all back down.
The Powershell Clear-host
on the other hand seems to wipe the previous output (actually in the same way that cmd’s cls
command does….). This could be quite a significant difference, depending on what you want to clear and why!
cp
The Posh version of cp is
1
copy-item
The following are built-in aliases for copy-item:
1
cp
2
3
copy
cp -R
To recursively copy:
1
copy -recurse
commands detail - d
date
The Powershell equivalent of the Unix date
is
1
get-date
The Powershell equivalent of the Unix date -s
is
1
set-date
I was anticipating doing a fairly tedious exercise of going through all the Unix date formats and then working out the Powershell equivalent, but discovered the Powershell Team has effectively done all this for me. There is a Powershell option -UFormat
which stands for ‘unix format’.
So the Powershell:
1
date -Uformat '%D'
2
3
09/08/14
is the same as the *nix
1
date +'%D'
2
3
09/08/14
This is handy…but I have found the odd difference. I tried this for a demo:
Unix:
1
date +'Today is %A the %d of %B, the %V week of the year %Y. My timezone is %Z, and \
2
here it is %R'
3
4
Today is Monday the 08 of September, the 37 week of the year 2014. My timezone is BS\
5
T, and here it is 17:24
Powershell:
1
get-date -Uformat 'Today is %A the %d of %B, the %V week of the year %Y. My timezone\
2
is %Z, and here it is %R'
3
4
Today is Monday the 08 of September, the 36 week of the year 2014. My timezone is +0\
5
1, and here it is 17:25
I presume the discrepancy in the week of the year is to do with when the week turns - as you can see I ran the command on a Monday. Some systems have the turn of the week being Monday, others have it on Sunday.
I don’t know why %Z
outputs different things….and I can’t help feeling I’m being churlish pointing this out. The -UFormat option is a really nice thing to have.
df -k
A quick and dirty Powershell equivalent to ‘df -k’ is
1
Get-WMIObject Win32_LogicalDisk -filter "DriveType=3" | ft
A slightly prettier version is this function:
1
function
get
-
serversize
{
Param
(
[
String
]
$ComputerName
)
2
3
4
Get
-
WMIObject
Win32_LogicalDisk
-
filter
"DriveType=3"
-
computer
$ComputerName
|
5
6
Select
SystemName
,
DeviceID
,
VolumeName
,
7
8
@
{
Name
=
"size (GB)"
;
Expression
=
{
"{0:N1}"
-
f
(
$_
.
size
/
1
gb
)}},
9
10
@
{
Name
=
"freespace (GB)"
;
Expression
=
{
"{0:N1}"
-
f
(
$_
.
freespace
/
1
gb
)}}
11
12
}
13
14
15
function
ss
{
Param
(
[
String
]
$ComputerName
)
16
17
get
-
serversize
$ComputerName
|
ft
18
19
}
….then you can just do:
1
$ ss my_server
….and get
1
SystemName DeviceID VolumeName size(GB) freespace(GB)
2
3
---------- -------- ---------- -------- -------------
4
5
my_server C: OS 30.0 7.8
6
7
my_server D: App 250.0 9.3
8
9
my_server E: 40.0 5.0
dirname
A good PowerShell equivalent to the unix dirname
is
1
gi c:\double_winners\chelsea.doc | select directory
However, this isn’t a direct equivalent. Here, I’m telling Powershell to look at an actual file and then return that file’s directory. The file has to exist. The unix ‘dirname’ doesn’t care whether the file you specify exists or not.
If you type in dirname /tmp/double_winners/chelsea.doc
on any Unix server it will return /tmp/double_winners
, I think. dirname
is essentially a string-manipulation command.
A more precise Powershell equivalent to the unix ‘dirname’ is this
1
[System.IO.Path]::GetDirectoryName('c:\double_winners\chelsea.doc')
….but it’s not as easy to type, and 9 times out of 10 I do want to get the folder for an existing file rather than an imaginary one.
du
While I think there are implementations of du
in PowerShell, personally my recommendation would be to download Mark Russinovich’s ‘du’ tool, which is here:
http://technet.microsoft.com/en-us/sysinternals/bb896651.aspx
This is part of the Microsoft’s ‘sysinternals’ suite.
commands detail - e
echo
echo
is an alias in PowerShell. As you would expect it’s an alias for the closest equivalent to the Linux echo
:
write-output
You use it as follows:
1
write-output "Blue is the colour"
As well as write-output there are a couple of options for use in Powershell scripts and functions:
write-debug
write-verbose
Whether these produce any output is controlled by commandline or environment flags.
echo -n
In bash, echo -n
echoes back the string without printing a newline, so if you do this:
1
$ echo
-n Blue is the colour
you get:
1
Blue is the colour$
….with your cursor ending up on the same line as the output, just after the dollar prompt
Powershell has an exact equivalent of ‘echo -n’. If you type:
1
PS C:\Users\matt> write-host -nonewline "Blue is the colour"
….then you get this:
1
PS C:\Users\matt> write-host -nonewline "Blue is the colour"
2
Blue is the colourPS C:\Users\matt>
Note that -nonewline
doesn’t ‘work’ if you’re in the ISE.
egrep
The best PowerShell equivalent to egrep
or grep
is select-string
:
1
select-string stamford blue_flag.txt
A nice feature of select-string
which isn’t available in grep
is the -context
option. The -context switch allows you to see a specified number of lines either side of the matching one. I think this is similar to SEARCH /WINDOW
option in DCL.
egrep -i
Powershell is case-insensitive by default, so:
1
select-string stamford blue_flag.txt
…would return:
1
blue_flag.txt:3:From Stamford Bridge to Wembley
If you want to do a case sensitive search, then you can use:
1
select-string -casesensitive stamford blue_flag.txt
egrep -v
The Powershell equivalent to the -v
option would be -notmatch
1
select-string -notmatch stamford blue_flag.txt
egrep ‘this|that’
To search for more than one string within a file in bash, you use the syntax:
1
egrep 'blue|stamford' blue_flag.txt
This will return lines which contain either ‘blue’ or ‘stamford’.
The PowerShell equivalent is to seperate the two strings with a comma, so:
1
$ select
-string stamford,blue blue_flag.txt
…returns:
1
blue_flag.txt:2:We'll keep the blue flag flying high
2
blue_flag.txt:3:From Stamford Bridge to Wembley
3
blue_flag.txt:4:We'll keep the blue flag flying high
| egrep -i sql
This is an interesting one, in that it points up a conceptual difference between PowerShell and Bash.
In bash, if you want to pipe into a grep, you would do this:
1
ps -ef | egrep sql
This would show you all the processes which include the string ‘sql’ somewhere in the line returned by ps
. The egrep is searching across the whole line. If the username is ‘mr_sql’ then a line would be returned, and if the process is ‘sqlplus’ than a line would also be returned.
To do something similar in PowerShell you would do something more specific
1
get-process | where processname -like '*sql*'
So the string ‘sql’ has to match the contents of the property processname
. As it happens, get-process by default only returns one text field, so in this case it’s relatively academic, but hopefully it illustrates the point.
env
The Linux ‘env’ shows all the environment variables.
In PowerShell there are two set of environment variables: - windows-level variables and - Powershell-level variable
Windows-level variables are given by:
1
Get-ChildItem Env: | fl
PowerShell-level variables are given by:
1
get-variable
errpt
I think errpt is possibly just an AIX thing (the linux equivalent is, I think, looking at /var/log/message
). It shows system error and log messages.
The PowerShell equivalent would be to look at the Windows eventlog, as follows
1
get-eventlog -computername bigserver -logname application -newest 15
The lognames that I typically look at are ‘system’, ‘application’ or ‘security’.
export PS1=”$ “
In bash the following changes the prompt when you are at the command line
1
export PS1="$ "
The Powershell equivalent to this is:
1
function
prompt
{
2
"$ "
3
}
I found this on Richard Siddaway’s blog: http://msmvps.com/blogs/richardsiddaway/archive/2013/07/21/fun-with-prompts.aspx
commands detail - f
find
The bash find
command has loads of functionality - I could possibly devote many pages to Powershell equivalents of the various options, but at it’s simplest the bash find
does this:
1
find . -name '*BB.txt'
2
3
./Archive/Script_WO7171BB.txt
4
5
./Archive/Script_WO8541BB.txt
6
7
./Archive/Script_WO8645_BB.txt
8
9
./Archive/WO8559B/Script_WO8559_Master_ScriptBB.txt
10
11
./Archive/WO8559B/WO8559_finalBB.txt
12
13
./Archive/WO8559B/WO8559_part1BB.txt
14
15
./Archive/WO8559B/WO8559_part2BB.txt
The simplest Powershell equivalent of the bash find
is simply to stick a -recurse
on the end of a dir
command
1
PS x:\> dir *BB.txt -recurse
2
3
Directory: x:\Archive\WO8559B
4
5
Mode LastWriteTime Length Name
6
---- ------------- ------ ----
7
----- 28/02/2012 17:15 608 Script_WO8559_Master_ScriptBB.txt
8
----- 28/02/2012 17:17 44 WO8559_finalBB.txt
9
----- 28/02/2012 17:17 14567 WO8559_part1BB.txt
10
----- 28/02/2012 17:15 1961 WO8559_part2BB.txt
11
12
Directory: x:\Archive
13
14
Mode LastWriteTime Length Name
15
---- ------------- ------ ----
16
----- 15/06/2011 08:56 2972 Script_WO7171BB.txt
17
----- 14/02/2012 16:39 3662 Script_WO8541BB.txt
18
----- 27/02/2012 15:22 3839 Script_WO8645_BB.txt
If you want Powersehll to give you output that looks more like the Unix find then you can pipe into | select fullname
1
PS x:\> dir *BB.txt -recurse | select fullname
2
3
FullName
4
--------
5
x:\Archive\WO8559B\Script_WO8559_Master_ScriptBB.txt
6
x:\Archive\WO8559B\WO8559_finalBB.txt
7
x:\Archive\WO8559B\WO8559_part1BB.txt
8
x:\Archive\WO8559B\WO8559_part2BB.txt
9
x:\Archive\Script_WO7171BB.txt
10
x:\Archive\Script_WO8541BB.txt
11
x:\Archive\Script_WO8645_BB.txt
for
for loop - start, stop, step
The equivalent of this bash:
1
for (( i = 1 ; i <= 5 ; i++ ))
2
do
3
echo "Hello, world $i"
4
done
5
6
Hello, world 1
7
Hello, world 2
8
Hello, world 3
9
Hello, world 4
10
Hello, world 5
…is
1
for ($i = 1; $i -le 5; $i++)
2
{
3
write-output "Hello, world $i"
4
}
5
6
Hello, world 1
7
Hello, world 2
8
Hello, world 3
9
Hello, world 4
10
Hello, world 5
for loop - foreach item in a list
For the Bash
1
for I in Chelsea Arsenal Spuds
2
do
3
echo $I
4
done
the equivalent Powershell is:
1
foreach ($Team in ("Chelsea", "Arsenal", "Spuds")) {write-output $Team}
for loop - for each word in a string
For the bash:
1
london="Chelsea Arsenal Spurs"
2
for team in $london; do echo "$team"; done
…the equivalent Powershell is:
1
$London = "Chelsea Arsenal Spuds"
2
foreach ($Team in ($London.split())) {write-output $Team}
for loops - for lines in a file
Bash:
1
for team in $(egrep -v mill london.txt)
2
> do
3
> echo $team
4
> done
Posh:
1
select-string -notmatch millwall london.txt | select line | foreach {write-output $_}
or:
1
foreach ($team in (select-string -notmatch millwall london.txt | select line)) {$tea\
2
m}
for loop - for each file in a folder
Bash:
1
for LocalFile in *
2
do
3
echo $LocalFile
4
done
Posh:
1
foreach ($LocalFile in $(gci)) {write-output $LocalFile.Name}
commands detail - g
Not got any commands beginning with ‘g’ yet.
commands detail - h
head
The PowerShell equivalent of the *nix head
is:
1
gc file.txt | select-object -first 10
history
The Powershell equivalent of history
is:
1
get-history
There is a built in alias history
It’s worth noting that history doesn’t persist across PowerShell sessions, although if you search online there are a couple of published techniques for making it persistent.
It’s also perhaps worth noting that Powershell gives you a couple of extra bits of information, if you want them:
1
get-history | gm -MemberType Property
2
3
4
TypeName: Microsoft.PowerShell.Commands.HistoryInfo
5
6
Name MemberType Definition \
7
8
---- ---------- ---------- \
9
10
CommandLine Property string CommandLine {get;} \
11
12
EndExecutionTime Property datetime EndExecutionTime {get;} \
13
14
ExecutionStatus Property System.Management.Automation.Runspaces.PipelineState E\
15
xecutionStatus {get;}
16
Id Property long Id {get;} \
17
18
StartExecutionTime Property datetime StartExecutionTime {get;}
history | egrep -i ls
There is no direct equivalent of the shell functionality you get with set -o vi
sadly. You can up- and down- arrow by default, but if you want to search through your history then you need to do something like this
1
history | select commandline | where-object
{
$_.commandline
-
like
'*ls*'
}
hostname
There is a windows hostname
which does much the same thing as the Unix
hostname
, but it’s not Powershell. It’s a standard-ish Windows executable that on my machine lives in c:\windows\system32
Details are here: https://docs.microsoft.com/en-us/windows-server/administration/windows-commands/hostname
You can get the server name through PowerShell like this:
1
get-wmiobject -class win32_operatingsystem | select __SERVER
commands detail - i
if-then-else
The bash if-then-elif-else
as per:
1
HOUR_OF_DAY=$(date +'%H')
2
3
if [ $HOUR_OF_DAY -lt 6 ]
4
then
5
echo "Still nightime"
6
elif [ $HOUR_OF_DAY -lt 12 ]
7
then
8
echo "Morning has broken"
9
elif [ $HOUR_OF_DAY -lt 18 ]
10
then
11
echo "After noon"
12
else
13
echo "Nightime again"
14
fi
…could be rendered in PowerShell as:
1
[int]$HourOfDay = $(get-date -UFormat '%H')
2
3
if ( $HourOfDay -lt 6 )
4
{
5
write-output "Still nightime"
6
}
7
elseif ( $HourOfDay -lt 12 )
8
{
9
write-output "Morning has broken"
10
}
11
elseif ( $HourOfDay -lt 18 )
12
{
13
write-output "After noon"
14
}
15
else
16
{
17
write-output "Nightime again"
18
}
if [ -f “$FileName” ]
Testing for the existence of a file in bash is done as follows
1
export FileName=~/.matt
2
if [ -f "$FileName" ]
3
then
4
echo "$FileName found."
5
else
6
echo "$FileName not found."
7
fi
In PowerShell this could be[1]
1
$FileName = "c:\powershell\.matt.ps1x"
2
if (test-path $FileName)
3
{echo "$FileName found"}
4
else
5
{echo "$FileName not found"}
Footnotes
[1] The way I’ve rendered the PowerShell here isn’t great, but I’ve left it like that for a couple of reasons. First, it shows the similarity between PowerShell and Bash, which I think is encouraging for anyone reading this e-book. Second it allows me make this brief point about using aliases.
echo
is handy. It’s short, and it looks like it does the same thing as echo
in Unix, MS-DOS and probably a few other languages besides. It pretty much does…but does echo
alias write-output
which allows you to pipe to other PowerShell commands, or does it alias to write-host
, which doesn’t?
I’ve been using PowerShell for a few years now but I didn’t know. I had to look it up. This is extra hassle if you’re reading a script, which is one of the reasons that it’s usually seen as being better practice in scripts to be explicit by using the full command rather than the alias.
Also, in PowerShell scripts rather than this:
1
if (test-path $FileName)
2
{write-host "$FileName found"}
…it would typically be seen as better to format using one of these two alternatives:
1
if (test-path $FileName) {
2
write-host "$FileName found"
3
}
or:
1
if (test-path $FileName)
2
{
3
write-host "$FileName found"
4
}
commands detail - j
None as yet
commands detail - k
kill
The equivalent of bash’s kill
is:
1
stop-process
A typical usage in Powershell might be:
1
# find the process
2
get-process | select id, ProcessName | where
{
$_.processname
-
like
'iex*'
}
3
4
# kill the process
5
stop-process 5240
There is a built in alias kill
which translates to stop-process
1
get-alias k*
2
3
CommandType Name
4
----------- ----
5
Alias kill -> Stop-Process
commands detail - l
locate
There isn’t a builtin PowerShell version of locate, but Chrissy LeMaire’s has written an Invoke-Locate script, ‘in the spirit of (Linux/Unix) GNU findutils’ locate’. It works really well.
https://gallery.technet.microsoft.com/scriptcenter/Invoke-Locate-PowerShell-0aa2673a
ls
The PowerShell equivalent of the Unix ls is:
1
Get-ChildItem
… for which there are aliases dir, ls and gci
ls -a
In linux, ls -a displays hidden files as well as ‘normal’ files.
So ls gives:
1
$ ls
2
README.md
but ls -a gives
1
$ ls -a
2
. .. .function-prompt.ps1.swp .git README.md
The Powershell equivalent of ls -a is get-childitem -force. Here, I’ve used the alias ls
1
$ ls
2
3
4
Directory: C:\U
sers\m
att\D
ocuments\W
indowsPowerShell\f
unctions
5
6
Mode LastWriteTime Length Name
7
---- ------------- ------ ----
8
-a--- 04
/06/2015 13
:20 1422
README.md
9
10
$ ls -force
11
12
Directory: C:\U
sers\m
att\D
ocuments\W
indowsPowerShell\f
unctions
13
14
15
Mode LastWriteTime Length Name
16
---- ------------- ------ ----
17
d--h- 04
/06/2015 13
:20 .git
18
-a-h- 20
/05/2015 17
:33 12288
.function-prompt.ps1.swp
19
-a--- 04
/06/2015 13
:20 1422
README.md
ls -ltr
The Powershell equivalent of the unix ls -ltr (or the DOS dir /OD), which lists files last update order.
1
dir c:\folder | sort-object -property lastwritetime
lsusb
The unix command lsusb shows USB devices. The PowerShell equivalent is:
1
gwmi Win32_USBControllerDevice
gwmi is an alias for get-wmiobject
commands detail - m
mailx
To send an email from the PowerShell command line, this worked for me:
1
$PSEmailServer = "exchange_server.domain.co.uk"
2
send-mailmessage -to eden.hazard@gmail.com -from matt@here.co.uk -subject "Hello"
man
The Powershell equivalent of man
is:
1
get-help
get-help
has the following built-in aliases:
help
man
There are a couple of things to note about get-help
.
There are two much-used options: -full
and -examples
. They both do what you’d expect, I think. To give some idea of scale, on my laptop get-help get-process
currently returns just over a screenful of information, whereas get-help -get-process -full
returns 9 screenfuls.
The help text can be brought up-to-date by running update-help from the command line.
You can easily write your own help text for your own functions, by using a feature called comment-based help.
man -k
In *nix man -k
allows you to search through all the man pages for mentions of a particular keyword. It returns a list of the man pages which are relevant to the word you’ve searched for. On some systems, it’s aliased to apropos
. Anyway, man -k disk
would perhaps return lines for, say, du
, df
and lsvol
(at the time of typing I don’t have a Linux install to hand, so I’m guessing here.)
There’s no seperate command for this in PowerShell, because the get-help
command does this by default if it doesn’t find a direct match.
So, if you type get-help get-process
you would get this:
1
NAME
2
Get-Process
3
4
SYNOPSIS
5
Gets the processes that are running on the local computer or a remote computer.
6
7
8
SYNTAX
9
Get-Process [[-Name] <String[]>] [-ComputerName <String[]>] [-FileVersionInfo] [\
10
-Module] [<CommonParameters>]
11
12
etc....
…whereas if you typed get-help process
you would get a list of help topics related to ‘process’[1]:
1
Name Category Synopsis
2
---- -------- --------
3
Debug-Process Cmdlet Debugs one or more processes running on the local computer.
4
Get-Process Cmdlet Gets the processes that are running on the local computer or \
5
a remote computer.
6
Start-Process Cmdlet Starts one or more processes on the local computer.
7
Stop-Process Cmdlet Stops one or more running processes.
8
Wait-Process Cmdlet Waits for the processes to be stopped before accepting more i\
9
nput.
more
Powershell incorporates a more
command which broadly works in the console similarly to the unix more
.
The Powershell more
is a wrapper for more.com
[2], which is an old Microsoft implementation of more
.
more
doesn’t work in the ISE, but you can however easily scroll back through output by pressing ‘Ctrl’ and ‘Up-arrow’ at the same time. This then allows you to use all the arrow keys (as well as Ctrl-c and Ctrl-V to cut and paste) to navigate around the output from previous commands.
mv
The PowerShell equivalent of mv
is:
1
Rename-Item
Footnotes
[1] I actually did get-help process | select name, category, synopsis | ft -a
to tidy up the output for the e-book.
[2] I found that in my current PowerShell installs, there wasn’t much information on more
. The get-help
command returned the barest of details.
To see what the command actually does I ran:
1
get-command more | select definition | format-list
commands detail - n
Nothing yet
commands detail - o
Nothing for commands beginning with ‘o’ yet.
commands detail - p
ps
The PowerShell equivalent of the ps
command is:
1
get-process
You can use get-process
to get information about other computers:
1
get-process -ComputerName bigserver gvim*
You can use select
and where
to ‘slice and dice’ the information.
1
get-process |
2
where
{
$_.PeakWorkingSet
-
gt
1
Mb
}
| select ProcessName,PeakWorkingSet
As with ps
, the get-process
command has many options. This section of the e-book will be expanded over the next few months but, to start with, these are some of the ps
examples from the Linux man
page.
ps -ef (see every process on the system)
By default get-process
shows all of the processes on the current PC or server
ps (show just current process)
If you wanted to just see details of your process you could do this:
1
get-process -pid $PID
$PID
is an ‘automatic variable’ which contains the PID (process identifier) of the current process
For a list of automatic variables see https://docs.microsoft.com/en-gb/powershell/module/microsoft.powershell.core/about/about_automatic_variables?view=powershell-6&viewFallbackFrom=powershell-Microsoft.PowerShell.Core
ps -ejH (print a process tree)
There is no PowerShell equivalent to the Unix ps -eJH
, because as I understand it Windows processes aren’t part of a process tree.
ps -eLf (get info about threads)
I think this shows information about the processes threads:
1
get-process -pid $pid | select -expand threads
ps -U (show particular user)
1
get-process -IncludeUserName | ? Username -eq "Ronnie\Matt"
ps -ef | grep firefox
1
get-process firefox
pwd
To show your current location in Powershell:
1
Get-Location
…or there are aliases gl
and pwd
.
There is also a built-in variable
1
$pwd
commands detail - q
None as yet
commands detail - r
read -p
In *nix:
1
read -p "Which is the only London club to win the Champions League? " team
2
echo $team
In Powershell:
1
$team = read-host "Which is the only London club to win the Champions League?"
2
Which is the only London club to win the Champions League? : Chelsea
3
4
$team
5
Chelsea
To not echo the input to screen, you would do
1
$SecretString = read-host "Whats your secret? "-assecurestring
This echoes out an asterisk for each character input
rm
1
Remove-Item
commands detail - s
script
1
start-transcript
sleep
1
start-sleep -seconds 5
or
1
start-sleep -milliseconds 250
or just:
1
sleep 3
…will sleep for 3 seconds
sort
1
get-process | sort-object -property VirtualMemorySize
sort -u
The closest PowerShell equivalent to the unix sort -u
is get-unique
1
gc c:\temp\2000.txt | sort | gu
Note: this only works as far I can see if you sort it first
Note 2: get-unique IS case sensitive
sql
This isn’t really a Powershell equivalent of a unix command, but in case it’s useful, to call Sqlserver’s implementation of the sql command line from Powershell you can use invoke-sqlcmd
1
Invoke-Sqlcmd -ServerInstance -query "Select blah" -database _catalog
You need to have the sql module loaded for this to work, or be running the Powershell console from within SSMS
commands detail - t
tail
1
gc file.txt | select-object -last 10
gc
is an alias for get-command
tail -f
1
gc -tail 10 -wait c:\windows\windowsupdate.log
tee
The Powershell equivalent of the unix tee
is tee-object
….which, by default is aliased to tee
So you can do this:
1
get-process | tee c:\temp\test_tee.txt
…to both get a list of processes on your screen and get that output saved into the file in c:\temp
time
The Powershell equivalent of the bash shell ‘time’ is ‘measure-command’.
So, in bash you would do this:
1
time egrep ORA- *log
….and get all the egrep output, then
1
real 0m4.649s
2
user 0m0.030s
3
sys 0m0.112s
In Powershell, you would do this
1
measure-command {select-string ORA- *.sql}
…and get…
1
Days
:
0
2
Hours
:
0
3
Minutes
:
0
4
Seconds
:
0
5
Milliseconds
:
105
6
Ticks
:
1057357
7
TotalDays
:
1.22379282407407
E
-
06
8
TotalHours
:
2.93710277777778
E
-
05
9
TotalMinutes
:
0.00176226166666667
10
TotalSeconds
:
0.1057357
11
TotalMilliseconds
:
105.7357
…you don’t get the ‘user CPU’ time and ‘system CPU’ time, but you do get the added bonus of seeing how long the command took rendered as a fraction of a day!
touch - create an empty file
1
set-content -Path c:\temp\new_empty_file.dat -Value $null
I found the set-content command at <a href=”http://superuser.com/questions/502374/equivalent-of-linux-touch-to-create-an-empty-file-with-powershell”>Super User</a>, the contributor being <a href=”http://superuser.com/users/23133/techie007”>user techie007</a>
touch - update the modified date
1
set-itemproperty -path c:\temp\new_empty_file.dat -name LastWriteTime -value $(get-d\
2
ate)
I got this from a comment by <a href=”https://twitter.com/manung”>Manung Han</a> on the <a href=”http://blog.lab49.com/archives/249#comment-1076”>Lab49 Blog</a>. Doug Finke shares <a href=”http://blog.lab49.com/archives/249”>touch function</a> in a later comment on the same post that fully implements the linux command.
commands detail - u
unalias
1
remove-item -path alias:cdtemp
uname
uname -s
uname -s
in Unix, according to the man page, gives the ‘kernel-version’ of the OS. This is the ‘top-level version’ of the Unix that you’re on. Typical values are ‘Linux’, or ‘AIX’ or ‘HP-UX’. So, on my laptop, typing uname -s
gives:
1
Linux
I’ve only used this when writing a Unix script which have to do slightly different things on different flavours of unix.
Obviously, there’s only one manufacturer for Windows software - Microsoft. So there’s no direct equivalent to uname -s
. The closest equivalent on Powershell would I think be:
get-wmiobject -class win32_operatingsystem | select caption
This returns:
1
caption
2
-------
3
Microsoft Windows 7 Professional
or
1
Microsoft Windows 8.1 Pro
or
1
Microsoft(R) Windows(R) Server 2003, Standard Edition
or
1
Microsoft Windows Server 2008 R2 Enterprise
or
1
Microsoft Windows Server 2012 Standard
uname -n
According to the Linux help, uname -n does this:
1
-n, --nodename
2
print the network node hostname
So, typing uname -n gives
1
$ uname -n
2
nancy.one2one.co.uk
I haven’t found a neat equivalent for this in Powershell, but this works:
1
get-wmiobject -class win32_computersystem | select dnshostname, domain
The output is:
1
dnshostname domain
2
----------- ------
3
nancy one2one.co.uk
uname -r
uname -r gives the kernel release in Unix. The output varies depending on the flavour of Unix - Wikipedia has a good list of examples
On my system uname -r gives:
1
2.6.32-200.20.1.el5uek:
The best Powershell equivalent would seem to be:
1
get-wmiobject -class win32_operatingsystem | select version
…which gives:
1
6.1.7601
The 7601 is Microsoft’s build number.
uname -v
uname -v typically gives the date of the unix build. As far a I can think, there isn’t a Powershell equivalent
uname -m
To be honest, I’m not entirely sure what uname -m shows us on Unix. The wikipedia page for uname shows various outputs none of which are hugely useful.
Running uname -m on my server gives:
1
x86_64
Is this a PowerShell equivalent?
1
$ get-ciminstance -class cim_computersystem |
select
SystemType
2
SystemType
3
----------
4
x64-based PC
uptime
On most, but from memory possibly not all, flavours of *nix ‘uptime’ tells you how long the server has been up and running
1
$ uptime
2
15
:54:24 up 9
days, 5
:43, 2
users, load average: 0
.10, 0
.09, 0
.07
A rough Powershell equivalent to show how long the server (or PC) has been running is:
1
get-wmiobject -class win32_operatingsystem | select LastBootUpTime
….of course you can also do
1
get-wmiobject -class win32_operatingsystem -ComputerName some_other_server |
2
select LastBootUpTime
…to get the bootup time for a remote server, or PC.
commands detail - v
No commands beginning with ‘v’ so far.
commands detail - w
wc -l
1
gc c:\temp\file.txt | measure-object | select count
to show the number of <i>non-blank</i> lines:
1
gc c:\temp\file.txt | measure-object -line
whoami
This shows the user that you are logged on as:
1
[Security.Principal.WindowsIdentity]::GetCurrent() | select name
whence or type
There isn’t a close equivalent to the unix whence
command, because within Powershell there isn’t a PATH variable for scripts. The environment’s PATH and PSMODULEPATH list the folders for windows executables and for Powershell modules.
get-command
shows the location of the windows executable, the name of the Powershell module or the translation of the alias, as follows:
1
get-command whoami,Get-Command,invoke-sqlcmd,sserv,schtasks.exe | select name,versio\
2
n,source,DisplayName
3
4
Name Version Source DisplayName \
5
6
---- ------- ------ ----------- \
7
8
whoami.exe 10.0.17134.1 c:\windows\system32\whoami.exe \
9
10
Get-Command 3.0.0.0 Microsoft.PowerShell.Core \
11
12
Invoke-Sqlcmd 1.0 sqlps \
13
14
sserv 0.0 WindowsStuff sserv -> show-nonstandar\
15
dservices
16
schtasks.exe 10.0.17134.1 c:\windows\system32\schtasks.exe \
17
commands detail - x
None yet
commands detail - y
None yet
commands detail - z
None yet
commands detail - non-alphabetical
To be completed
Todo
While the first version of this e-book is being written this list will be largely mechanical stuff which needs to be done to get the e-book into a suitable state. Subsequently it will be more a list of unix stuff for which I/we stilll need to find or document a PowerShell equivalent.
for future versions
- look at http://blogs.technet.com/b/josebda/archive/2015/04/18/windows-powershell-equivalents-for-common-networking-commands-ipconfig-ping-nslookup.aspx
test conditions (not entirely sure that’s the right unix terminology) - built test conditions like if file exists and is not a directory, if variable exists and is not null
pushd/popd
shutdown -r - restart-computer
more/less - remember it doesn’t work in ISE
find - the various options. -newer, -exec
uname uname options
crontab -l cp -r ls -R .profile
bg cp cut
env eval file find free (memory) fuser filename head
tee
/var/log/message write & (run in background) PS1 (line contunuation prompt) declare -F type Parameter passing cut -f 3 for (p127) while (p139) until case select p113, p136 String comparisons p118 File attribute operations p122 fileinfo Number comparisons p126 IFS (internal field separator) p127 PS3 getopts p145 let p145 arrays p160 here -documents p165 debugging stuff p221 -n (syntax check) -v -x
For the section on ‘ | egrep -i’ i.e. how to search for something within the pipeline, I’ve currently got instructions on how to use -like against a particular property. It would be good to have an alternative which did allow you to search across the whole output. Not very useful typically, but it would be nice to cover it off
export (variables)
my search history function
Mark L’s comments - would expect to see stuff like ‘if-then-else’ in the introductory pages - would be worth looking at the man pages for bash itself (and perhaps for cmd) - cover re-direction - ‘special variables’ - $HOME, $PROFILE
More detail on invoke-locate ?
Cover Get-Item as well as Get-ChildItem for ls
Convert from gwmi to get-ciminstance
More on mailx/send-mailmessage
Think about whether to expand any and all aliases to the full command name
More on mv?
Fill out detail on the ps process tree option. All unix processes being descendants of root, windows processes not necessarily being descendants of anything
More on more :). More isn’t an alias for out-host -paging
ps options - starting with those in the cygwin or bash help
ps -0 (get security info) ps -eo euser,ruser,suser,fuser,f,comm,label ps axZ ps -eM
….have been looking at the cim but not got anything much yet. http://powershell.com/cs/blogs/tips/archive/2009/12/17/get-process-owners.aspx has wmi
ps -o
1
To see every process with a user-defined format:
2
ps -eo pid,tid,class,rtprio,ni,pri,psr,pcpu,stat,wchan:14,comm
3
ps axo stat,euid,ruid,tty,tpgid,sess,pgrp,ppid,pid,pcpu,comm
4
ps -eopid,tt,user,fname,tmout,f,wchan
ps -C
1
Print only the process IDs of syslogd:
2
ps -C syslogd -o pid=
ps -p
1
Print only the name of PID 42:
2
ps -p 42 -o comm=
rm options
rmdir
sort and sort -uniq - more detail on each
uptime - restore the ‘sos’ function etc….but work out what the approved verb would be for ‘show’
who am i - as opposed to whoami. I think it shows the user you originally logged on as
the non-alphabetical stuff: \ and backtick
$ env | sort _=/bin/env CVS_RSH=ssh G_BROKEN_FILENAMES=1 HISTSIZE=1000 HOME=/home/matt HOSTNAME=whatever.co.uk INPUTRC=/etc/inputrc LANG=en_GB LESSOPEN=|/usr/bin/lesspipe.sh %s LOGNAME=matt LS_COLORS=no=00:fi=00:di=00;34:ln=00;36:pi=40;33:so=00;35:bd=40;33;01:cd=40;33;01:or=01;05;37;41:mi=01;05;37;41:ex=00;32:.cmd=00;32:.exe=00;32:.com=00;32:.btm=00;32:.bat=00;32:.sh=00;32:.csh=00;32:.tar=00;31:.tgz=00;31:.arj=00;31:.taz=00;31:.lzh=00;31:.zip=00;31:.z=00;31:.Z=00;31:.gz=00;31:.bz2=00;31:.bz=00;31:.tz=00;31:.rpm=00;31:.cpio=00;31:.jpg=00;35:.gif=00;35:.bmp=00;35:.xbm=00;35:.xpm=00;35:.png=00;35:.tif=00;35: MAIL=/var/spool/mail/matt PATH=/usr/kerberos/bin:/usr/local/bin:/bin:/usr/bin:/home/matt/bin PS1=$ PWD=/home/matt SHELL=/bin/bash SHLVL=1 SSH_TTY=/dev/pts/1 TERM=xterm