End to End Testing in C#: Unit, Integration, and Acceptance Testing
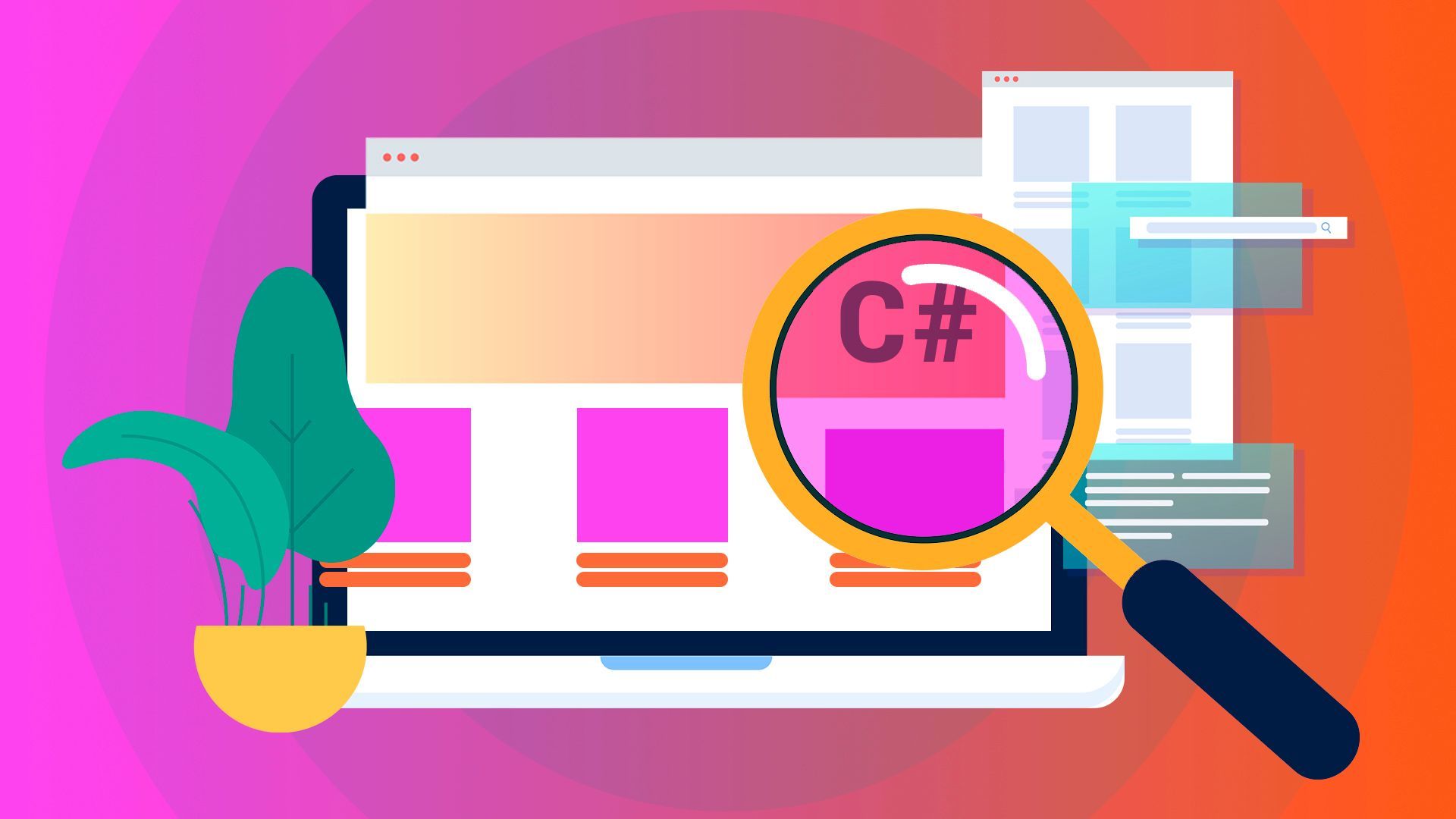
End to End Testing in C#: Unit, Integration, and Acceptance Testing
The following 3 courses are included in this track...
Learn Unit Testing with NUnit and C#, TDD in C# From A to Z, Automate Application with Specflow and Selenium WebDriver C#
EU customers: Price excludes VAT.
VAT is added during checkout.
You can pay in US $ or in your local currency (EUR, GBP, CAD, etc.) when you checkout with a credit card using Stripe.
About the Track
Taking this bundle, you'll learn:
- The basics of writing unit tests
- Best Practices of writing unit tests
- API of NUnit
- Writing test doubles
- Using dependency injection to make code testable
- A lot of TDD practice
- Katas and TDD
- Acceptance and Integration Tests
- Implement a WPF app by TDD
- TDD Best Practices
- SpecFlow for writing acceptance tests
- Selenium: automating a web site
- TestStack.White for testing a WPF app
Take this bundle right now and become a better software engineer!
Verified Certificate
When you successfully complete the Track, you will receive a verified Certificate of Completion from Leanpub, certifying that you have completed the entire Track.
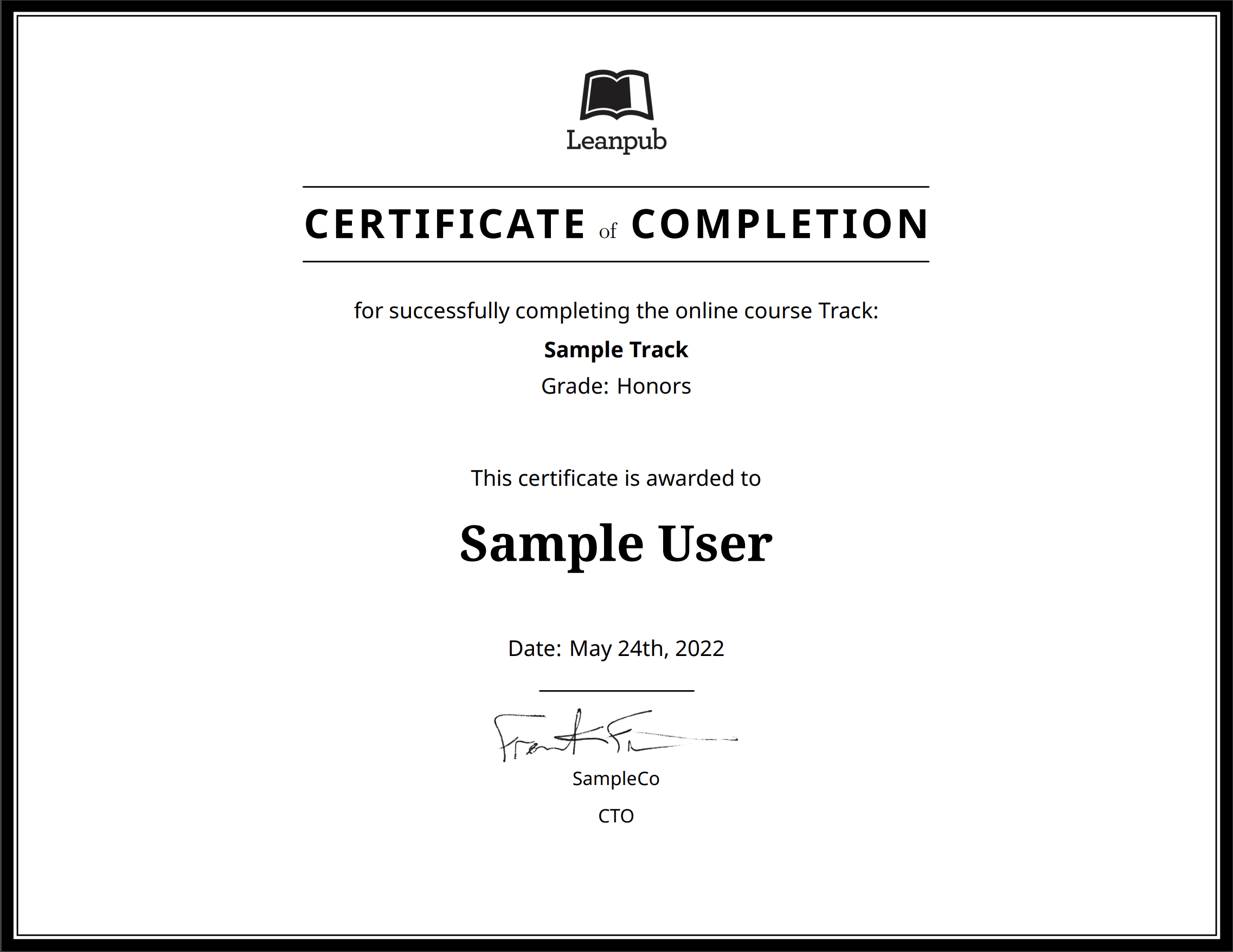
3 Courses Included
- 28
learners
- 0%
complete
english
Learn Unit Testing with NUnit and C#

Teaching Approach
No fluff, no ranting, no beating the air. I esteem your time. The course material is succinct, yet comprehensive. All important concepts are covered. Particularly important topics are covered in-depth. For absolute beginners, I offer my help on Skype absolutely free, if requested.
Take this course, and you will be satisfied.
Build a solid foundation in Unit Testing with this course
This course is all about writing effective unit tests using C# programming language and NUnit as a unit testing framework. Along the way, we will learn the concepts related to unit testing. Today unit testing is an absolutely required skill from any professional developer. Companies expect from developers to know how to write unit tests including all the most important topics such as mocking and test driven development (TDD in short). This course does not cover all the features of NUnit. This course is way more interesting.
Learning unit testing puts a powerful and very useful tool at your fingertips. Being familiar with unit testing you can write reliable and maintainable applications. It is very hard to lead a project which is not covered by unit tests.
Content and Overview
This course is primarily aimed at beginner developers. It provides solid theoretical base reinforced by tons of practical material.
We start with basics of unit testing. What is a unit test? What unit testing frameworks exist? How to run and debug unit tests. After getting acquainted with the basics, we will get to the NUnit framework. Here you’ll learn how to install the framework, set the runner. Then you’ll learn the basics of assertions and arrange-act-assert triplet. Other key features of NUnit are also covered:
- Running tests from the console
- Setup and teardown unit tests
- Parameterized tests
- Grouping and ignoring
Practicing writing of unit tests, it’s impossible to avoid applying mocks. I like the word “test double” more, in general. By the way, you’ll learn what the difference between the following notion is:
- Test double
- Fake
- Dummy
- Stub
- Mock
You’ll learn how to write test doubles manually. You will also see a simple example of how to use a mocking framework for using mocks. I’ll use NSubstitute mocking framework for demonstration.
At the end of this section, you’ll get acquainted with two key approaches to unit testing, Classic or Detroit School and London School of unit testing.
You’ll separately learn the basic of test-driven development. It is hard to imagine a modern professional developer who doesn’t know about TDD, so you’ll learn what it is and what it is about. You’ll see the Red-Green-Refactor triplet in action.
I could not complete the course avoiding the best practices of writing unit tests. You’ll learn the basic concepts of the modern approach to unit testing called “pragmatic unit testing”. You’ll see what problems static classes and singletons bring regarding the unit testing. They make code harder to unit test. After that, you’ll learn the problem of extracting interfaces just for the sake of introducing shims for injecting dependencies.
You’ll know should you write unit tests for the trivial code. You’ll learn a lot more in the course.
So, in short, the course covers the following topics:
- Basic notions of Unit Testing
- NUnit and its basic features
- Test Doubles including fakes, dummies, stubs, spies and mocks
- How to write manual test doubles and how to use a mocking framework
- TDD, red-green-refactor triplet
- A great number of best practices of writing unit tests
In the end, we will recap what you have learned, and you will try to understand where you have to go further with the intention to master your skills.
- 62
learners
- 0%
complete
english
TDD in C# From A to Z

Today unit testing is the absolutely required skill which is required from any professional developer. Companies expect from developers to know how to write unit tests including all the most important topics such as mocking and test driven development (TDD in short).
This course is all about practicing TDD using C# programming language and NUnit as a unit testing framework. Along the way, we will learn the concepts related to unit testing. This course does not cover all the features of NUnit. This course is way more interesting.
Learning unit testing and TDD puts a powerful and very useful tool at your fingertips. Being familiar with unit testing and TDD you can write reliable and maintainable applications. It is very hard to lead a project which is not covered by unit tests.
Content and Overview
This course is primarily aimed at developers who’re already familiar with the basics of unit testing and dependency injection. Some experience in C# programming is required. The course provides solid theoretical base reinforced by tons of practical material.
We start with basics of test-driven development. Why we need TDD? What is TDD? When TDD fails, three laws of TDD, different types of tests, tooling and other fundamental topics. This section is mostly theoretical.
Theory is dead without practice, so starting from the second section, you’ll see tons of programming sessions where I’ll demonstrate how to implement generating of Fibonacci numbers, FizzBuzz, parsing of roman numerals, updateable spin synchronization primitives, tic-tac-toe or crosses and noughts game and game in sticks. You’ll also learn:
- How a regular agile development process looks like
- That you need to learn shortcuts to practice TDD more smoothly
- Three Main TDD techniques: faking, triangulation and obvious implementation
- Which tests to write first
- How to start writing a test in a TDD manner
- Stack kata
- Immutable stack kata
- And list kata
- What is acceptance testing
- About the SpecFlow acceptance testing framework
- How to write acceptance tests with SpecFlow in Gherkin language
- What are UI tests
- What tools for writing UI Tests exist
- How to access UI through the TestStack.White framework
Have you heard about katas? No, I’m talking about programming. In the third section, you’ll learn what is a code kata and I’ll demonstrate three code katas:
Growing an application by writing tests first, we’re not only writing unit tests first. So, in the next section, you’ll learn what is acceptance testing and integration testing. You’ll learn:
You’ll need to see how to apply all the material learned by this moment. Practice helps very much with understanding especially when we uncover highly practical topics such as TDD. That’s why I decided to show you how all the things work in practice altogether. So, in the next section, you’ll see a real enterprise approach for working on a software project in action.
- I’ll build a bridge to UI through TestStack.White applying the Page Object design pattern
- I’ll write acceptance tests using the bridge built for accessing UI
- I’ll implement ViewModels and all the corresponding business-logic
- What is TDD in the end? Is it possible to live without it?
- The relationships between TDD and Agile development process
- Should we design architecture upfront or not?
- Do unit tests guarantee the success?
- Quality of tests, some criterions
- How to express data for writing unit tests
- Shouldly for writing more readable assertions
- Singletons, Static classes and testability, Builder design pattern
- And some other important topics
The last two sections are rather philosophical. We will discuss:
- What is TDD in the end? Is it possible to live without it?
- The relationships between TDD and Agile development process
- Should we design architecture upfront or not?
- Do unit tests guarantee the success?
- Quality of tests, some criterions
- How to express data for writing unit tests
- Shouldly for writing more readable assertions
- Singletons, Static classes and testability, Builder design pattern
- and some other important topics
Here is my Teaching Approach -
No fluff, no ranting, no beating the air. I esteem your time. The course material is succinct, yet comprehensive. All important concepts are covered. Particularly important topics are covered in-depth. For absolute beginners, I offer my help on Skype absolutely free, if requested.
Take this course, and you will be satisfied.
- 25
learners
- 0%
complete
english
Automate Application with Specflow and Selenium WebDriver C#

This course is all about growing object-oriented software guided by tests.
SpecFlow is a framework which brings Behavior-Driven Development (BDD) into life. It is all about filling the gap between technical people and domain experts. SpecFlow is based of a Gherkin language which is very close to natural (though, it is formalized). So, non-technical people can write executable documentation on their own.
Selenium is a framework which allows to drive browsers (Chrome, Firefox, Opera etc.). In other words, with the power of Selenium, you can write a program which automatically interacts with elements on a web page.
TestStack.White (sometimes, written as "teststack white") is a framework which allows to drive WinForms and WPF applications.
This course covers:
- Theoretical background behind different types of testing (unit, integration, and acceptance testing)
- SpecFlow: generating steps, run and debug tests, passing parameters, scenario outline, data table, converting parameters, converting data table, custom conversions, sharing data, categorizing tests, scoped execution, hooks and other features
- Selenium WebDriver: Locators, XPath Locators, CSS Locators, Interacting with all the types of Elements, Timeouts: Explicit and Implicit, Locators Priority, Picking Scenarios to Test, "Page Object" design pattern, Page Object Factory, uploading files
- Scraping a Live Web Site with Selenium WebDriver
- Selenium Extras: managing a web browser
- TestStack.White and Building a WPF App by TDD (with MVVM)
- Appendixes: intro to Unit Testing and Test-Driven Development
Instructors
I'm thankful enough for that I love what I do.
I began my career as a postgraduate student participating in Microsoft ImagineCup contest.
I've been working with .NET platform since 2003. I've been professionally architecting and implementing software for more than 10 years, primarily based on the .NET platform. I'm passionate about building rich and powerful applications using modern technologies. I'm a certified specialist in Windows Applications and Service Communication Applications by Microsoft. I'm one of the coordinators of the MskDotNet User Group in Moscow.
"If it's work, we try to do less. If it's art, we try to do more." - Seth Godin.
What I can say is that software is my art.
The Leanpub 60 Day 100% Happiness Guarantee
Within 60 days of purchase you can get a 100% refund on any Leanpub purchase, in two clicks.
Now, this is technically risky for us, since you'll have the book or course files either way. But we're so confident in our products and services, and in our authors and readers, that we're happy to offer a full money back guarantee for everything we sell.
You can only find out how good something is by trying it, and because of our 100% money back guarantee there's literally no risk to do so!
So, there's no reason not to click the Add to Cart button, is there?
See full terms...
Earn $8 on a $10 Purchase, and $16 on a $20 Purchase
We pay 80% royalties on purchases of $7.99 or more, and 80% royalties minus a 50 cent flat fee on purchases between $0.99 and $7.98. You earn $8 on a $10 sale, and $16 on a $20 sale. So, if we sell 5000 non-refunded copies of your book for $20, you'll earn $80,000.
(Yes, some authors have already earned much more than that on Leanpub.)
In fact, authors have earnedover $14 millionwriting, publishing and selling on Leanpub.
Learn more about writing on Leanpub
Free Updates. DRM Free.
If you buy a Leanpub book, you get free updates for as long as the author updates the book! Many authors use Leanpub to publish their books in-progress, while they are writing them. All readers get free updates, regardless of when they bought the book or how much they paid (including free).
Most Leanpub books are available in PDF (for computers) and EPUB (for phones, tablets and Kindle). The formats that a book includes are shown at the top right corner of this page.
Finally, Leanpub books don't have any DRM copy-protection nonsense, so you can easily read them on any supported device.
Learn more about Leanpub's ebook formats and where to read them